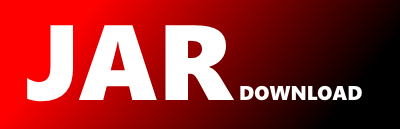
com.threewks.thundr.bigmetrics.field.DateTimeFieldProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thundr-bigmetrics Show documentation
Show all versions of thundr-bigmetrics Show documentation
A thundr module for supporting metrics in big query
The newest version!
/*
* This file is a component of thundr, a software library from 3wks.
* Read more: http://www.3wks.com.au/thundr
* Copyright (C) 2014 3wks,
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.threewks.thundr.bigmetrics.field;
import java.util.LinkedHashMap;
import java.util.Map;
import org.joda.time.DateTime;
import org.joda.time.format.DateTimeFormat;
import org.joda.time.format.DateTimeFormatter;
import com.atomicleopard.expressive.Expressive;
import com.threewks.thundr.bigmetrics.bigquery.BigQueryType;
/**
* This {@link FieldProcessor} denormalises the date so that as well as being stored as a timestamp,
* individual components of the date are also stored.
*/
public class DateTimeFieldProcessor implements FieldProcessor {
public static final DateTimeFormatter TimestampFormat = DateTimeFormat.forPattern("yyyy-MM-dd HH:mm:ss.SSS").withZoneUTC();
private Map namedFormatters = new LinkedHashMap<>();
public DateTimeFieldProcessor() {
namedFormatters.put("year", DateTimeFormat.forPattern("yyyy").withZoneUTC());
namedFormatters.put("month", DateTimeFormat.forPattern("MM").withZoneUTC());
namedFormatters.put("day", DateTimeFormat.forPattern("dd").withZoneUTC());
namedFormatters.put("weekday", DateTimeFormat.forPattern("e").withZoneUTC());
namedFormatters.put("hour", DateTimeFormat.forPattern("HH").withZoneUTC());
namedFormatters.put("minute", DateTimeFormat.forPattern("mm").withZoneUTC());
namedFormatters.put("second", DateTimeFormat.forPattern("ss").withZoneUTC());
namedFormatters.put("millis", DateTimeFormat.forPattern("SSS").withZoneUTC());
namedFormatters.put("zone", DateTimeFormat.forPattern("ZZ").withZoneUTC());
}
@Override
public Map process(String name, BigQueryType type, DateTime data) {
Map denormalised = new LinkedHashMap<>();
denormalised.put(name, data == null ? null : TimestampFormat.print(data));
for (Map.Entry formatter : namedFormatters.entrySet()) {
denormalised.put(name + "_" + formatter.getKey(), data == null ? null : formatter.getValue().print(data));
}
return denormalised;
}
@Override
public Map fields(String name, BigQueryType type) {
// @formatter:off
return Expressive.map(
name, BigQueryType.Timestamp,
name+ "_year", BigQueryType.Integer,
name+ "_month", BigQueryType.Integer,
name+ "_day", BigQueryType.Integer,
name+ "_weekday",BigQueryType.Integer,
name+ "_hour", BigQueryType.Integer,
name+ "_minute",BigQueryType.Integer,
name+ "_second",BigQueryType.Integer,
name+ "_millis",BigQueryType.Integer,
name+ "_zone", BigQueryType.String
);
// @formatter:on
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy