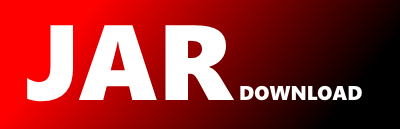
com.threewks.thundr.bigmetrics.bigquery.BigQueryService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thundr-bigmetrics Show documentation
Show all versions of thundr-bigmetrics Show documentation
A thundr module for supporting metrics in big query
The newest version!
/*
* This file is a component of thundr, a software library from 3wks.
* Read more: http://www.3wks.com.au/thundr
* Copyright (C) 2014 3wks,
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.threewks.thundr.bigmetrics.bigquery;
import java.util.List;
import java.util.Map;
/**
* Service abstracting the low level Google Big Query calls. This service is meant for Big Metrics internal use only
*/
public interface BigQueryService {
public static final String TABLE = "TABLE";
public static final String VIEW = "VIEW";
/**
* Defines a given job status
*/
public static enum JobStatus {
Running,
Success,
Error
}
/**
* Get the status of a job.
*
* @param jobId the id of the job.
* @return the job status.
*/
JobStatus getJobStatus(String jobId);
/**
* Execute a query.
*
* @param query the Bigquery SQL to execute.
* @return the job id of the query job.
*/
String executeQuery(String query);
/**
* Create a new table.
*
* @param tableId the table id.
* @param fields a map of field types.
*/
void createTable(String tableId, Map fields);
/**
* Get the schema of the given table.
*
* @param tableId the id of the table to get.
* @return a map of field names to field types.
*/
Map getTableSchema(String tableId);
/**
* Create a view from the given query.
*
* @param viewId the id of the view to save.
* @param query the query to execute to create the view.
*/
void createView(String viewId, String query);
/**
* Updates the given view to the given query.
*
* @param viewId the id of the view to change.
* @param query the query to execute to create the view.
*/
void updateView(String viewId, String query);
/**
* Get the results of a query.
*
* @param jobId the query job id.
* @param pageSize the page size.
* @param pageToken the page token.
* @return the query results.
*/
QueryResult queryResult(String jobId, long pageSize, String pageToken);
ExportResult exportQueryResults(String jobId, String bucketName, String objectName);
/**
* Insert data into a table.
*
* @param insertId the unique token for the insert (usually the header id taken from the queue that calls this method)
* @param tableId the table id.
* @param data the data to insert into the table.
*/
void insert(String insertId, String tableId, Map data);
String getDatasetId();
/**
* List all tables.
*
* Does not include views.
*
* @return a list of all tables
* @see #listViews()
*/
List listTables();
/**
* List the tables whose name matches the given regular expression.
* Does not include views
*
* @param matching regex string to match
* @return the list of tables matching the given regex
* @see #listViews(String)
*/
List listTables(String matching);
/**
* List all views
*
* @return a list of all views
*/
List listViews();
/**
* List the views whose name matches the given regular expression.
*
* @param matching regex string to match
* @return a list of views matching the given regex
*/
List listViews(String matching);
/**
* Returns the query which defines the specific view, or null if the view doesn't exist.
*
* @param viewId the view id
* @return the view definition
*/
public String getViewDefinition(String viewId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy