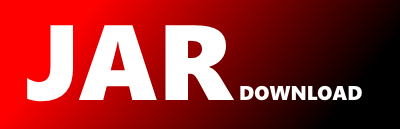
com.tibco.bw.maven.plugin.admin.dto.Machine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bw6-maven-plugin Show documentation
Show all versions of bw6-maven-plugin Show documentation
Plugin Code for Apache Maven and TIBCO BusinessWorks™.
This is the Maven Plugin for BW6 and BWCE Build.
package com.tibco.bw.maven.plugin.admin.dto;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlElement;
/**
*
* Copyright (c) 2014 TIBCO Software Inc. All Rights Reserved.
*
* @author Rohit Pegallapati
*
*
*/
public class Machine {
private String name;
// private String description;
private String ipAddress;
private MachineStatus status;
private List appNodes;
private List agents;
private List installationRefs;
private List agentRefs;
private List appNodeRefs;
private List appSpaceRefs;
private List domainRefs;
private List archiveRefs;
private String os;
private String osPatchLevel;
// private boolean full;
public Machine() {
}
@XmlElement
public String getName() {
return this.name;
}
/**
* @param name
* the name to set
*/
public void setName(final String name) {
this.name = name;
}
/**
* @param ipAddress
* the ipAddress to set
*/
public void setIpAddress(final String ipAddress) {
this.ipAddress = ipAddress;
}
/**
* @return the ipAddress
*/
@XmlElement
public String getIpAddress() {
return this.ipAddress;
}
/**
* @return the installations
*/
/*
* @XmlElement public Collection getInstallations() { return
* this.installations; }
*
*
* /**
*
* @param installations the installations to set
*/
/*
* public void setInstallations(final Collection
* installations) { this.installations = installations != null ?
* installations : Collections.EMPTY_LIST; }
*
* public void addInstallation(final Installation installation) {
* this.installations.add(installation); }
*/
/**
*
* @param installationRefs the installrefs
*/
public void setInstallationRefs(final List installationRefs) {
this.installationRefs = installationRefs;
}
@XmlElement
public void setDomainRefs(final List domainRefs) {
this.domainRefs = domainRefs;
}
public void setAppSpaceRefs(final List appSpaceRefs) {
this.appSpaceRefs = appSpaceRefs;
}
@XmlElement
public void setArchiveRefs(final List archiveRefs) {
this.archiveRefs = archiveRefs;
}
@XmlElement
public List getDomainRefs() {
return this.domainRefs;
}
@XmlElement
public List getAppSpaceRefs() {
return this.appSpaceRefs;
}
@XmlElement
public List getArchiveRefs() {
return this.archiveRefs;
}
@XmlElement
public List getInstallationRefs() {
return this.installationRefs;
}
@XmlElement
public void setAgentRefs(final List agentRefs) {
this.agentRefs = agentRefs;
}
@XmlElement
public List getAgentRefs() {
return this.agentRefs;
}
@XmlElement
public void setAppNodeRefs(final List appNodeRefs) {
this.appNodeRefs = appNodeRefs;
}
@XmlElement
public List getAppNodeRefs(final List appNodeRefs) {
return this.appNodeRefs;
}
/**
* @return the agents
*/
@XmlElement
public Collection getAgents() {
return this.agents;
}
/**
* @param agents
* the agents to set
*/
public void setAgents(final List agents) {
this.agents = agents;
}
/**
* @return the appNodes on this machine
*/
@XmlElement
public Collection getAppNodes() {
return this.appNodes;
}
/**
* @param appNodes
* the appNodes to set
*/
public void setAppNodes(final List appNodes) {
this.appNodes = appNodes;
}
/**
* @return the status
*/
@XmlElement
public MachineStatus getStatus() {
return this.status;
}
/**
* @param status
* the status to set
*/
public void setStatus(final MachineStatus status) {
if (status != null) {
this.status = status;
}
}
/**
* @return the OS patch level of this machine
*/
@XmlElement
public String getOSPatchLevel() {
return this.osPatchLevel;
}
/**
* @param osPatchLevel
* the osPatchLevel to set
*/
public void setOSPatchLevel(final String osPatchLevel) {
if (osPatchLevel != null) {
this.osPatchLevel = osPatchLevel;
}
}
@XmlElement
public String getOs() {
return this.os;
}
public void setOs(final String os) {
this.os = os;
}
@XmlElement
public String getOsPatchLevel() {
return this.osPatchLevel;
}
public void setOsPatchLevel(final String osPatchLevel) {
this.osPatchLevel = osPatchLevel;
}
@XmlElement
public List getAppNodeRefs() {
return this.appNodeRefs;
}
public static enum MachineStatus {
Unreachable, Running
};
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy