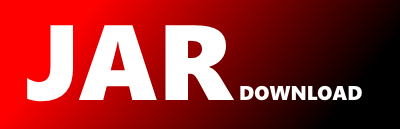
com.threecrickets.jvm.json.generic.MapEncoder Maven / Gradle / Ivy
Show all versions of json-jvm Show documentation
/**
* Copyright 2010-2016 Three Crickets LLC.
*
* The contents of this file are subject to the terms of the Mozilla Public
* License version 1.1: http://www.mozilla.org/MPL/MPL-1.1.html
*
* Alternatively, you can obtain a royalty free commercial license with less
* limitations, transferable or non-transferable, directly from Three Crickets
* at http://threecrickets.com/
*/
package com.threecrickets.jvm.json.generic;
import java.io.IOException;
import java.util.Iterator;
import java.util.Map;
import com.threecrickets.jvm.json.JsonContext;
import com.threecrickets.jvm.json.JsonEncoder;
/**
* A JSON encoder for {@link Map} implementations.
*
* @author Tal Liron
*/
public class MapEncoder implements JsonEncoder
{
//
// JsonEncoder
//
public boolean canEncode( Object object, JsonContext context )
{
return object instanceof Map;
}
public void encode( Object object, JsonContext context ) throws IOException
{
@SuppressWarnings("unchecked")
Map map = (Map) object;
context.out.append( '{' );
if( !map.isEmpty() )
{
context.newline();
for( Iterator> i = map.entrySet().iterator(); i.hasNext(); )
{
Map.Entry entry = i.next();
context.indentNested();
context.quoted( entry.getKey() );
context.colon();
context.nest().encode( entry.getValue() );
if( i.hasNext() )
context.comma();
}
context.newline();
context.indent();
}
context.out.append( '}' );
}
}