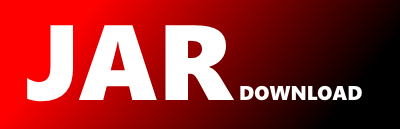
com.threewks.thundr.http.service.BaseHttpRequest Maven / Gradle / Ivy
/*
* This file is a component of thundr, a software library from 3wks.
* Read more: http://3wks.github.io/thundr/
* Copyright (C) 2014 3wks,
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.threewks.thundr.http.service;
import java.net.HttpCookie;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import com.threewks.thundr.http.Authorization;
import com.threewks.thundr.http.ContentType;
import com.threewks.thundr.http.Header;
public abstract class BaseHttpRequest> implements HttpRequest {
protected BaseHttpService, Self, ?> httpService;
@SuppressWarnings("unchecked")
private Self self = (Self) this;
protected String url;
protected Map headers = new LinkedHashMap<>();
protected Collection cookies = new ArrayList<>();
protected Map parameters = new LinkedHashMap<>();
protected List fileParameters = Collections.emptyList();
protected Object body = null;
protected long timeout = 60000;
protected boolean followRedirects = true;
protected String username;
protected String password;
protected String scheme;
protected BaseHttpRequest(BaseHttpService, Self, ?> httpService, String url) {
this.url = url;
this.httpService = httpService;
}
protected BaseHttpRequest(BaseHttpService, Self, ?> httpService, String url, Map headers, Collection cookies, Map parameters,
List fileParameters, Object body, long timeout, boolean followRedirects, String username, String password, String scheme) {
super();
this.httpService = httpService;
this.url = url;
this.headers = headers;
this.cookies = cookies;
this.parameters = parameters;
this.fileParameters = fileParameters;
this.body = body;
this.timeout = timeout;
this.followRedirects = followRedirects;
this.username = username;
this.password = password;
this.scheme = scheme;
}
protected abstract Self createInstance(BaseHttpService, Self, ?> httpService, String url, Map headers, Collection cookies, Map parameters,
List fileParameters, Object body, long timeout, boolean followRedirects, String username, String password, String scheme);
@Override
public Self followRedirects(boolean followRedirects) {
return createInstance(httpService, url, headers, cookies, parameters, fileParameters, body, timeout, followRedirects, username, password, scheme);
}
@Override
public HttpRequest timeout(long timeout) {
return createInstance(httpService, url, headers, cookies, parameters, fileParameters, body, timeout, followRedirects, username, password, scheme);
}
@Override
public HttpRequest body(Object body) {
return createInstance(httpService, url, headers, cookies, parameters, fileParameters, body, timeout, followRedirects, username, password, scheme);
}
@Override
public HttpRequest header(String name, String value) {
return this.headers(Collections.singletonMap(name, value));
}
@Override
public HttpRequest headers(Map header) {
Map headers = new LinkedHashMap<>(this.headers);
headers.putAll(header);
return createInstance(httpService, url, headers, cookies, parameters, fileParameters, body, timeout, followRedirects, username, password, scheme);
}
@Override
public HttpRequest parameter(String name, String value) {
return this.parameter(name, (Object) value);
}
@Override
public HttpRequest parameter(String name, Object value) {
return this.parameters(Collections.singletonMap(name, value));
}
@Override
public HttpRequest parameters(Map parameters) {
Map newParameters = new LinkedHashMap<>(this.parameters);
newParameters.putAll(parameters);
return createInstance(httpService, url, headers, cookies, newParameters, fileParameters, body, timeout, followRedirects, username, password, scheme);
}
@Override
public HttpRequest contentType(ContentType contentType) {
return this.header(Header.ContentType, contentType.value());
}
@Override
public HttpRequest contentType(String contentType) {
return this.header(Header.ContentType, contentType);
}
@Override
public HttpRequest cookie(HttpCookie cookie) {
return this.cookies(Collections.singletonList(cookie));
}
@Override
public HttpRequest cookies(Collection cookie) {
List cookies = new ArrayList(this.cookies);
cookies.addAll(cookie);
return createInstance(httpService, url, headers, cookies, parameters, fileParameters, body, timeout, followRedirects, username, password, scheme);
}
@Override
public HttpRequest file(String name, String filename, ContentType contentType, Object view) {
return this.file(name, filename, contentType.value(), view);
}
@Override
public HttpRequest file(String name, String filename, String contentType, Object view) {
List fileParameters = new ArrayList<>(this.fileParameters);
fileParameters.add(new FileParameter(name, filename, contentType, view));
return createInstance(httpService, url, headers, cookies, parameters, fileParameters, body, timeout, followRedirects, username, password, scheme);
}
@Override
public HttpResponse get() {
return httpService.get(self);
}
@Override
public HttpResponse post() {
return httpService.post(self);
}
@Override
public HttpResponse put() {
return httpService.put(self);
}
@Override
public HttpResponse delete() {
return httpService.delete(self);
}
@Override
public HttpResponse head() {
return httpService.head(self);
}
@Override
public HttpRequest authorize(String username, String password) {
return authorize(username, password, Authorization.Basic);
}
@Override
public HttpRequest authorize(String username, String password, String scheme) {
return createInstance(httpService, url, headers, cookies, parameters, fileParameters, body, timeout, followRedirects, username, password, scheme);
}
public String getUrl() {
return url;
}
public Map getHeaders() {
return headers;
}
public Collection getCookies() {
return cookies;
}
public Map getParameters() {
return parameters;
}
public List getFileParameters() {
return fileParameters;
}
public Object getBody() {
return body;
}
public long getTimeout() {
return timeout;
}
public boolean isFollowRedirects() {
return followRedirects;
}
public String getUsername() {
return username;
}
public String getPassword() {
return password;
}
public String getScheme() {
return scheme;
}
public BaseHttpService, Self, ?> getHttpService() {
return httpService;
}
public String getHeader(String header) {
for (Map.Entry entry : headers.entrySet()) {
if (entry.getKey().equalsIgnoreCase(header)) {
return entry.getValue();
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy