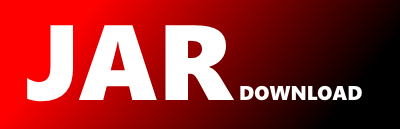
com.threerings.facebook.FacebookSessionApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ooo-facebook Show documentation
Show all versions of ooo-facebook Show documentation
Various Facebook helper bits used by OOO.
The newest version!
//
// $Id$
package com.threerings.facebook;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
import java.util.Set;
import com.google.code.facebookapi.FacebookException;
import com.google.code.facebookapi.FacebookJaxbRestClient;
import com.google.common.base.Function;
import com.google.common.base.Predicate;
import com.google.common.collect.Iterables;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
import static com.threerings.facebook.Log.log;
/**
* A class to perform common requests given a view into the Facebook API with a given person's
* sessionKey.
*/
public class FacebookSessionApi
{
public static FQL.Field UID = new FQL.Field("uid");
public static FQL.Field NAME = new FQL.Field("name");
public static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy