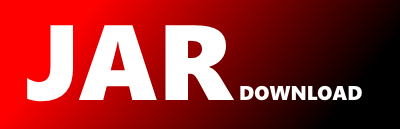
com.threewks.thundr.http.url.HttpResponseUrlConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thundr-http Show documentation
Show all versions of thundr-http Show documentation
Provides an API for http connectivity functionality for thundr projects.
The newest version!
/*
* This file is a component of thundr, a software library from 3wks.
* Read more: http://3wks.github.io/thundr/
* Copyright (C) 2015 3wks,
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.threewks.thundr.http.url;
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.net.HttpCookie;
import java.net.HttpURLConnection;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Collections;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang3.StringUtils;
import com.atomicleopard.expressive.EList;
import com.atomicleopard.expressive.Expressive;
import com.threewks.thundr.http.Header;
import com.threewks.thundr.http.service.BaseHttpResponse;
import com.threewks.thundr.http.service.HttpException;
import com.threewks.thundr.http.service.HttpResponse;
public class HttpResponseUrlConnection extends BaseHttpResponse implements HttpResponse {
private HttpURLConnection urlConnection;
private HashMap> cookies;
public HttpResponseUrlConnection(HttpServiceUrlConnection httpService, HttpURLConnection urlConnection) {
super(httpService);
this.urlConnection = urlConnection;
}
@Override
public int getStatus() {
try {
return urlConnection.getResponseCode();
} catch (IOException e) {
throw new HttpException(e, "Failed to read response code for %s %s: %s", urlConnection.getRequestMethod(), urlConnection.getURL(), e.getMessage());
}
}
@Override
public String getContentType() {
return urlConnection.getContentType();
}
@Override
public String getHeader(String name) {
return urlConnection.getHeaderField(name);
}
@Override
public List getHeaders(String name) {
Map> headers = getHeaders();
for (Map.Entry> entry : headers.entrySet()) {
if (StringUtils.equalsIgnoreCase(entry.getKey(), name)) {
return entry.getValue();
}
}
return Collections. emptyList();
}
@Override
public Map> getHeaders() {
return urlConnection.getHeaderFields();
}
@Override
public HttpCookie getCookie(String name) {
EList cookies = cookies().get(name);
return cookies == null ? null : cookies.first();
}
@Override
public List getCookies(String name) {
EList cookies = cookies().get(name);
return cookies;
}
@Override
public List getCookies() {
return Expressive.flatten(cookies().values());
}
@Override
public String getBody() {
String contentEncoding = urlConnection.getContentEncoding();
contentEncoding = contentEncoding == null ? "UTF-8" : contentEncoding;
try {
return new String(getBodyAsBytes(), contentEncoding);
} catch (UnsupportedEncodingException e) {
throw new HttpException(e, "Failed to read body as string for %s %s - character encoding '%s' not supported: %s", urlConnection.getRequestMethod(), urlConnection.getURL(),
contentEncoding, e.getMessage());
}
}
@Override
public byte[] getBodyAsBytes() {
return com.threewks.thundr.util.Streams.readBytes(getBodyAsStream());
}
@Override
public InputStream getBodyAsStream() {
try {
InputStream errorStream = urlConnection.getErrorStream();
return errorStream == null ? urlConnection.getInputStream() : errorStream;
} catch (IOException e) {
throw new HttpException(e, "Failed to read body for %s %s: %s", urlConnection.getRequestMethod(), urlConnection.getURL(), e.getMessage());
}
}
@Override
public URI getUri() {
try {
return urlConnection.getURL().toURI();
} catch (URISyntaxException e) {
throw new HttpException(e, "Failed to convert request url to uri for %s %s: %s", urlConnection.getRequestMethod(), urlConnection.getURL(), e.getMessage());
}
}
Map> cookies() {
if (cookies == null) {
cookies = new LinkedHashMap>();
for (String setCookieHeader : getCookieHeaders()) {
List cookieSet = parseCookies(setCookieHeader);
for (HttpCookie httpCookie : cookieSet) {
String name = httpCookie.getName();
EList existingCookies = cookies.get(name);
if (existingCookies == null) {
existingCookies = Expressive.list();
cookies.put(name, existingCookies);
}
existingCookies.add(httpCookie);
}
}
}
return cookies;
}
protected EList getCookieHeaders() {
List cookies = getHeaders(Header.SetCookie);
List cookies2 = getHeaders(Header.SetCookie2);
EList cookieHeaders = Expressive.list();
if (cookies != null) {
cookieHeaders.addItems(cookies);
}
if (cookies2 != null) {
cookieHeaders.addItems(cookies2);
}
return cookieHeaders;
}
@Override
public String toString() {
return String.format("%s %s - %s (%s)", urlConnection.getRequestMethod(), urlConnection.getURL(), getStatus(), getContentType());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy