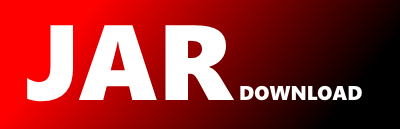
com.tibco.bw.maven.plugin.admin.dto.Application Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bw6-maven-plugin Show documentation
Show all versions of bw6-maven-plugin Show documentation
Plugin Code for Apache Maven and TIBCO BusinessWorks™.
This is the Maven Plugin for BW6 and BWCE Build.
/*
* Copyright(c) 2014 TIBCO Software Inc.
* All rights reserved.
*
* This software is confidential and proprietary information of TIBCO Software Inc.
*
*/
package com.tibco.bw.maven.plugin.admin.dto;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlElement;
/**
* DTO for Application
*
* @author Tim Diekmann
*
* @since 1.0.0
*/
public class Application {
public enum ApplicationDeploymentStates {
Deployed, DeployFailed, UndeployFailed, OutOfSync
}
public enum ApplicationRuntimeStates {
Running, Stopped, Impaired, FlowControlled, Paused, StartFailed, AppError, DeployFailed, Unreachable, Deploying, Stopping, Degraded, Starting
}
public enum ApplicationConfigurationStates {
InSync, OutOfSync
}
private String name;
private String appSpaceName;
private String domainName;
private String version;
private String description;
private HRef archiveRef;
private List configuration;
private int revision;
private ApplicationRuntimeStates state; // this is the aggregate runtime status of the application
private String archiveName;
private String archivePath;
private String profileName;
private String docURL;
private ApplicationDeploymentStates deploymentStatus;
private List deploymentStatusDetail;
private List instances;
private HRef profileContentRef; // href to get the profile configured for a given application
private HRef href; // href to the application to be used to get/undeploy operations and as a baseurl for start/stop actions
private Collection components;
private Collection processes;
private String message;
private String code;
public Application() {
this.instances = new ArrayList();
this.deploymentStatusDetail = new ArrayList();
this.components = new ArrayList<>();
this.processes = new ArrayList<>();
}
/**
* @return the name
*/
@XmlElement
public String getName() {
return this.name;
}
/**
* @param name
* the name to set
*/
public void setName(final String name) {
this.name = name;
}
/**
* @return the appSpaceName
*/
@XmlElement
public String getAppSpaceName() {
return this.appSpaceName;
}
/**
* @param appSpaceName
* the appSpaceName to set
*/
public void setAppSpaceName(final String appSpaceName) {
this.appSpaceName = appSpaceName;
}
/**
* @return the domainName
*/
@XmlElement
public String getDomainName() {
return this.domainName;
}
/**
* @param domainName
* the domainName to set
*/
public void setDomainName(final String domainName) {
this.domainName = domainName;
}
/**
* @return the version
*/
@XmlElement
public String getVersion() {
return this.version;
}
/**
* @param version
* the version to set
*/
public void setVersion(final String version) {
this.version = version;
}
/**
* @return the description
*/
@XmlElement
public String getDescription() {
return this.description;
}
/**
* @param description
* the description to set
*/
public void setDescription(final String description) {
this.description = description;
}
/**
* @return the earFileRef
*/
@XmlElement
public HRef getArchiveRef() {
return this.archiveRef;
}
/**
* @param earFileRef
* the earFileRef to set
*/
public void setArchiveRef(final HRef earFileRef) {
this.archiveRef = earFileRef;
}
/**
* @return the configuration
*/
@XmlElement
public List getConfiguration() {
return this.configuration;
}
/**
* @param configuration
* the configuration to set
*/
public void setConfiguration(final List configuration) {
this.configuration = configuration;
}
/**
* @return the revision
*/
@XmlElement
public int getRevision() {
return this.revision;
}
/**
* @param revision
* the revision to set
*/
public void setRevision(final int revision) {
this.revision = revision;
}
/**
* @return the status
*/
@XmlElement
public ApplicationRuntimeStates getState() {
return this.state;
}
/**
* @param state
* the status to set
*/
public void setState(final ApplicationRuntimeStates state) {
this.state = state;
}
/**
* @return the archiveName
*/
@XmlElement
public String getArchiveName() {
return this.archiveName;
}
/**
* @param earFileName
* the earFileName to set
*/
public void setArchiveName(final String earFileName) {
this.archiveName = earFileName;
}
/**
* @return the archivePath
*/
@XmlElement
public String getArchivePath() {
return this.archivePath;
}
/**
* @param earFilePath
* the earFilePath to set
*/
public void setArchivePath(final String earFilePath) {
this.archivePath = earFilePath;
}
/**
* @return the profileName
*/
@XmlElement
public String getProfileName() {
return this.profileName;
}
/**
* @param profileName
* the profileName to set
*/
public void setProfileName(final String profileName) {
this.profileName = profileName;
}
/**
* @return the deploymentStatus
*/
@XmlElement
public ApplicationDeploymentStates getDeploymentStatus() {
return this.deploymentStatus;
}
/**
* @param deploymentStatus
* the deploymentStatus to set
*/
public void setDeploymentStatus(final ApplicationDeploymentStates deploymentStatus) {
this.deploymentStatus = deploymentStatus;
}
/**
* @return the deploymentStatusDetail
*/
@XmlElement
public List getDeploymentStatusDetail() {
return this.deploymentStatusDetail;
}
/**
* @param deploymentStatusDetail
* the deploymentStatusDetail to set
*/
public void setDeploymentStatusDetail(final List deploymentStatusDetail) {
this.deploymentStatusDetail = deploymentStatusDetail;
}
/**
* @return the instances
*/
@XmlElement
public List getInstances() {
return this.instances;
}
/**
* @param instances
* the instances to set
*/
public void setAppInstances(final List instances)
{
this.instances = instances;
}
/**
* @return the profileContent
*/
@XmlElement
public HRef getProfileContentRef() {
return this.profileContentRef;
}
/**
* @param profileContent
* the profileContent to set
*/
public void setProfileContentRef(final HRef profileContent) {
this.profileContentRef = profileContent;
}
/**
* @return the url to the application
*/
@XmlElement
public HRef getRef() {
return this.href;
}
/**
* @param href
* the href to set
*/
public void setRef(final HRef href) {
this.href = href;
}
/**
* @return the docURL
*/
@XmlElement
public String getDocURL() {
return this.docURL;
}
/**
* @param docURL
* the docURL to set
*/
public void setDocURL(final String docURL) {
this.docURL = docURL;
}
/**
* @return the components
*/
@XmlElement
public Collection getComponents() {
return this.components;
}
/**
* @param components
* the components to set
*/
public void setComponents(final Collection components) {
this.components = components;
}
/**
* @return the processes
*/
@XmlElement
public Collection getProcesses() {
return this.processes;
}
/**
* @param processes
* the processes to set
*/
public void setProcesses(final Collection processes) {
this.processes = processes;
}
/**
* @return the message
*/
@XmlElement
public String getMessage() {
return message;
}
/**
*
* @param message
* the message to set
*/
public void setMessage(String message) {
this.message = message;
}
/**
*
* @return the cpde
*/
@XmlElement
public String getCode() {
return code;
}
/**
*
* @param code
* the code to set
*/
public void setCode(String code) {
this.code = code;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy