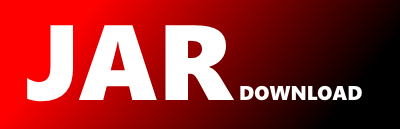
com.threewks.thundr.http.service.BaseHttpResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thundr-http Show documentation
Show all versions of thundr-http Show documentation
Provides an API for http connectivity functionality for thundr projects.
The newest version!
/*
* This file is a component of thundr, a software library from 3wks.
* Read more: http://3wks.github.io/thundr/
* Copyright (C) 2015 3wks,
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.threewks.thundr.http.service;
import java.net.HttpCookie;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.commons.lang3.StringUtils;
import com.threewks.thundr.logger.Logger;
public abstract class BaseHttpResponse> implements HttpResponse {
private static Pattern charsetPattern = Pattern.compile(".*?charset=([^;,\\s]+).*");
protected BaseHttpService, ?, Self> httpService;
public BaseHttpResponse(BaseHttpService, ?, Self> httpService) {
this.httpService = httpService;
}
@Override
public T getBody(Class as) {
return httpService.convertIncoming(getBodyAsStream(), as);
}
/**
* Safely parse a cookie header. If any exceptions are encountered then the exception is caught
* and an empty list is returned.
*
* @param setCookieHeader the header to parse.
* @return a list of cookies, or an empty list if anything goes wrong parsing the header.
*/
protected List parseCookies(String setCookieHeader) {
List cookies = new ArrayList();
if (StringUtils.isNotBlank(setCookieHeader)) {
try {
cookies = HttpCookie.parse(setCookieHeader);
} catch (Exception e) {
try {
// old version of java (<7) fail for cookies with HttpOnly present, we'll strip that out and try again
cookies = HttpCookie.parse(setCookieHeader.replaceAll("(?i);\\s*HttpOnly", ""));
} catch (Exception e2) {
Logger.warn("Unable to parse cookie from header '%s': %s", setCookieHeader, e2.getMessage());
}
}
}
return cookies;
}
protected String getCharset() {
String fullContentType = getContentType();
if (fullContentType != null) {
Matcher matcher = charsetPattern.matcher(fullContentType);
if (matcher.matches()) {
return matcher.group(1);
}
}
return "UTF-8";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy