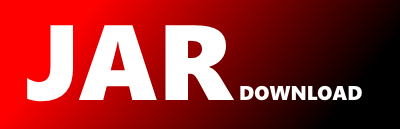
com.tibco.bw.maven.plugin.application.BWJsonMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bw6-maven-plugin Show documentation
Show all versions of bw6-maven-plugin Show documentation
Plugin Code for Apache Maven and TIBCO BusinessWorks™.
This is the Maven Plugin for BW6 and BWCE Build.
package com.tibco.bw.maven.plugin.application;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import org.apache.maven.model.Model;
import org.apache.maven.model.io.xpp3.MavenXpp3Reader;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.Component;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import org.codehaus.plexus.util.xml.pull.XmlPullParserException;
import org.yaml.snakeyaml.Yaml;
import org.yaml.snakeyaml.DumperOptions;
@Mojo(name = "bwfabric8json", defaultPhase = LifecyclePhase.INSTALL)
public class BWJsonMojo extends AbstractMojo{
@Parameter(defaultValue="${project}", readonly=true)
private MavenProject project;
@Parameter(defaultValue = "${project.projectBuildingRequest.activeProfileIds}", property = "profile", required = false)
private List profileIds;
private String getWorkspacepath() {
String workspacePath= System.getProperty("user.dir");
String wsPath= workspacePath;
if(wsPath.indexOf(".parent")!=-1){
wsPath= workspacePath.substring(0, workspacePath.lastIndexOf(".parent"));
}
return wsPath;
}
public static void mkDirs(File root, List dirs, int pos) {
if(dirs!=null){
if (pos == dirs.size()) return;
String s=dirs.get(pos);
File subdir = new File(root, s);
if(!subdir.exists())
subdir.mkdir();
mkDirs(subdir, dirs, pos+1);
}
}
private void copyFile(File source, File destin) throws IOException{
InputStream in = new FileInputStream(source);
OutputStream out = new FileOutputStream(destin);
byte[] buf = new byte[1024];
int len;
while ((len = in.read(buf)) > 0) {
out.write(buf, 0, len);
}
in.close();
out.close();
}
private Properties getK8sPropertiesFromFile() throws MojoExecutionException{
String file=(getWorkspacepath() + File.separator + "k8s-dev.properties");
String profile=null;
if(profileIds!=null && !profileIds.isEmpty()){
profile= profileIds.get(0);
}
if(profile!=null){
file= (getWorkspacepath() + File.separator + "k8s-"+profile+".properties");
}
Properties prop = new Properties();
InputStream input = null;
try {
input = new FileInputStream(file);
// load the Kubernetes properties file
prop.load(input);
}
catch(Exception e)
{
throw new MojoExecutionException("Could not load input from "+file+" due to exception: "+e);
}
return prop;
}
private Properties getDockerPropertiesFromFile() throws MojoExecutionException{
String fileDocker=(getWorkspacepath() + File.separator + "docker-dev.properties");
String profile=null;
if(profileIds!=null && !profileIds.isEmpty()){
profile=profileIds.get(0);
}
if(profile!=null){
fileDocker= (getWorkspacepath() + File.separator + "docker-"+profile+".properties");
}
Properties propsDocker = new Properties();
FileInputStream input = null;
try {
input = new FileInputStream(fileDocker);
// load the Docker properties file
propsDocker.load(input);
}
catch(Exception e)
{
throw new MojoExecutionException("Could not load input from "+fileDocker+" due to exception: "+e);
}
return propsDocker;
}
private void writeToYamlFile(String location,
Map data) throws MojoExecutionException {
//snakeyml for writing maps nested objects to yml file
DumperOptions options = new DumperOptions();
options.setDefaultFlowStyle(DumperOptions.FlowStyle.BLOCK);
Yaml yml = new Yaml(options);
FileWriter writer=null;
try {
writer = new FileWriter(location);
} catch (IOException e1) {
throw new MojoExecutionException("Could not write to service.yml due to exception: "+e1);
}
if(writer!=null)
yml.dump(data, writer);
}
private String getAppVersion() throws MojoExecutionException{
MavenXpp3Reader reader = new MavenXpp3Reader();
Model model=null;
String version=null;
try {
model = reader.read(new FileReader(getWorkspacepath()+".parent"+File.separator+"pom.xml"));
} catch (FileNotFoundException e1) {
throw new MojoExecutionException("File pom.xml not found for reading application version");
} catch (IOException e1) {
throw new MojoExecutionException("Exception while reading pom.xml : "+e1);
} catch (XmlPullParserException e1) {
throw new MojoExecutionException("Error while parsing POM file: "+e1);
}
if(model!=null){
version= model.getVersion();
}
return version;
}
private void createServiceYmlFile(Properties k8sprop) throws MojoExecutionException{
String locationService = getWorkspacepath() + File.separator + "src/main/fabric8/service.yml";
File serviceFile = new File(Paths.get(locationService).toString());
try {
serviceFile.createNewFile();
} catch (IOException e1) {
throw new MojoExecutionException("Could not create file service.yml due to exception: "+e1);
}
Map dataService = new HashMap();
dataService.put("kind", "Service");
Map metadataService=new HashMap();
metadataService.put("name", k8sprop.getProperty("fabric8.service.name"));
Map serviceLabels= new HashMap();
serviceLabels.put("container", k8sprop.getProperty("fabric8.container.name"));
serviceLabels.put("project", k8sprop.getProperty("fabric8.label.project"));
serviceLabels.put("provider","fabric8");
serviceLabels.put("group", "com.tibco.bw");
metadataService.put("labels",serviceLabels);
metadataService.put("namespace",k8sprop.getProperty("fabric8.namespace"));
dataService.put("metadata", metadataService);
Map specdataService=new HashMap();
specdataService.put("type", k8sprop.getProperty("fabric8.service.type"));
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy