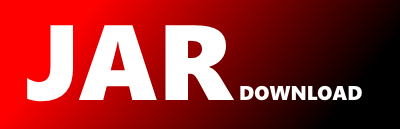
com.tigrisdata.db.api.v1.grpc.TigrisDBGrpc Maven / Gradle / Ivy
package com.tigrisdata.db.api.v1.grpc;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.45.0)",
comments = "Source: server/v1/api.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class TigrisDBGrpc {
private TigrisDBGrpc() {}
public static final String SERVICE_NAME = "TigrisDB";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getBeginTransactionMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "BeginTransaction",
requestType = com.tigrisdata.db.api.v1.grpc.Api.BeginTransactionRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.BeginTransactionResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getBeginTransactionMethod() {
io.grpc.MethodDescriptor getBeginTransactionMethod;
if ((getBeginTransactionMethod = TigrisDBGrpc.getBeginTransactionMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getBeginTransactionMethod = TigrisDBGrpc.getBeginTransactionMethod) == null) {
TigrisDBGrpc.getBeginTransactionMethod = getBeginTransactionMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "BeginTransaction"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.BeginTransactionRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.BeginTransactionResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("BeginTransaction"))
.build();
}
}
}
return getBeginTransactionMethod;
}
private static volatile io.grpc.MethodDescriptor getCommitTransactionMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CommitTransaction",
requestType = com.tigrisdata.db.api.v1.grpc.Api.CommitTransactionRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.CommitTransactionResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCommitTransactionMethod() {
io.grpc.MethodDescriptor getCommitTransactionMethod;
if ((getCommitTransactionMethod = TigrisDBGrpc.getCommitTransactionMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getCommitTransactionMethod = TigrisDBGrpc.getCommitTransactionMethod) == null) {
TigrisDBGrpc.getCommitTransactionMethod = getCommitTransactionMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "CommitTransaction"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.CommitTransactionRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.CommitTransactionResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("CommitTransaction"))
.build();
}
}
}
return getCommitTransactionMethod;
}
private static volatile io.grpc.MethodDescriptor getRollbackTransactionMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "RollbackTransaction",
requestType = com.tigrisdata.db.api.v1.grpc.Api.RollbackTransactionRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.RollbackTransactionResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRollbackTransactionMethod() {
io.grpc.MethodDescriptor getRollbackTransactionMethod;
if ((getRollbackTransactionMethod = TigrisDBGrpc.getRollbackTransactionMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getRollbackTransactionMethod = TigrisDBGrpc.getRollbackTransactionMethod) == null) {
TigrisDBGrpc.getRollbackTransactionMethod = getRollbackTransactionMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "RollbackTransaction"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.RollbackTransactionRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.RollbackTransactionResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("RollbackTransaction"))
.build();
}
}
}
return getRollbackTransactionMethod;
}
private static volatile io.grpc.MethodDescriptor getInsertMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Insert",
requestType = com.tigrisdata.db.api.v1.grpc.Api.InsertRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.InsertResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getInsertMethod() {
io.grpc.MethodDescriptor getInsertMethod;
if ((getInsertMethod = TigrisDBGrpc.getInsertMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getInsertMethod = TigrisDBGrpc.getInsertMethod) == null) {
TigrisDBGrpc.getInsertMethod = getInsertMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Insert"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.InsertRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.InsertResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("Insert"))
.build();
}
}
}
return getInsertMethod;
}
private static volatile io.grpc.MethodDescriptor getReplaceMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Replace",
requestType = com.tigrisdata.db.api.v1.grpc.Api.ReplaceRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.ReplaceResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getReplaceMethod() {
io.grpc.MethodDescriptor getReplaceMethod;
if ((getReplaceMethod = TigrisDBGrpc.getReplaceMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getReplaceMethod = TigrisDBGrpc.getReplaceMethod) == null) {
TigrisDBGrpc.getReplaceMethod = getReplaceMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Replace"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.ReplaceRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.ReplaceResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("Replace"))
.build();
}
}
}
return getReplaceMethod;
}
private static volatile io.grpc.MethodDescriptor getDeleteMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Delete",
requestType = com.tigrisdata.db.api.v1.grpc.Api.DeleteRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.DeleteResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDeleteMethod() {
io.grpc.MethodDescriptor getDeleteMethod;
if ((getDeleteMethod = TigrisDBGrpc.getDeleteMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getDeleteMethod = TigrisDBGrpc.getDeleteMethod) == null) {
TigrisDBGrpc.getDeleteMethod = getDeleteMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Delete"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.DeleteRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.DeleteResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("Delete"))
.build();
}
}
}
return getDeleteMethod;
}
private static volatile io.grpc.MethodDescriptor getUpdateMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Update",
requestType = com.tigrisdata.db.api.v1.grpc.Api.UpdateRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.UpdateResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getUpdateMethod() {
