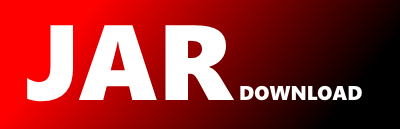
com.threerings.facebook.FBGraphUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ooo-facebook Show documentation
Show all versions of ooo-facebook Show documentation
Various Facebook helper bits used by OOO.
The newest version!
//
// $Id$
package com.threerings.facebook;
import java.io.IOException;
import java.net.URL;
import java.util.Iterator;
import java.util.Map;
import com.samskivert.io.StreamUtil;
import com.samskivert.net.HttpPostUtil;
import com.samskivert.util.ServiceWaiter;
import com.samskivert.util.StringUtil;
import com.google.common.collect.Maps;
import com.google.gson.Gson;
import static com.threerings.facebook.Log.log;
public class FBGraphUtil
{
public FBGraphUtil (String facebookAppId, String facebookSecret)
{
_fbAppId = facebookAppId;
_fbSecret = facebookSecret;
}
public String getThirdPartyId (String sessionKey, String fbUserId)
{
Map params = Maps.newHashMap();
params.put("client_id", _fbAppId);
params.put("client_secret", _fbSecret);
params.put("sessions", sessionKey);
SessionExchangeResult[] exchanges =
getResult(EXCHANGE_SESSION, params, SessionExchangeResult[].class, true);
if (exchanges == null || exchanges.length != 1) {
log.warning("Invalid session exchange result", "exchanges", exchanges);
return null;
}
params.clear();
params.put("fields", "third_party_id");
params.put("access_token", exchanges[0].access_token);
return getResult(fbUserId, params, ThirdPartyIdResult.class).third_party_id;
}
protected T getResult (String apiUrl, Map params, Class clazz)
{
return getResult(apiUrl, params, clazz, false);
}
/**
* Fetches the result from the Facebook Graph API and deserializes the result via json.
*/
protected T getResult (String apiUrl, Map params, Class clazz,
boolean httpPost)
{
Iterator> entryIter = params.entrySet().iterator();
StringBuilder paramString = new StringBuilder();
while (entryIter.hasNext()) {
Map.Entry entry = entryIter.next();
paramString.append(StringUtil.encode(entry.getKey())).append('=').append(
StringUtil.encode(entry.getValue()));
if (entryIter.hasNext()) {
paramString.append('&');
}
}
try {
apiUrl = GRAPH_API_URL + apiUrl;
String jsonString = httpPost ?
HttpPostUtil.httpPost(new URL(apiUrl), paramString.toString(), TIMEOUT) :
StreamUtil.toString(new URL(apiUrl + "?" + paramString.toString()).openStream());
return new Gson().fromJson(jsonString, clazz);
} catch (ServiceWaiter.TimeoutException e) {
log.warning("TimeoutException waiting graph request", "apiUrl", apiUrl,
"params", paramString, e);
} catch (IOException e) {
log.warning("IOException fetching graph request", "apiUrl", apiUrl,
"params", paramString, e);
}
return null;
}
protected static class SessionExchangeResult
{
public String access_token;
public int expires;
}
protected static class ThirdPartyIdResult
{
public String third_party_id;
public String id;
}
protected static final String GRAPH_API_URL = "https://graph.facebook.com/";
protected static final String EXCHANGE_SESSION = "oauth/exchange_sessions";
protected static final int TIMEOUT = 15*1000;
protected final String _fbAppId;
protected final String _fbSecret;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy