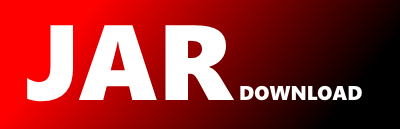
com.thesett.aima.search.util.TreeSearchState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of search Show documentation
Show all versions of search Show documentation
Search code developed from 'Artificial Intelligence a Modern Approach', Prentice Hall.
The newest version!
/*
* Copyright The Sett Ltd, 2005 to 2014.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.thesett.aima.search.util;
import java.util.ArrayList;
import java.util.Iterator;
import com.thesett.aima.search.Operator;
import com.thesett.aima.search.TraversableState;
import com.thesett.common.util.Tree;
/**
* TreeSearchState is a {@link TraversableState} that enables {@link com.thesett.aima.search.QueueBasedSearchMethod}s to
* be applied to {@link com.thesett.common.util.Tree} s. It does not dictate the method of searching, or the order in
* which the nodes and leafs of the tree are visited; a free choice of search algorithm is permitted. The constructor
* accepts a predicate over the tree element type that is used to determine whether node or leaf points in the tree
* correspond to goal states of the search.
*
*
CRC Card
* Responsibilities Collaborations
* Translate operations into new states.
* Report the cost of an operation.
* Enumerate the valid operations on a state.
* Provide links to successor states.
* Report goal state status.
*
*
* @author Rupert Smith
*/
public class TreeSearchState© 2015 - 2025 Weber Informatics LLC | Privacy Policy