io.grpc.MethodDescriptor getUpdateMethod;
if ((getUpdateMethod = TigrisDBGrpc.getUpdateMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getUpdateMethod = TigrisDBGrpc.getUpdateMethod) == null) {
TigrisDBGrpc.getUpdateMethod = getUpdateMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Update"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.UpdateRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.UpdateResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("Update"))
.build();
}
}
}
return getUpdateMethod;
}
private static volatile io.grpc.MethodDescriptor getReadMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Read",
requestType = com.tigrisdata.db.api.v1.grpc.Api.ReadRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.ReadResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getReadMethod() {
io.grpc.MethodDescriptor getReadMethod;
if ((getReadMethod = TigrisDBGrpc.getReadMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getReadMethod = TigrisDBGrpc.getReadMethod) == null) {
TigrisDBGrpc.getReadMethod = getReadMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Read"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.ReadRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.ReadResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("Read"))
.build();
}
}
}
return getReadMethod;
}
private static volatile io.grpc.MethodDescriptor getCreateOrUpdateCollectionMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CreateOrUpdateCollection",
requestType = com.tigrisdata.db.api.v1.grpc.Api.CreateOrUpdateCollectionRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.CreateOrUpdateCollectionResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCreateOrUpdateCollectionMethod() {
io.grpc.MethodDescriptor getCreateOrUpdateCollectionMethod;
if ((getCreateOrUpdateCollectionMethod = TigrisDBGrpc.getCreateOrUpdateCollectionMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getCreateOrUpdateCollectionMethod = TigrisDBGrpc.getCreateOrUpdateCollectionMethod) == null) {
TigrisDBGrpc.getCreateOrUpdateCollectionMethod = getCreateOrUpdateCollectionMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "CreateOrUpdateCollection"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.CreateOrUpdateCollectionRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.CreateOrUpdateCollectionResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("CreateOrUpdateCollection"))
.build();
}
}
}
return getCreateOrUpdateCollectionMethod;
}
private static volatile io.grpc.MethodDescriptor getDropCollectionMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DropCollection",
requestType = com.tigrisdata.db.api.v1.grpc.Api.DropCollectionRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.DropCollectionResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDropCollectionMethod() {
io.grpc.MethodDescriptor getDropCollectionMethod;
if ((getDropCollectionMethod = TigrisDBGrpc.getDropCollectionMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getDropCollectionMethod = TigrisDBGrpc.getDropCollectionMethod) == null) {
TigrisDBGrpc.getDropCollectionMethod = getDropCollectionMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DropCollection"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.DropCollectionRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.DropCollectionResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("DropCollection"))
.build();
}
}
}
return getDropCollectionMethod;
}
private static volatile io.grpc.MethodDescriptor getListDatabasesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListDatabases",
requestType = com.tigrisdata.db.api.v1.grpc.Api.ListDatabasesRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.ListDatabasesResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getListDatabasesMethod() {
io.grpc.MethodDescriptor getListDatabasesMethod;
if ((getListDatabasesMethod = TigrisDBGrpc.getListDatabasesMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getListDatabasesMethod = TigrisDBGrpc.getListDatabasesMethod) == null) {
TigrisDBGrpc.getListDatabasesMethod = getListDatabasesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListDatabases"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.ListDatabasesRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.ListDatabasesResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("ListDatabases"))
.build();
}
}
}
return getListDatabasesMethod;
}
private static volatile io.grpc.MethodDescriptor getListCollectionsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListCollections",
requestType = com.tigrisdata.db.api.v1.grpc.Api.ListCollectionsRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.ListCollectionsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getListCollectionsMethod() {
io.grpc.MethodDescriptor getListCollectionsMethod;
if ((getListCollectionsMethod = TigrisDBGrpc.getListCollectionsMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getListCollectionsMethod = TigrisDBGrpc.getListCollectionsMethod) == null) {
TigrisDBGrpc.getListCollectionsMethod = getListCollectionsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListCollections"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.ListCollectionsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.ListCollectionsResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("ListCollections"))
.build();
}
}
}
return getListCollectionsMethod;
}
private static volatile io.grpc.MethodDescriptor getCreateDatabaseMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CreateDatabase",
requestType = com.tigrisdata.db.api.v1.grpc.Api.CreateDatabaseRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.CreateDatabaseResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCreateDatabaseMethod() {
io.grpc.MethodDescriptor getCreateDatabaseMethod;
if ((getCreateDatabaseMethod = TigrisDBGrpc.getCreateDatabaseMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getCreateDatabaseMethod = TigrisDBGrpc.getCreateDatabaseMethod) == null) {
TigrisDBGrpc.getCreateDatabaseMethod = getCreateDatabaseMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "CreateDatabase"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.CreateDatabaseRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.CreateDatabaseResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("CreateDatabase"))
.build();
}
}
}
return getCreateDatabaseMethod;
}
private static volatile io.grpc.MethodDescriptor getDropDatabaseMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DropDatabase",
requestType = com.tigrisdata.db.api.v1.grpc.Api.DropDatabaseRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.DropDatabaseResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDropDatabaseMethod() {
io.grpc.MethodDescriptor getDropDatabaseMethod;
if ((getDropDatabaseMethod = TigrisDBGrpc.getDropDatabaseMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getDropDatabaseMethod = TigrisDBGrpc.getDropDatabaseMethod) == null) {
TigrisDBGrpc.getDropDatabaseMethod = getDropDatabaseMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DropDatabase"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.DropDatabaseRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.DropDatabaseResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("DropDatabase"))
.build();
}
}
}
return getDropDatabaseMethod;
}
private static volatile io.grpc.MethodDescriptor getDescribeDatabaseMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DescribeDatabase",
requestType = com.tigrisdata.db.api.v1.grpc.Api.DescribeDatabaseRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.DescribeDatabaseResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDescribeDatabaseMethod() {
io.grpc.MethodDescriptor getDescribeDatabaseMethod;
if ((getDescribeDatabaseMethod = TigrisDBGrpc.getDescribeDatabaseMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getDescribeDatabaseMethod = TigrisDBGrpc.getDescribeDatabaseMethod) == null) {
TigrisDBGrpc.getDescribeDatabaseMethod = getDescribeDatabaseMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DescribeDatabase"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.DescribeDatabaseRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.DescribeDatabaseResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("DescribeDatabase"))
.build();
}
}
}
return getDescribeDatabaseMethod;
}
private static volatile io.grpc.MethodDescriptor getDescribeCollectionMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DescribeCollection",
requestType = com.tigrisdata.db.api.v1.grpc.Api.DescribeCollectionRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.DescribeCollectionResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDescribeCollectionMethod() {
io.grpc.MethodDescriptor getDescribeCollectionMethod;
if ((getDescribeCollectionMethod = TigrisDBGrpc.getDescribeCollectionMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getDescribeCollectionMethod = TigrisDBGrpc.getDescribeCollectionMethod) == null) {
TigrisDBGrpc.getDescribeCollectionMethod = getDescribeCollectionMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DescribeCollection"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.DescribeCollectionRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.DescribeCollectionResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("DescribeCollection"))
.build();
}
}
}
return getDescribeCollectionMethod;
}
private static volatile io.grpc.MethodDescriptor getStreamMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Stream",
requestType = com.tigrisdata.db.api.v1.grpc.Api.StreamRequest.class,
responseType = com.tigrisdata.db.api.v1.grpc.Api.StreamResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getStreamMethod() {
io.grpc.MethodDescriptor getStreamMethod;
if ((getStreamMethod = TigrisDBGrpc.getStreamMethod) == null) {
synchronized (TigrisDBGrpc.class) {
if ((getStreamMethod = TigrisDBGrpc.getStreamMethod) == null) {
TigrisDBGrpc.getStreamMethod = getStreamMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Stream"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.StreamRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.tigrisdata.db.api.v1.grpc.Api.StreamResponse.getDefaultInstance()))
.setSchemaDescriptor(new TigrisDBMethodDescriptorSupplier("Stream"))
.build();
}
}
}
return getStreamMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static TigrisDBStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public TigrisDBStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new TigrisDBStub(channel, callOptions);
}
};
return TigrisDBStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static TigrisDBBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public TigrisDBBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new TigrisDBBlockingStub(channel, callOptions);
}
};
return TigrisDBBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static TigrisDBFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public TigrisDBFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new TigrisDBFutureStub(channel, callOptions);
}
};
return TigrisDBFutureStub.newStub(factory, channel);
}
/**
*/
public static abstract class TigrisDBImplBase implements io.grpc.BindableService {
/**
*
* Starts a new transaction and returns a transactional object. All reads/writes performed
* within a transaction will run with serializable isolation.
*
*/
public void beginTransaction(com.tigrisdata.db.api.v1.grpc.Api.BeginTransactionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getBeginTransactionMethod(), responseObserver);
}
/**
*
* Atomically commit all the changes performed in the context of the transaction. Commit provides all
* or nothing semantics by ensuring no partial updates are in the database due to a transaction failure.
*
*/
public void commitTransaction(com.tigrisdata.db.api.v1.grpc.Api.CommitTransactionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCommitTransactionMethod(), responseObserver);
}
/**
*
* Rollback transaction discards all the changes
* performed in the transaction
*
*/
public void rollbackTransaction(com.tigrisdata.db.api.v1.grpc.Api.RollbackTransactionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getRollbackTransactionMethod(), responseObserver);
}
/**
*
* Inserts new documents in the collection and returns an AlreadyExists error if any of the documents
* in the request already exists. Insert provides idempotency by returning an error if the document
* already exists. To replace documents, use REPLACE API instead of INSERT.
*
*/
public void insert(com.tigrisdata.db.api.v1.grpc.Api.InsertRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getInsertMethod(), responseObserver);
}
/**
*
* Inserts the documents or replaces the existing documents in the collections.
*
*/
public void replace(com.tigrisdata.db.api.v1.grpc.Api.ReplaceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReplaceMethod(), responseObserver);
}
/**
*
* Delete a range of documents in the collection using the condition provided in the filter.
*
*/
public void delete(com.tigrisdata.db.api.v1.grpc.Api.DeleteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDeleteMethod(), responseObserver);
}
/**
*
* Update a range of documents in the collection using the condition provided in the filter.
*
*/
public void update(com.tigrisdata.db.api.v1.grpc.Api.UpdateRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getUpdateMethod(), responseObserver);
}
/**
*
* Reads range of documents from the collection using the condition in the filter.
*
*/
public void read(com.tigrisdata.db.api.v1.grpc.Api.ReadRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getReadMethod(), responseObserver);
}
/**
*
* Creates a new collection or atomically upgrades the collection to the new schema changes in the database
* passed in the request.
*
*/
public void createOrUpdateCollection(com.tigrisdata.db.api.v1.grpc.Api.CreateOrUpdateCollectionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCreateOrUpdateCollectionMethod(), responseObserver);
}
/**
*
* Drop the collection and all its documents in the database passed in the request.
*
*/
public void dropCollection(com.tigrisdata.db.api.v1.grpc.Api.DropCollectionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDropCollectionMethod(), responseObserver);
}
/**
*
* List returns all the databases.
*
*/
public void listDatabases(com.tigrisdata.db.api.v1.grpc.Api.ListDatabasesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListDatabasesMethod(), responseObserver);
}
/**
*
* List all collections in the database passed in the request.
*
*/
public void listCollections(com.tigrisdata.db.api.v1.grpc.Api.ListCollectionsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListCollectionsMethod(), responseObserver);
}
/**
*
* Creates a new database and returns a AlreadyExists error if the database already exists.
*
*/
public void createDatabase(com.tigrisdata.db.api.v1.grpc.Api.CreateDatabaseRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCreateDatabaseMethod(), responseObserver);
}
/**
*
* Drop database deletes all the collections in the database along with all it documents.
*
*/
public void dropDatabase(com.tigrisdata.db.api.v1.grpc.Api.DropDatabaseRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDropDatabaseMethod(), responseObserver);
}
/**
*
* Describe database describes the information related to database along
* with all the collections inside database.
*
*/
public void describeDatabase(com.tigrisdata.db.api.v1.grpc.Api.DescribeDatabaseRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDescribeDatabaseMethod(), responseObserver);
}
/**
*
* Describe collection describes the information related to collection.
*
*/
public void describeCollection(com.tigrisdata.db.api.v1.grpc.Api.DescribeCollectionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDescribeCollectionMethod(), responseObserver);
}
/**
*/
public void stream(com.tigrisdata.db.api.v1.grpc.Api.StreamRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getStreamMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getBeginTransactionMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.BeginTransactionRequest,
com.tigrisdata.db.api.v1.grpc.Api.BeginTransactionResponse>(
this, METHODID_BEGIN_TRANSACTION)))
.addMethod(
getCommitTransactionMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.CommitTransactionRequest,
com.tigrisdata.db.api.v1.grpc.Api.CommitTransactionResponse>(
this, METHODID_COMMIT_TRANSACTION)))
.addMethod(
getRollbackTransactionMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.RollbackTransactionRequest,
com.tigrisdata.db.api.v1.grpc.Api.RollbackTransactionResponse>(
this, METHODID_ROLLBACK_TRANSACTION)))
.addMethod(
getInsertMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.InsertRequest,
com.tigrisdata.db.api.v1.grpc.Api.InsertResponse>(
this, METHODID_INSERT)))
.addMethod(
getReplaceMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.ReplaceRequest,
com.tigrisdata.db.api.v1.grpc.Api.ReplaceResponse>(
this, METHODID_REPLACE)))
.addMethod(
getDeleteMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.DeleteRequest,
com.tigrisdata.db.api.v1.grpc.Api.DeleteResponse>(
this, METHODID_DELETE)))
.addMethod(
getUpdateMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.UpdateRequest,
com.tigrisdata.db.api.v1.grpc.Api.UpdateResponse>(
this, METHODID_UPDATE)))
.addMethod(
getReadMethod(),
io.grpc.stub.ServerCalls.asyncServerStreamingCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.ReadRequest,
com.tigrisdata.db.api.v1.grpc.Api.ReadResponse>(
this, METHODID_READ)))
.addMethod(
getCreateOrUpdateCollectionMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.CreateOrUpdateCollectionRequest,
com.tigrisdata.db.api.v1.grpc.Api.CreateOrUpdateCollectionResponse>(
this, METHODID_CREATE_OR_UPDATE_COLLECTION)))
.addMethod(
getDropCollectionMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.DropCollectionRequest,
com.tigrisdata.db.api.v1.grpc.Api.DropCollectionResponse>(
this, METHODID_DROP_COLLECTION)))
.addMethod(
getListDatabasesMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.ListDatabasesRequest,
com.tigrisdata.db.api.v1.grpc.Api.ListDatabasesResponse>(
this, METHODID_LIST_DATABASES)))
.addMethod(
getListCollectionsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.ListCollectionsRequest,
com.tigrisdata.db.api.v1.grpc.Api.ListCollectionsResponse>(
this, METHODID_LIST_COLLECTIONS)))
.addMethod(
getCreateDatabaseMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.CreateDatabaseRequest,
com.tigrisdata.db.api.v1.grpc.Api.CreateDatabaseResponse>(
this, METHODID_CREATE_DATABASE)))
.addMethod(
getDropDatabaseMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.DropDatabaseRequest,
com.tigrisdata.db.api.v1.grpc.Api.DropDatabaseResponse>(
this, METHODID_DROP_DATABASE)))
.addMethod(
getDescribeDatabaseMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.DescribeDatabaseRequest,
com.tigrisdata.db.api.v1.grpc.Api.DescribeDatabaseResponse>(
this, METHODID_DESCRIBE_DATABASE)))
.addMethod(
getDescribeCollectionMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.DescribeCollectionRequest,
com.tigrisdata.db.api.v1.grpc.Api.DescribeCollectionResponse>(
this, METHODID_DESCRIBE_COLLECTION)))
.addMethod(
getStreamMethod(),
io.grpc.stub.ServerCalls.asyncServerStreamingCall(
new MethodHandlers<
com.tigrisdata.db.api.v1.grpc.Api.StreamRequest,
com.tigrisdata.db.api.v1.grpc.Api.StreamResponse>(
this, METHODID_STREAM)))
.build();
}
}
/**
*/
public static final class TigrisDBStub extends io.grpc.stub.AbstractAsyncStub {
private TigrisDBStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected TigrisDBStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new TigrisDBStub(channel, callOptions);
}
/**
*
* Starts a new transaction and returns a transactional object. All reads/writes performed
* within a transaction will run with serializable isolation.
*
*/
public void beginTransaction(com.tigrisdata.db.api.v1.grpc.Api.BeginTransactionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getBeginTransactionMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Atomically commit all the changes performed in the context of the transaction. Commit provides all
* or nothing semantics by ensuring no partial updates are in the database due to a transaction failure.
*
*/
public void commitTransaction(com.tigrisdata.db.api.v1.grpc.Api.CommitTransactionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCommitTransactionMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Rollback transaction discards all the changes
* performed in the transaction
*
*/
public void rollbackTransaction(com.tigrisdata.db.api.v1.grpc.Api.RollbackTransactionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getRollbackTransactionMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Inserts new documents in the collection and returns an AlreadyExists error if any of the documents
* in the request already exists. Insert provides idempotency by returning an error if the document
* already exists. To replace documents, use REPLACE API instead of INSERT.
*
*/
public void insert(com.tigrisdata.db.api.v1.grpc.Api.InsertRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getInsertMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Inserts the documents or replaces the existing documents in the collections.
*
*/
public void replace(com.tigrisdata.db.api.v1.grpc.Api.ReplaceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getReplaceMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Delete a range of documents in the collection using the condition provided in the filter.
*
*/
public void delete(com.tigrisdata.db.api.v1.grpc.Api.DeleteRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDeleteMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Update a range of documents in the collection using the condition provided in the filter.
*
*/
public void update(com.tigrisdata.db.api.v1.grpc.Api.UpdateRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getUpdateMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Reads range of documents from the collection using the condition in the filter.
*
*/
public void read(com.tigrisdata.db.api.v1.grpc.Api.ReadRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getReadMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Creates a new collection or atomically upgrades the collection to the new schema changes in the database
* passed in the request.
*
*/
public void createOrUpdateCollection(com.tigrisdata.db.api.v1.grpc.Api.CreateOrUpdateCollectionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCreateOrUpdateCollectionMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Drop the collection and all its documents in the database passed in the request.
*
*/
public void dropCollection(com.tigrisdata.db.api.v1.grpc.Api.DropCollectionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDropCollectionMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* List returns all the databases.
*
*/
public void listDatabases(com.tigrisdata.db.api.v1.grpc.Api.ListDatabasesRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getListDatabasesMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* List all collections in the database passed in the request.
*
*/
public void listCollections(com.tigrisdata.db.api.v1.grpc.Api.ListCollectionsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getListCollectionsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Creates a new database and returns a AlreadyExists error if the database already exists.
*
*/
public void createDatabase(com.tigrisdata.db.api.v1.grpc.Api.CreateDatabaseRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCreateDatabaseMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Drop database deletes all the collections in the database along with all it documents.
*
*/
public void dropDatabase(com.tigrisdata.db.api.v1.grpc.Api.DropDatabaseRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDropDatabaseMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Describe database describes the information related to database along
* with all the collections inside database.
*
*/
public void describeDatabase(com.tigrisdata.db.api.v1.grpc.Api.DescribeDatabaseRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDescribeDatabaseMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Describe collection describes the information related to collection.
*
*/
public void describeCollection(com.tigrisdata.db.api.v1.grpc.Api.DescribeCollectionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDescribeCollectionMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void stream(com.tigrisdata.db.api.v1.grpc.Api.StreamRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getStreamMethod(), getCallOptions()), request, responseObserver);
}
}
/**
*/
public static final class TigrisDBBlockingStub extends io.grpc.stub.AbstractBlockingStub {
private TigrisDBBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected TigrisDBBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new TigrisDBBlockingStub(channel, callOptions);
}
/**
*
* Starts a new transaction and returns a transactional object. All reads/writes performed
* within a transaction will run with serializable isolation.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.BeginTransactionResponse beginTransaction(com.tigrisdata.db.api.v1.grpc.Api.BeginTransactionRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getBeginTransactionMethod(), getCallOptions(), request);
}
/**
*
* Atomically commit all the changes performed in the context of the transaction. Commit provides all
* or nothing semantics by ensuring no partial updates are in the database due to a transaction failure.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.CommitTransactionResponse commitTransaction(com.tigrisdata.db.api.v1.grpc.Api.CommitTransactionRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCommitTransactionMethod(), getCallOptions(), request);
}
/**
*
* Rollback transaction discards all the changes
* performed in the transaction
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.RollbackTransactionResponse rollbackTransaction(com.tigrisdata.db.api.v1.grpc.Api.RollbackTransactionRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getRollbackTransactionMethod(), getCallOptions(), request);
}
/**
*
* Inserts new documents in the collection and returns an AlreadyExists error if any of the documents
* in the request already exists. Insert provides idempotency by returning an error if the document
* already exists. To replace documents, use REPLACE API instead of INSERT.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.InsertResponse insert(com.tigrisdata.db.api.v1.grpc.Api.InsertRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getInsertMethod(), getCallOptions(), request);
}
/**
*
* Inserts the documents or replaces the existing documents in the collections.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.ReplaceResponse replace(com.tigrisdata.db.api.v1.grpc.Api.ReplaceRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getReplaceMethod(), getCallOptions(), request);
}
/**
*
* Delete a range of documents in the collection using the condition provided in the filter.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.DeleteResponse delete(com.tigrisdata.db.api.v1.grpc.Api.DeleteRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDeleteMethod(), getCallOptions(), request);
}
/**
*
* Update a range of documents in the collection using the condition provided in the filter.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.UpdateResponse update(com.tigrisdata.db.api.v1.grpc.Api.UpdateRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getUpdateMethod(), getCallOptions(), request);
}
/**
*
* Reads range of documents from the collection using the condition in the filter.
*
*/
public java.util.Iterator read(
com.tigrisdata.db.api.v1.grpc.Api.ReadRequest request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getReadMethod(), getCallOptions(), request);
}
/**
*
* Creates a new collection or atomically upgrades the collection to the new schema changes in the database
* passed in the request.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.CreateOrUpdateCollectionResponse createOrUpdateCollection(com.tigrisdata.db.api.v1.grpc.Api.CreateOrUpdateCollectionRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCreateOrUpdateCollectionMethod(), getCallOptions(), request);
}
/**
*
* Drop the collection and all its documents in the database passed in the request.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.DropCollectionResponse dropCollection(com.tigrisdata.db.api.v1.grpc.Api.DropCollectionRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDropCollectionMethod(), getCallOptions(), request);
}
/**
*
* List returns all the databases.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.ListDatabasesResponse listDatabases(com.tigrisdata.db.api.v1.grpc.Api.ListDatabasesRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getListDatabasesMethod(), getCallOptions(), request);
}
/**
*
* List all collections in the database passed in the request.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.ListCollectionsResponse listCollections(com.tigrisdata.db.api.v1.grpc.Api.ListCollectionsRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getListCollectionsMethod(), getCallOptions(), request);
}
/**
*
* Creates a new database and returns a AlreadyExists error if the database already exists.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.CreateDatabaseResponse createDatabase(com.tigrisdata.db.api.v1.grpc.Api.CreateDatabaseRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCreateDatabaseMethod(), getCallOptions(), request);
}
/**
*
* Drop database deletes all the collections in the database along with all it documents.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.DropDatabaseResponse dropDatabase(com.tigrisdata.db.api.v1.grpc.Api.DropDatabaseRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDropDatabaseMethod(), getCallOptions(), request);
}
/**
*
* Describe database describes the information related to database along
* with all the collections inside database.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.DescribeDatabaseResponse describeDatabase(com.tigrisdata.db.api.v1.grpc.Api.DescribeDatabaseRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDescribeDatabaseMethod(), getCallOptions(), request);
}
/**
*
* Describe collection describes the information related to collection.
*
*/
public com.tigrisdata.db.api.v1.grpc.Api.DescribeCollectionResponse describeCollection(com.tigrisdata.db.api.v1.grpc.Api.DescribeCollectionRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDescribeCollectionMethod(), getCallOptions(), request);
}
/**
*/
public java.util.Iterator stream(
com.tigrisdata.db.api.v1.grpc.Api.StreamRequest request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getStreamMethod(), getCallOptions(), request);
}
}
/**
*/
public static final class TigrisDBFutureStub extends io.grpc.stub.AbstractFutureStub {
private TigrisDBFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected TigrisDBFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new TigrisDBFutureStub(channel, callOptions);
}
/**
*
* Starts a new transaction and returns a transactional object. All reads/writes performed
* within a transaction will run with serializable isolation.
*
*/
public com.google.common.util.concurrent.ListenableFuture beginTransaction(
com.tigrisdata.db.api.v1.grpc.Api.BeginTransactionRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getBeginTransactionMethod(), getCallOptions()), request);
}
/**
*
* Atomically commit all the changes performed in the context of the transaction. Commit provides all
* or nothing semantics by ensuring no partial updates are in the database due to a transaction failure.
*
*/
public com.google.common.util.concurrent.ListenableFuture commitTransaction(
com.tigrisdata.db.api.v1.grpc.Api.CommitTransactionRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCommitTransactionMethod(), getCallOptions()), request);
}
/**
*
* Rollback transaction discards all the changes
* performed in the transaction
*
*/
public com.google.common.util.concurrent.ListenableFuture rollbackTransaction(
com.tigrisdata.db.api.v1.grpc.Api.RollbackTransactionRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getRollbackTransactionMethod(), getCallOptions()), request);
}
/**
*
* Inserts new documents in the collection and returns an AlreadyExists error if any of the documents
* in the request already exists. Insert provides idempotency by returning an error if the document
* already exists. To replace documents, use REPLACE API instead of INSERT.
*
*/
public com.google.common.util.concurrent.ListenableFuture insert(
com.tigrisdata.db.api.v1.grpc.Api.InsertRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getInsertMethod(), getCallOptions()), request);
}
/**
*
* Inserts the documents or replaces the existing documents in the collections.
*
*/
public com.google.common.util.concurrent.ListenableFuture replace(
com.tigrisdata.db.api.v1.grpc.Api.ReplaceRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getReplaceMethod(), getCallOptions()), request);
}
/**
*
* Delete a range of documents in the collection using the condition provided in the filter.
*
*/
public com.google.common.util.concurrent.ListenableFuture delete(
com.tigrisdata.db.api.v1.grpc.Api.DeleteRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDeleteMethod(), getCallOptions()), request);
}
/**
*
* Update a range of documents in the collection using the condition provided in the filter.
*
*/
public com.google.common.util.concurrent.ListenableFuture update(
com.tigrisdata.db.api.v1.grpc.Api.UpdateRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getUpdateMethod(), getCallOptions()), request);
}
/**
*
* Creates a new collection or atomically upgrades the collection to the new schema changes in the database
* passed in the request.
*
*/
public com.google.common.util.concurrent.ListenableFuture createOrUpdateCollection(
com.tigrisdata.db.api.v1.grpc.Api.CreateOrUpdateCollectionRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCreateOrUpdateCollectionMethod(), getCallOptions()), request);
}
/**
*
* Drop the collection and all its documents in the database passed in the request.
*
*/
public com.google.common.util.concurrent.ListenableFuture dropCollection(
com.tigrisdata.db.api.v1.grpc.Api.DropCollectionRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDropCollectionMethod(), getCallOptions()), request);
}
/**
*
* List returns all the databases.
*
*/
public com.google.common.util.concurrent.ListenableFuture listDatabases(
com.tigrisdata.db.api.v1.grpc.Api.ListDatabasesRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getListDatabasesMethod(), getCallOptions()), request);
}
/**
*
* List all collections in the database passed in the request.
*
*/
public com.google.common.util.concurrent.ListenableFuture listCollections(
com.tigrisdata.db.api.v1.grpc.Api.ListCollectionsRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getListCollectionsMethod(), getCallOptions()), request);
}
/**
*
* Creates a new database and returns a AlreadyExists error if the database already exists.
*
*/
public com.google.common.util.concurrent.ListenableFuture createDatabase(
com.tigrisdata.db.api.v1.grpc.Api.CreateDatabaseRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCreateDatabaseMethod(), getCallOptions()), request);
}
/**
*
* Drop database deletes all the collections in the database along with all it documents.
*
*/
public com.google.common.util.concurrent.ListenableFuture dropDatabase(
com.tigrisdata.db.api.v1.grpc.Api.DropDatabaseRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDropDatabaseMethod(), getCallOptions()), request);
}
/**
*
* Describe database describes the information related to database along
* with all the collections inside database.
*
*/
public com.google.common.util.concurrent.ListenableFuture describeDatabase(
com.tigrisdata.db.api.v1.grpc.Api.DescribeDatabaseRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDescribeDatabaseMethod(), getCallOptions()), request);
}
/**
*
* Describe collection describes the information related to collection.
*
*/
public com.google.common.util.concurrent.ListenableFuture describeCollection(
com.tigrisdata.db.api.v1.grpc.Api.DescribeCollectionRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDescribeCollectionMethod(), getCallOptions()), request);
}
}
private static final int METHODID_BEGIN_TRANSACTION = 0;
private static final int METHODID_COMMIT_TRANSACTION = 1;
private static final int METHODID_ROLLBACK_TRANSACTION = 2;
private static final int METHODID_INSERT = 3;
private static final int METHODID_REPLACE = 4;
private static final int METHODID_DELETE = 5;
private static final int METHODID_UPDATE = 6;
private static final int METHODID_READ = 7;
private static final int METHODID_CREATE_OR_UPDATE_COLLECTION = 8;
private static final int METHODID_DROP_COLLECTION = 9;
private static final int METHODID_LIST_DATABASES = 10;
private static final int METHODID_LIST_COLLECTIONS = 11;
private static final int METHODID_CREATE_DATABASE = 12;
private static final int METHODID_DROP_DATABASE = 13;
private static final int METHODID_DESCRIBE_DATABASE = 14;
private static final int METHODID_DESCRIBE_COLLECTION = 15;
private static final int METHODID_STREAM = 16;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final TigrisDBImplBase serviceImpl;
private final int methodId;
MethodHandlers(TigrisDBImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_BEGIN_TRANSACTION:
serviceImpl.beginTransaction((com.tigrisdata.db.api.v1.grpc.Api.BeginTransactionRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_COMMIT_TRANSACTION:
serviceImpl.commitTransaction((com.tigrisdata.db.api.v1.grpc.Api.CommitTransactionRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_ROLLBACK_TRANSACTION:
serviceImpl.rollbackTransaction((com.tigrisdata.db.api.v1.grpc.Api.RollbackTransactionRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_INSERT:
serviceImpl.insert((com.tigrisdata.db.api.v1.grpc.Api.InsertRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_REPLACE:
serviceImpl.replace((com.tigrisdata.db.api.v1.grpc.Api.ReplaceRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DELETE:
serviceImpl.delete((com.tigrisdata.db.api.v1.grpc.Api.DeleteRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_UPDATE:
serviceImpl.update((com.tigrisdata.db.api.v1.grpc.Api.UpdateRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_READ:
serviceImpl.read((com.tigrisdata.db.api.v1.grpc.Api.ReadRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CREATE_OR_UPDATE_COLLECTION:
serviceImpl.createOrUpdateCollection((com.tigrisdata.db.api.v1.grpc.Api.CreateOrUpdateCollectionRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DROP_COLLECTION:
serviceImpl.dropCollection((com.tigrisdata.db.api.v1.grpc.Api.DropCollectionRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LIST_DATABASES:
serviceImpl.listDatabases((com.tigrisdata.db.api.v1.grpc.Api.ListDatabasesRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LIST_COLLECTIONS:
serviceImpl.listCollections((com.tigrisdata.db.api.v1.grpc.Api.ListCollectionsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CREATE_DATABASE:
serviceImpl.createDatabase((com.tigrisdata.db.api.v1.grpc.Api.CreateDatabaseRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DROP_DATABASE:
serviceImpl.dropDatabase((com.tigrisdata.db.api.v1.grpc.Api.DropDatabaseRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DESCRIBE_DATABASE:
serviceImpl.describeDatabase((com.tigrisdata.db.api.v1.grpc.Api.DescribeDatabaseRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DESCRIBE_COLLECTION:
serviceImpl.describeCollection((com.tigrisdata.db.api.v1.grpc.Api.DescribeCollectionRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_STREAM:
serviceImpl.stream((com.tigrisdata.db.api.v1.grpc.Api.StreamRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static abstract class TigrisDBBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
TigrisDBBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.tigrisdata.db.api.v1.grpc.Api.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("TigrisDB");
}
}
private static final class TigrisDBFileDescriptorSupplier
extends TigrisDBBaseDescriptorSupplier {
TigrisDBFileDescriptorSupplier() {}
}
private static final class TigrisDBMethodDescriptorSupplier
extends TigrisDBBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
TigrisDBMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (TigrisDBGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new TigrisDBFileDescriptorSupplier())
.addMethod(getBeginTransactionMethod())
.addMethod(getCommitTransactionMethod())
.addMethod(getRollbackTransactionMethod())
.addMethod(getInsertMethod())
.addMethod(getReplaceMethod())
.addMethod(getDeleteMethod())
.addMethod(getUpdateMethod())
.addMethod(getReadMethod())
.addMethod(getCreateOrUpdateCollectionMethod())
.addMethod(getDropCollectionMethod())
.addMethod(getListDatabasesMethod())
.addMethod(getListCollectionsMethod())
.addMethod(getCreateDatabaseMethod())
.addMethod(getDropDatabaseMethod())
.addMethod(getDescribeDatabaseMethod())
.addMethod(getDescribeCollectionMethod())
.addMethod(getStreamMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy