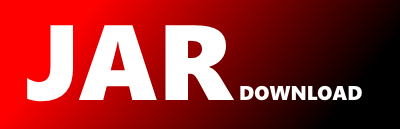
com.thesett.junit.extensions.AsymptoticTestCase Maven / Gradle / Ivy
Show all versions of junit-toolkit Show documentation
/*
* Copyright The Sett Ltd, 2005 to 2014.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.thesett.junit.extensions;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import junit.framework.TestCase;
/**
* AsymptoticTestCase is an extension of TestCase for writing unit tests to analyze asymptotic time and space behaviour.
*
* ParameterizedTestCases allow tests to be defined which have test methods that take a single int argument. Normal
* JUnit test methods do not take any arguments. This int argument can be interpreted in any way by the test but it is
* intended to denote the 'size' of the test to be run. For example, when testing the performance of a data structure
* for different numbers of data elements held in the data structure the int parameter should be interpreted as the
* number of elements. Test timings for different numbers of elements can then be captured and the asymptotic behaviour
* of the data structure with respect to time analyzed. Any non-parameterized tests defined in extensions of this class
* will also be run.
*
*
TestCases derived from this class may also define tear down methods to clean up their memory usage. This is
* intended to be used in conjunction with memory listeners that report the amount of memory a test uses. The idea is to
* write a test that allocates memory in the main test method in such a way that it leaves that memory still allocated
* at the end of the test. The amount of memory used can then be measured before calling the tear down method to clean
* it up. In the data structure example above, a test will allocate as many elements as are requested by the int
* parameter and deallocate them in the tear down method. In this way memory readings for different numbers of elements
* can be captured and the asymptotic behaviour of the data structure with respect to space analyzed.
*
*
CRC Card
* Responsibilities Collaborations
* Store the current int parameter value. {@link TKTestResult} and see {@link AsymptoticTestDecorator} too.
* Invoke parameterized test methods.
*
*
* @author Rupert Smith
* @todo If possible try to move the code that invokes the test and setup/teardown methods into {@link TKTestResult}
* or {@link AsymptoticTestDecorator} rather than this class. This would mean that tests don't have to extend
* this class to do time and space performance analysis, these methods could be added to any JUnit TestCase
* class instead. This would be an improvement because existing unit tests wouldn't have to extend a different
* class to work with this extension, and also tests that extend other junit extension classes could have
* parameterized and tear down methods too.
*/
public class AsymptoticTestCase extends TestCase implements InstrumentedTest
{
/** Used for logging. */
/*private static final Logger log = Logger.getLogger(AsymptoticTestCase.class);*/
/** The name of the test case. */
private final String testCaseName;
/** Thread local for holding measurements on a per thread basis. */
final ThreadLocal