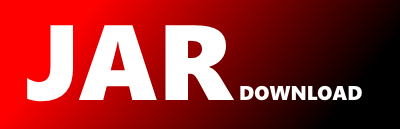
com.thesett.aima.search.impl.MaxStepsAlgorithm Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of search Show documentation
Show all versions of search Show documentation
Search code developed from 'Artificial Intelligence a Modern Approach', Prentice Hall.
/*
* Copyright The Sett Ltd, 2005 to 2014.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.thesett.aima.search.impl;
import java.util.Collection;
import java.util.Queue;
import com.thesett.aima.search.SearchNode;
import com.thesett.aima.search.SearchNotExhaustiveException;
import com.thesett.aima.search.Traversable;
import com.thesett.aima.search.spi.QueueSearchState;
import com.thesett.common.util.logic.UnaryPredicate;
/**
* Implements the simplest queue based search algorithm that checks the next element on the queue until the search space
* is exhausted, or a maximum number of steps is reached.
*
* By default the next element is removed from the queue and has its successors expanded on the queue (provided they
* have not already been) and is then goal checked. The peek-at-head flag may be set to alter this behaviour (through
* the {@link #setPeekAtHead} method), so that the next element on the queue has its successors expanded onto the queue
* without being removed itself. The head element of the queue is then removed and goal checked. This head element may
* be different to the next element that had its successors expanded where an ordered queue is used and the ordering is
* such that some of the successors were placed before their parent node. This is usefull for producing searches that
* examine child nodes before parent nodes, for example a post-fix search.
*
* There is a reverse successors option that may be set (through the {@link #setReverseEnqueueOrder} method), which
* causes the successors to be expanded onto the queue in reverse order to which they are presented. This is usefull for
* stack based searches, such as depth first search, which by the nature of the stack reverse the order of elements.
* Setting this flag will do a double reverse of the element order and restore an intuituve left-to-right search order
* over elements. Generally speaking, set this flag whenever using a LIFO based queue.
*
* A maximum number of node expansions may be set. If the search does not find a goal within this maximum number
* then a {@link SearchNotExhaustiveException} is raised.
*
*
CRC Card
* Responsibilities Collaborations
* Search based on the queue ordering until a goal node is found or the maximum allowed number of steps is
* reached.
*
*
* @author Rupert Smith
*/
public class MaxStepsAlgorithm© 2015 - 2025 Weber Informatics LLC | Privacy Policy