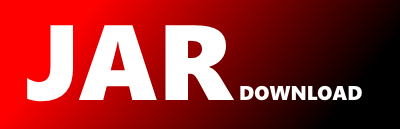
com.thorstenmarx.webtools.test.MockCacheLayer Maven / Gradle / Ivy
package com.thorstenmarx.webtools.test;
/*-
* #%L
* webtools-manager
* %%
* Copyright (C) 2016 - 2019 Thorsten Marx
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
import com.github.benmanes.caffeine.cache.Expiry;
import com.thorstenmarx.webtools.api.cache.CacheLayer;
import com.thorstenmarx.webtools.api.cache.Expirable;
import java.io.Serializable;
import java.util.Optional;
import java.util.concurrent.TimeUnit;
/**
*
* @author marx
*/
public class MockCacheLayer implements CacheLayer {
private Cache cache;
public MockCacheLayer() {
cache = Caffeine.newBuilder().expireAfter(new Expiry() {
@Override
public long expireAfterCreate(String key, Expirable value, long currentTime) {
return value.getCacheTime();
}
@Override
public long expireAfterUpdate(String key, Expirable value, long currentTime, long currentDuration) {
return currentDuration;
}
@Override
public long expireAfterRead(String key, Expirable value, long currentTime, long currentDuration) {
return currentDuration;
}
}).build();
}
@Override
public void add(final String key, final T value, final long duration, final TimeUnit unit) {
Expirable cache_value = new Expirable(value, unit.toNanos(duration));
cache.put(key, cache_value);
}
@Override
public Optional get(final String key, final Class clazz) {
Expirable ifPresent = cache.getIfPresent(key);
if (ifPresent != null && clazz.isInstance(ifPresent.getValue())) {
return Optional.ofNullable(clazz.cast(ifPresent.getValue()));
}
return Optional.empty();
}
@Override
public boolean exists(final String key) {
return cache.getIfPresent(key) != null;
}
@Override
public void invalidate(final String key) {
cache.invalidate(key);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy