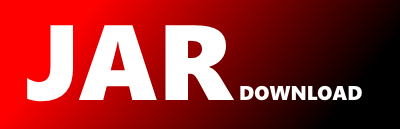
com.thomasjensen.checkstyle.addons.checks.misc.DecomposedPath Maven / Gradle / Ivy
The newest version!
package com.thomasjensen.checkstyle.addons.checks.misc;
/*
* Checkstyle-Addons - Additional Checkstyle checks
* Copyright (c) 2015-2022, the Checkstyle Addons contributors
*
* This program is free software: you can redistribute it and/or modify it under the
* terms of the GNU General Public License, version 3, as published by the Free
* Software Foundation.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A
* PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with this
* program. If not, see .
*/
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import javax.annotation.Nonnull;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import net.jcip.annotations.Immutable;
/**
* Encapsulates the components of a checked file path.
*/
@Immutable
public class DecomposedPath
{
private final String modulePath;
private final String mdlPath;
private final String specificPath;
private final String simpleFilename;
private final Set fileExtensions;
private final List specificFolders;
/**
* Constructor.
*
* @param pModulePath the module path, for example subsystem1/module1
* @param pMdlPath the MDL path, for example src/main/java
* @param pSpecificPath the specific path, for example com/acme/Foo.java
* @param pSimpleFilename the simple file name, for example Foo.java
* @param pFileExtensions the list of file extensions, for example java
, but it could be a list if
* multiple dots are present (e.g. tar.gz, gz
)
* @param pSpecificFolders the simple names of all folders on the specific path, for example com,
* acme
*/
public DecomposedPath(@Nonnull final String pModulePath, @Nonnull final String pMdlPath,
@Nonnull final String pSpecificPath, @Nonnull final String pSimpleFilename,
@Nonnull final Set pFileExtensions, @Nonnull final List pSpecificFolders)
{
modulePath = pModulePath;
mdlPath = pMdlPath;
specificPath = pSpecificPath;
simpleFilename = pSimpleFilename;
fileExtensions = Collections.unmodifiableSet(new HashSet<>(pFileExtensions));
specificFolders = Collections.unmodifiableList(new ArrayList<>(pSpecificFolders));
}
@Nonnull
public String getModulePath()
{
return modulePath;
}
@Nonnull
public String getMdlPath()
{
return mdlPath;
}
@Nonnull
public String getSpecificPath()
{
return specificPath;
}
@Nonnull
public String getSimpleFilename()
{
return simpleFilename;
}
@Nonnull
@SuppressFBWarnings(value = "EI_EXPOSE_REP", justification = "It's really a Collections.unmodifiableSet().")
public Set getFileExtensions()
{
return fileExtensions;
}
@Nonnull
@SuppressFBWarnings(value = "EI_EXPOSE_REP", justification = "It's really a Collections.unmodifiableList().")
public List getSpecificFolders()
{
return specificFolders;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy