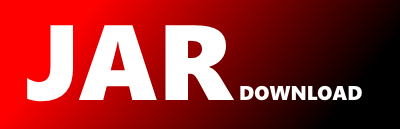
com.threerings.nexus.distrib.DValue Maven / Gradle / Ivy
The newest version!
//
// Nexus Core - a framework for developing distributed applications
// http://github.com/threerings/nexus/blob/master/LICENSE
package com.threerings.nexus.distrib;
import com.threerings.nexus.io.Streamable;
import static com.threerings.nexus.util.Log.log;
/**
* A value attribute for a Nexus object. Contains a single value, which may be updated.
*/
public class DValue extends react.Value implements DAttribute
{
/**
* Creates a new attribute with the specified owner and initial value.
*/
public static DValue create (NexusObject owner, T value) {
return new DValue(owner, value);
}
@Override public void readContents (Streamable.Input in) {
_value = in.readValue();
}
@Override public void writeContents (Streamable.Output out) {
out.writeValue(_value);
}
protected DValue (NexusObject owner, T value) {
super(value);
_owner = owner;
_index = owner.registerAttr(this);
}
@Override protected void emitChange (T value, T oldValue) {
// we don't call super as we defer notification until the event is dispatched
ChangeEvent event = new ChangeEvent(_owner.getId(), _index, value);
event.oldValue = oldValue;
_owner.postEvent(event);
}
protected void applyChange (T value, T oldValue) {
if (oldValue == DistribUtil.sentinelValue()) {
// we came in over the network: read our old value and update _value
oldValue = updateLocal(value);
} // else: we were initiated in this JVM: _value was already updated
notifyChange(value, oldValue);
}
/** An event emitted when a value changes. */
protected static class ChangeEvent extends DAttribute.Event {
public T oldValue = DistribUtil.sentinelValue();
public ChangeEvent (int targetId, short index, T value) {
super(targetId, index);
_value = value;
}
@Override public void applyTo (NexusObject target) {
target.>getAttribute(this.index).applyChange(_value, oldValue);
}
@Override protected void toString (StringBuilder buf) {
super.toString(buf);
buf.append(", value=").append(_value);
}
protected final T _value;
}
/** The object that owns this attribute. */
protected final NexusObject _owner;
/** The index of this attribute in its containing object. */
protected final short _index;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy