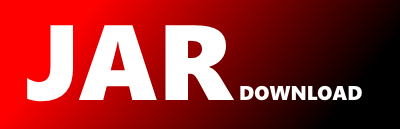
com.thoughtworks.gauge.datastore.ScenarioDataStore Maven / Gradle / Ivy
/*----------------------------------------------------------------
* Copyright (c) ThoughtWorks, Inc.
* Licensed under the Apache License, Version 2.0
* See LICENSE.txt in the project root for license information.
*----------------------------------------------------------------*/
package com.thoughtworks.gauge.datastore;
import java.util.Collections;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
public class ScenarioDataStore {
private static final InheritableThreadLocal> MAP = new InheritableThreadLocal>() {
@Override
protected ConcurrentHashMap
© 2015 - 2024 Weber Informatics LLC | Privacy Policy