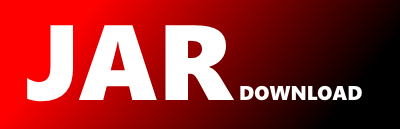
com.thorstenmarx.webtools.test.MockEntities Maven / Gradle / Ivy
/*
* Copyright (C) 2019 Thorsten Marx
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package com.thorstenmarx.webtools.test;
/*-
* #%L
* webtools-actions
* %%
* Copyright (C) 2016 - 2019 Thorsten Marx
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import com.thorstenmarx.webtools.api.actions.model.AdvancedSegment;
import com.thorstenmarx.webtools.api.entities.Entities;
import com.thorstenmarx.webtools.api.entities.Result;
import com.thorstenmarx.webtools.api.entities.Serializer;
import com.thorstenmarx.webtools.api.entities.Store;
import com.thorstenmarx.webtools.api.entities.criteria.Criteria;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
import java.util.Map;
import java.util.UUID;
/**
*
* @author marx
*/
public class MockEntities implements Entities {
@Override
public Store store(Class clazz) {
return new MockStore<>();
}
@Override
public Store store(Class clazz, Serializer serializer) {
return new MockStore<>();
}
public static class MockStore implements Store {
public Map entities = new HashMap<>();
@Override
public Criteria criteria() {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public void delete(T entity) {
entities.remove(entity);
}
@Override
public T get(String id) {
return entities.get(id);
}
@Override
public Result list(final int offset, final int limit) {
return new MockResult<>(List.copyOf(entities.values()), offset, limit);
}
@Override
public String save(T entity) {
String uid = UUID.randomUUID().toString();
((AdvancedSegment)entity).setId(uid);
entities.put(uid, entity);
return uid;
}
@Override
public List save(List entities) {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
@Override
public void clear() {
entities.clear();
}
@Override
public int size() {
return entities.size();
}
}
public static class MockResult extends ArrayList implements Result {
private final int offset;
private final int limit;
protected MockResult (final List result, final int offset, final int limit) {
super();
addAll(result);
this.offset = offset;
this.limit = limit;
}
@Override
public int totalSize() {
return size();
}
@Override
public int offset() {
return offset;
}
@Override
public int limit() {
return limit;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy