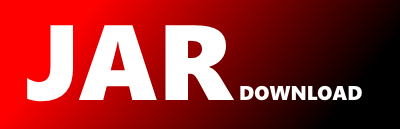
com.threewks.thundr.http.service.BaseHttpService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thundr-http Show documentation
Show all versions of thundr-http Show documentation
Provides an API for http connectivity functionality for thundr projects.
/*
* This file is a component of thundr, a software library from 3wks.
* Read more: http://3wks.github.io/thundr/
* Copyright (C) 2014 3wks,
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.threewks.thundr.http.service;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.net.HttpCookie;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang3.StringUtils;
import org.apache.http.HttpEntity;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.entity.mime.content.ByteArrayBody;
import org.apache.http.entity.mime.content.ContentBody;
import com.atomicleopard.expressive.ETransformer;
import com.atomicleopard.expressive.Expressive;
import com.threewks.thundr.http.Authorization;
import com.threewks.thundr.http.ContentType;
import com.threewks.thundr.http.Header;
import com.threewks.thundr.http.URLEncoder;
import com.threewks.thundr.transformer.TransformerManager;
public abstract class BaseHttpService, Request extends BaseHttpRequest, Response extends BaseHttpResponse> implements
HttpService {
protected List HttpMethodsWithoutBodies = Expressive.list("GET", "HEAD", "OPTIONS", "DELETE");
protected List HttpMethodsWithBodies = Expressive.list("POST", "PUT");
protected String encoding = "UTF-8";
protected TransformerManager transformerManager;
protected BaseHttpService(TransformerManager transformerManager) {
this.transformerManager = transformerManager;
}
@Override
public abstract Request request(String url);
protected abstract Response head(Request request) throws HttpException;
protected abstract Response get(Request request) throws HttpException;
protected abstract Response put(Request request) throws HttpException;
protected abstract Response post(Request request) throws HttpException;
protected abstract Response delete(Request request) throws HttpException;
protected HttpEntity prepareMultipart(Request request) {
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
for (Map.Entry parameter : request.getParameters().entrySet()) {
String value = getValueAs(parameter.getValue(), String.class);
builder.addTextBody(parameter.getKey(), value, org.apache.http.entity.ContentType.create("text/plain", "UTF-8"));
}
for (FileParameter fileParam : request.getFileParameters()) {
Object body = fileParam.getBody();
byte[] data = getValueAs(body, byte[].class);
org.apache.http.entity.ContentType contentType = org.apache.http.entity.ContentType.create(fileParam.getContentType());
ContentBody contentBody = new ByteArrayBody(data, contentType, fileParam.getFilename());
builder.addPart(fileParam.getName(), contentBody);
}
HttpEntity httpEntity = builder.build();
return httpEntity;
}
@SuppressWarnings("unchecked")
protected T getValueAs(Object value, Class desiredType) {
Class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy