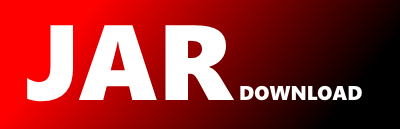
com.tibco.bw.maven.plugin.admin.dto.Domain Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bw6-maven-plugin Show documentation
Show all versions of bw6-maven-plugin Show documentation
Plugin Code for Apache Maven and TIBCO BusinessWorks™.
This is the Maven Plugin for BW6 and BWCE Build.
/*
* Copyright(c) 2014 TIBCO Software Inc.
* All rights reserved.
*
* This software is confidential and proprietary information of TIBCO Software Inc.
*
*/
package com.tibco.bw.maven.plugin.admin.dto;
import java.util.List;
import javax.xml.bind.annotation.XmlElement;
/**
* @author Tim Diekmann
*
* @since 1.0.0
*/
public class Domain {
private String name;
private String description;
private String date;
private String owner;
private boolean full;
private List configRefs;
private List appspaceRefs;
private List configs;
private List appspaces;
public Domain() {
}
/**
* @return the full
*/
@XmlElement
public boolean isFull() {
return this.full;
}
/**
* @param full
* the full to set
*/
public void setFull(final boolean full) {
this.full = full;
}
/**
* @return the configRefs
*/
@XmlElement
public List getConfigRefs() {
return this.configRefs;
}
/**
* @param configRefs
* the configRefs to set
*/
public void setConfigRefs(final List configRefs) {
this.configRefs = configRefs;
}
/**
* @return the appspaceRefs
*/
@XmlElement
public List getAppspaceRefs() {
return this.appspaceRefs;
}
/**
* @param appspaceRefs
* the appspaceRefs to set
*/
public void setAppspaceRefs(final List appspaceRefs) {
this.appspaceRefs = appspaceRefs;
}
/**
* @return the configs
*/
@XmlElement
public List getConfigs() {
return this.configs;
}
/**
* @param configs
* the configs to set
*/
public void setConfigs(final List configs) {
this.configs = configs;
}
@XmlElement
public String getName() {
return this.name;
}
@XmlElement
public String getOwner() {
return this.owner;
}
@XmlElement
public String getDate() {
return this.date;
}
@XmlElement
public String getDescription() {
return this.description;
}
/**
* @param name
* the name to set
*/
public void setName(final String name) {
this.name = name;
}
/**
* @param description
* the description to set
*/
public void setDescription(final String description) {
this.description = description;
}
/**
* @param dateTime
* and time at which this domain is created the date to set
*/
public void setDate(final String dateTime) {
this.date = dateTime;
}
/**
* @param owner
* who created this domain the owner to set
*/
public void setOwner(final String owner) {
this.owner = owner;
}
/**
* @return all the AppSpaces that are part of this domain
*/
@XmlElement
public List getAppspaces() {
return this.appspaces;
}
/**
* @param appspaces
* the list of AppSpaces to set
*/
public void setAppspaces(final List appspaces) {
this.appspaces = appspaces;
}
/**
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return String.format("com.tibco.bw.maven.plugin.admin.dto.Domain(%s)", this.name);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy