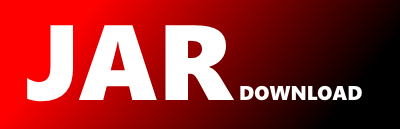
com.tigrisdata.db.client.TigrisAsyncCollection Maven / Gradle / Ivy
/*
* Copyright 2022 Tigris Data, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the
* License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.tigrisdata.db.client;
import com.tigrisdata.db.client.error.TigrisException;
import com.tigrisdata.db.type.TigrisCollectionType;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
/**
* An async TigrisDB collection
*
* @param type of collection
*/
public interface TigrisAsyncCollection {
/**
* @param filter filter to narrow down read
* @param fields optionally specify fields you want to be returned from server
* @param readRequestOptions read options
* @param reader reader callback
*/
void read(
TigrisFilter filter,
ReadFields fields,
ReadRequestOptions readRequestOptions,
TigrisAsyncReader reader);
/**
* Reads matching documents
*
* @param filter filter to narrow down read
* @param fields optionally specify fields you want to be returned from server
* @param reader reader callback
*/
void read(TigrisFilter filter, ReadFields fields, TigrisAsyncReader reader);
/**
* Reads a single document. This method is generally recommended for point lookup, if used for
* non-point lookup any arbitrary matching document will be returned.
*
* @param filter filter to read one document
* @return a future to the document
*/
CompletableFuture> readOne(TigrisFilter filter);
/**
* @param documents list of documents to insert
* @param insertRequestOptions insert option
* @return a future to the {@link InsertResponse}
* @throws TigrisException in case of an error
*/
CompletableFuture insert(
List documents, InsertRequestOptions insertRequestOptions) throws TigrisException;
/**
* @param documents list of documents to insert
* @return a future to the {@link InsertResponse}
* @throws TigrisException in case of an error
*/
CompletableFuture insert(List documents) throws TigrisException;
/**
* inserts a single document to the collection
*
* @param document document to insert
* @return a future to the {@link InsertResponse}
* @throws TigrisException in case of an error
*/
CompletableFuture insert(T document) throws TigrisException;
/**
* Inserts the documents if they don't exist already, replaces them otherwise.
*
* @param documents list of documents to replace
* @param insertOrReplaceRequestOptions option
* @return a future to the {@link InsertOrReplaceResponse}
* @throws TigrisException in case of an error
*/
CompletableFuture insertOrReplace(
List documents, InsertOrReplaceRequestOptions insertOrReplaceRequestOptions)
throws TigrisException;
/**
* Inserts the documents if they don't exist already, replaces them otherwise.
*
* @param documents list of documents to replace
* @return a future to the {@link InsertOrReplaceResponse}
* @throws TigrisException in case of an error
*/
CompletableFuture insertOrReplace(List documents)
throws TigrisException;
/**
* @param filter filters documents to update
* @param fields specifies what and how to update the fields from filtered documents
* @param updateRequestOptions options
* @return a future to the {@link UpdateResponse}
* @throws TigrisException in case of an error
*/
CompletableFuture update(
TigrisFilter filter, UpdateFields fields, UpdateRequestOptions updateRequestOptions)
throws TigrisException;
/**
* @param filter filters documents to update
* @param fields specifies what and how to update the fields from filtered documents
* @return a future to the {@link UpdateResponse}
* @throws TigrisException in case of an error
*/
CompletableFuture update(TigrisFilter filter, UpdateFields fields)
throws TigrisException;
/**
* Deletes the matching documents in the collection.
*
* @param filter filter to narrow down the documents to delete
* @param deleteRequestOptions delete option
* @return a future to the {@link DeleteResponse}
*/
CompletableFuture delete(
TigrisFilter filter, DeleteRequestOptions deleteRequestOptions);
/**
* Deletes the matching documents in the collection.
*
* @param filter filter to narrow down the documents to delete
* @return a future to the {@link DeleteResponse}
*/
CompletableFuture delete(TigrisFilter filter);
/**
* Describes the collection
*
* @param collectionOptions options
* @return future to the collection description
* @throws TigrisException in case of an error
*/
CompletableFuture describe(CollectionOptions collectionOptions)
throws TigrisException;
/** @return Name of the collection */
String name();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy