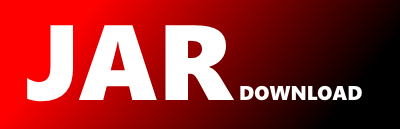
com.thesett.aima.logic.fol.wam.optimizer.Matcher Maven / Gradle / Ivy
/*
* Copyright The Sett Ltd, 2005 to 2014.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.thesett.aima.logic.fol.wam.optimizer;
import java.util.Iterator;
import java.util.Queue;
import com.thesett.common.util.SequenceIterator;
/**
* Matcher is a sequence that is used to drive a {@link StateMachine}. An input sequence is fed into an FSMD and the
* output of the state machine is presented as another sequence.
*
*
CRC Card
* Responsibilities Collaborations
* Feed an input sequence into a state machine.
* Extract the output of a state machine as a sequence.
* Inform the state machine when the end of the input sequence is reached.
* Sink output from the state machine.
* Accept flush commands from the state machine, indicating that a sequence of outputs is complete.
*
*
* @param © 2015 - 2025 Weber Informatics LLC | Privacy Policy