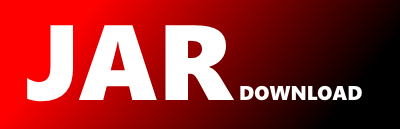
com.threewks.thundr.http.url.HttpServiceUrlConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thundr-http Show documentation
Show all versions of thundr-http Show documentation
Provides an API for http connectivity functionality for thundr projects.
The newest version!
/*
* This file is a component of thundr, a software library from 3wks.
* Read more: http://3wks.github.io/thundr/
* Copyright (C) 2015 3wks,
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.threewks.thundr.http.url;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.ProtocolException;
import java.net.URL;
import java.util.Map;
import org.apache.http.HttpEntity;
import com.threewks.thundr.http.ContentType;
import com.threewks.thundr.http.Header;
import com.threewks.thundr.http.service.BaseHttpService;
import com.threewks.thundr.http.service.HttpException;
import com.threewks.thundr.http.service.HttpService;
import com.threewks.thundr.transformer.TransformerManager;
import com.threewks.thundr.util.Streams;
public class HttpServiceUrlConnection extends BaseHttpService implements HttpService {
public HttpServiceUrlConnection(TransformerManager transformerManager) {
super(transformerManager);
}
@Override
public HttpRequestUrlConnection request(String url) {
return new HttpRequestUrlConnection(this, url);
}
@Override
protected HttpResponseUrlConnection head(HttpRequestUrlConnection request) throws HttpException {
return getInternal(request, "HEAD");
}
@Override
protected HttpResponseUrlConnection get(HttpRequestUrlConnection request) throws HttpException {
return getInternal(request, "GET");
}
@Override
protected HttpResponseUrlConnection put(HttpRequestUrlConnection request) throws HttpException {
return postInternal(request, "PUT");
}
@Override
protected HttpResponseUrlConnection post(HttpRequestUrlConnection request) throws HttpException {
return postInternal(request, "POST");
}
@Override
protected HttpResponseUrlConnection delete(HttpRequestUrlConnection request) throws HttpException {
return getInternal(request, "DELETE");
}
protected HttpResponseUrlConnection getInternal(HttpRequestUrlConnection request, String method) {
String urlString = buildUrlString(request, method);
try {
HttpURLConnection urlConnection = open(request, method, urlString);
setContentTypeIfNotPresent(urlConnection, ContentType.TextPlain);
addAuthorization(urlConnection, request);
addHeaders(urlConnection, request);
addCookies(urlConnection, request);
return new HttpResponseUrlConnection(this, urlConnection);
} catch (Exception e) {
throw new HttpException(e, "Failed to %s url %s: %s", method, request.getUrl(), e.getMessage());
}
}
public HttpResponseUrlConnection postInternal(HttpRequestUrlConnection request, String method) {
String urlString = buildUrlString(request, method);
try {
HttpURLConnection urlConnection = open(request, method, urlString);
setContentTypeIfNotPresent(urlConnection, ContentType.ApplicationFormUrlEncoded);
addAuthorization(urlConnection, request);
addHeaders(urlConnection, request);
addCookies(urlConnection, request);
addBody(urlConnection, request);
return new HttpResponseUrlConnection(this, urlConnection);
} catch (Exception e) {
throw new HttpException(e, "Failed to %s url %s: %s", method, request.getUrl(), e.getMessage());
}
}
private HttpURLConnection open(HttpRequestUrlConnection request, String method, String urlString) throws IOException, ProtocolException {
URL url = new URL(urlString);
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
urlConnection.setInstanceFollowRedirects(request.isFollowRedirects());
urlConnection.setConnectTimeout((int) request.getTimeout());
urlConnection.setAllowUserInteraction(false);
urlConnection.setRequestMethod(method.toUpperCase());
urlConnection.setDoInput(true);
return urlConnection;
}
protected void setContentTypeIfNotPresent(HttpURLConnection urlConnection, ContentType contentType) {
urlConnection.addRequestProperty(Header.ContentType, contentType.value());
}
protected void addAuthorization(HttpURLConnection urlConnection, HttpRequestUrlConnection request) {
String authorization = getAuthorization(request);
if (authorization != null) {
urlConnection.setRequestProperty(Header.Authorization, authorization);
}
}
protected void addCookies(HttpURLConnection urlConnection, HttpRequestUrlConnection request) {
String cookieStrings = createCookieHeaderValue(request);
if (cookieStrings != null) {
urlConnection.setRequestProperty("Cookie", cookieStrings);
}
}
protected void addHeaders(HttpURLConnection urlConnection, HttpRequestUrlConnection request) {
Map headers = request.getHeaders();
for (Map.Entry header : headers.entrySet()) {
urlConnection.setRequestProperty(header.getKey(), header.getValue());
}
}
protected void addBody(HttpURLConnection urlConnection, HttpRequestUrlConnection request) throws IOException {
Object body = request.getBody();
OutputStream outputStream;
if (isMultipart(request)) {
HttpEntity multipart = prepareMultipart(request);
long contentLength = multipart.getContentLength();
urlConnection.setDoOutput(true);
if (contentLength > -1) {
urlConnection.setFixedLengthStreamingMode(contentLength);
urlConnection.addRequestProperty(Header.ContentLength, contentLength + "");
} else {
urlConnection.setChunkedStreamingMode(1024);
}
urlConnection.setRequestProperty(Header.ContentType, multipart.getContentType().getValue());
outputStream = urlConnection.getOutputStream();
multipart.writeTo(outputStream);
} else if (body == null) {
// if the body has been explicitly set, the consumer is handling data encoding,
// otherwise we assume its a form post
String stringBody = createFormPostBody(request);
byte[] data = stringBody.getBytes(encoding);
urlConnection.setFixedLengthStreamingMode(data.length);
urlConnection.addRequestProperty(Header.ContentLength, data.length + "");
urlConnection.setDoOutput(true);
outputStream = urlConnection.getOutputStream();
outputStream.write(data);
} else {
urlConnection.setChunkedStreamingMode(1024);
urlConnection.setDoOutput(true);
outputStream = urlConnection.getOutputStream();
InputStream data = convertOutgoing(body);
Streams.copy(data, outputStream);
}
outputStream.flush();
outputStream.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy