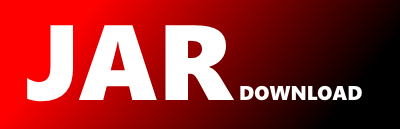
server.v1.api.proto Maven / Gradle / Ivy
// Copyright 2022 Tigris Data, Inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
syntax = "proto3";
import "google/api/annotations.proto";
import "google/protobuf/timestamp.proto";
option go_package = "github.com/tigrisdata/tigrisdb/api";
option java_package = "com.tigrisdata.db.api.v1.grpc";
// BUG: gnostic protoc-gen-openapi doesn't support enum generation
// https://github.com/google/gnostic/issues/255
message ErrorDetails {
uint32 code = 1;
string msg = 2;
}
// Additional options to modify write requests.
message WriteOptions {
// Perform operation in the context of this transaction.
TransactionCtx tx_ctx = 1;
}
// Options that can be used to modify the results, for example "limit" to control the number of documents
// returned by the server.
message ReadRequestOptions {
// Perform operation in the context of this transaction.
TransactionCtx tx_ctx = 1;
// Limit the number of documents returned by the read operation.
int64 limit = 2;
// Number of documents to skip before starting to return resulting documents.
int64 skip = 3;
// A cursor for use in pagination. The next streams will return documents after this offset.
bytes offset = 4;
}
// Database requests modifying options.
message DatabaseOptions {}
// Collection requests modifying options.
message CollectionOptions {
// Perform operation in the context of this transaction.
TransactionCtx tx_ctx = 1;
}
// Options that can be used to modify the transaction semantics.
message TransactionOptions {}
// Contains ID which uniquely identifies transaction
// This context is returned by BeginTransaction request and
// should be passed in the subsequent requests in order to run
// them in the context of the same transaction.
message TransactionCtx {
// Unique for a single transactional request.
string id = 1;
// Serves as an internal identifier.
string origin = 2;
}
// Start new transaction in database specified by "db".
message BeginTransactionRequest {
// Database name this transaction belongs to.
string db = 1;
// The transaction options.
TransactionOptions options = 2;
}
// Start transaction returns transaction context
// which uniquely identifies the transaction
message BeginTransactionResponse {
// Returns a tigris transactional context with details about the transactions.
TransactionCtx tx_ctx = 1;
}
// Commit transaction with the given ID
message CommitTransactionRequest {
// Database name this transaction belongs to.
string db = 1;
// Tigris transactional context with details about the transactions.
TransactionCtx tx_ctx = 2;
}
message CommitTransactionResponse {
// Status of commit transaction operation.
string status = 1;
}
// Rollback transaction with the given ID
message RollbackTransactionRequest {
// Database name this transaction belongs to.
string db = 1;
// Tigris transactional context with details about the transactions.
TransactionCtx tx_ctx = 2;
}
message RollbackTransactionResponse {
// Status of rollback transaction operation.
string status = 1;
}
// additional options for insert requests.
message InsertRequestOptions {
WriteOptions write_options = 1;
}
// Has metadata related to the documents stored.
message ResponseMetadata {
// Time at which the document was inserted/replaced. Measured in nano-seconds since the Unix epoch.
google.protobuf.Timestamp created_at = 1;
// Time at which the document was updated. Measured in nano-seconds since the Unix epoch.
google.protobuf.Timestamp updated_at = 2;
}
message InsertRequest {
// Database name where to insert documents.
string db = 1;
// Collection name where to insert documents.
string collection = 2;
// Array of documents to insert. Each document is a JSON object.
repeated bytes documents = 3;
InsertRequestOptions options = 4;
}
message InsertResponse {
// Has metadata related to the documents stored.
ResponseMetadata metadata = 1;
// An enum with value set as "inserted"
string status = 2;
}
// Additional options for replace requests.
message ReplaceRequestOptions {
WriteOptions write_options = 1;
}
message ReplaceRequest {
// Database name where to replace documents.
string db = 1;
// Collection name where to replace documents.
string collection = 2;
// Array of documents to be replaced. Each document is a JSON object.
repeated bytes documents = 3;
ReplaceRequestOptions options = 4;
}
message ReplaceResponse {
// Has metadata related to the documents stored.
ResponseMetadata metadata = 1;
// an enum with value set as "replaced"
string status = 2;
}
// Additional options for deleted requests.
message DeleteRequestOptions {
WriteOptions write_options = 1;
}
message DeleteRequest {
// Database name where to insert documents.
string db = 1;
// Collection name where to insert documents.
string collection = 2;
// Delete documents which matching specified filter.
// A filter can simply be key, value where key
// is the field name and value would be the value for this field. Or a filter can be logical where
// two or more fields can be logically joined using $or and $and. A few examples of filter:
// To delete a user document where the id has a value 1: ```{"id": 1 }```
// To delete all the user documents where the key "id" has a value 1 or 2 or 3: `{"$or": [{"id": 1}, {"id": 2}, {"id": 3}]}`
bytes filter = 3;
DeleteRequestOptions options = 4;
}
message DeleteResponse {
// Has metadata related to the documents stored.
ResponseMetadata metadata = 1;
// an enum with value set as "deleted"
string status = 2;
}
// Additional options for update requests.
message UpdateRequestOptions {
WriteOptions write_options = 1;
}
message UpdateRequest {
// Database name where to update documents
string db = 1;
// Collection name where to update documents
string collection = 2;
// Fields contains set of fields
// with the values which need to be updated.
// Should be proper JSON object.
bytes fields = 3;
// Update documents which matching specified filter.
// A filter can simply be key, value where key
// is the field name and value would be the value for this field. Or a filter can be logical where
// two or more fields can be logically joined using $or and $and. A few examples of filter:
// To update a user document where the id has a value 1: ```{"id": 1 }```
// To update all the user documents where the key "id" has a value 1 or 2 or 3: `{"$or": [{"id": 1}, {"id": 2}, {"id": 3}]}`
bytes filter = 4;
UpdateRequestOptions options = 5;
}
message UpdateResponse {
// Has metadata related to the documents stored.
ResponseMetadata metadata = 1;
// Returns the number of documents modified.
int32 modified_count = 2;
// an enum with value set as "updated".
string status = 3;
}
message ReadRequest {
// Database name to read documents from.
string db = 1;
// Collection name to read documents from.
string collection = 2;
// Returns documents matching this filter.
// A filter can simply be key, value where key
// is the field name and value would be the value for this field. Or a filter can be logical where
// two or more fields can be logically joined using $or and $and. A few examples of filter:
// To read a user document where the id has a value 1: ```{"id": 1 }```
// To read all the user documents where the key "id" has a value 1 or 2 or 3: `{"$or": [{"id": 1}, {"id": 2}, {"id": 3}]}`
bytes filter = 3;
// To read specific fields from a document. Default is all.
bytes fields = 4;
// Options that can be used to modify the results, for example "limit" to control the number of documents
// returned by the server.
ReadRequestOptions options = 5;
}
message ReadResponse {
// Object containing the collection document.
bytes data = 1;
// An internal key, used for pagination.
bytes resume_token = 2;
// Has metadata related to the documents stored.
ResponseMetadata metadata = 3;
}
message CreateDatabaseRequest {
// Create database with this name.
string db = 1;
DatabaseOptions options = 2;
}
message CreateDatabaseResponse {
// A detailed response message.
string message = 1;
// An enum with value set as "created".
string status = 2;
}
message DropDatabaseRequest {
// Drop database with this name. **Note**: Deletes all the collections in the database.
// Use with caution.
string db = 1;
DatabaseOptions options = 2;
}
message DropDatabaseResponse {
// A detailed response message.
string message = 1;
// An enum with value set as "dropped".
string status = 2;
}
message CreateOrUpdateCollectionRequest {
// Database name where to create collection.
string db = 1;
// Collection name to create.
string collection = 2;
// Schema of the documents in this collection. The schema specifications are same as
// JSON schema specification defined here.
// The following is an schema example:
// `{
// "name": "user",
// "description": "Collection of documents with details of users",
// "properties": {
// "id": {
// "description": "A unique identifier for the user",
// "type": "integer"
// },
// "name": {
// "description": "Name of the user",
// "type": "string",
// "maxLength": 100
// },
// "balance": {
// "description": "User account balance",
// "type": "number"
// }
// },
// "primary_key": ["id"]
// }`
bytes schema = 3;
bool only_create = 4;
CollectionOptions options = 5;
}
message CreateOrUpdateCollectionResponse {
// A detailed response message.
string message = 1;
// An enum with value set as "created" or "updated"
string status = 2;
}
message DropCollectionRequest {
// Database name of the collection.
string db = 1;
// Collection name to drop.
string collection = 2;
CollectionOptions options = 3;
}
message DropCollectionResponse {
// A detailed response message.
string message = 1;
// An enum with value set as "dropped".
string status = 2;
}
message DatabaseInfo {
// Database name.
string db = 1;
// Metadata about the database. (ex. ...)
DatabaseMetadata metadata = 2;
}
message CollectionInfo {
// Collection name.
string collection = 1;
// Metadata about the collection.
CollectionMetadata metadata = 2;
}
message ListDatabasesRequest {
}
message ListDatabasesResponse {
// List of the databases.
repeated DatabaseInfo databases = 1;
}
message ListCollectionsRequest {
// List collection in this database.
string db = 1;
CollectionOptions options = 2;
}
message ListCollectionsResponse {
// Name of the database.
string db = 1;
// List of the collections info in the database.
repeated CollectionInfo collections = 2;
}
message DescribeDatabaseRequest {
// Name of the database.
string db = 1;
}
message DescribeCollectionRequest {
// Name of the database.
string db = 1;
// Name of the collection.
string collection = 2;
// Collection options.
CollectionOptions options = 3;
}
// A detailed description about the database and all associated collections.
// Description of the collection includes schema details as well.
message DescribeDatabaseResponse {
// Name of the database.
string db = 1;
// Metadata about the database.
DatabaseMetadata metadata = 2;
// A detailed description about all the collections.
// The description returns collection metadata and the schema.
repeated CollectionDescription collections = 3;
}
// A detailed description about the collection.
// The description returns collection metadata and the schema.
message DescribeCollectionResponse {
// Name of the collection.
string collection = 1;
// Metadata about the collection.
CollectionMetadata metadata = 2;
// Collections schema
bytes schema = 3;
}
message DatabaseDescription {
}
message CollectionDescription {
// Name of the collection.
string collection = 1;
// Metadata about the collection.
CollectionMetadata metadata = 2;
// Collections schema
bytes schema = 3;
}
message DatabaseMetadata {
}
message CollectionMetadata {
}
message StreamRequest {
string db = 1;
}
message StreamResponse {
repeated StreamChange changes = 1;
}
message StreamChange {
string collection_name = 1;
bytes data = 2;
}
service TigrisDB {
// Starts a new transaction and returns a transactional object. All reads/writes performed
// within a transaction will run with serializable isolation.
rpc BeginTransaction(BeginTransactionRequest) returns (BeginTransactionResponse) {
option (google.api.http) = {
post : "/api/v1/databases/{db}/transactions/begin"
body : "*"
};
}
// Atomically commit all the changes performed in the context of the transaction. Commit provides all
// or nothing semantics by ensuring no partial updates are in the database due to a transaction failure.
rpc CommitTransaction(CommitTransactionRequest) returns (CommitTransactionResponse) {
option (google.api.http) = {
post : "/api/v1/databases/{db}/transactions/commit"
body : "*"
};
}
// Rollback transaction discards all the changes
// performed in the transaction
rpc RollbackTransaction(RollbackTransactionRequest) returns (RollbackTransactionResponse) {
option (google.api.http) = {
post : "/api/v1/databases/{db}/transactions/rollback"
body : "*"
};
}
// Inserts new documents in the collection and returns an AlreadyExists error if any of the documents
// in the request already exists. Insert provides idempotency by returning an error if the document
// already exists. To replace documents, use REPLACE API instead of INSERT.
rpc Insert(InsertRequest) returns (InsertResponse) {
option (google.api.http) = {
post : "/api/v1/databases/{db}/collections/{collection}/documents/insert"
body : "*"
};
}
// Inserts the documents or replaces the existing documents in the collections.
rpc Replace(ReplaceRequest) returns (ReplaceResponse) {
option (google.api.http) = {
put : "/api/v1/databases/{db}/collections/{collection}/documents/replace"
body : "*"
};
}
// Delete a range of documents in the collection using the condition provided in the filter.
rpc Delete(DeleteRequest) returns (DeleteResponse) {
option (google.api.http) = {
delete : "/api/v1/databases/{db}/collections/{collection}/documents/delete"
body : "*"
};
}
// Update a range of documents in the collection using the condition provided in the filter.
rpc Update(UpdateRequest) returns (UpdateResponse) {
option (google.api.http) = {
put : "/api/v1/databases/{db}/collections/{collection}/documents/update"
body : "*"
};
}
// Reads range of documents from the collection using the condition in the filter.
rpc Read(ReadRequest) returns (stream ReadResponse) {
option (google.api.http) = {
post : "/api/v1/databases/{db}/collections/{collection}/documents/read"
body : "*"
};
}
// Creates a new collection or atomically upgrades the collection to the new schema changes in the database
// passed in the request.
rpc CreateOrUpdateCollection(CreateOrUpdateCollectionRequest) returns (CreateOrUpdateCollectionResponse) {
option (google.api.http) = {
post : "/api/v1/databases/{db}/collections/{collection}/createOrUpdate"
body : "*"
};
}
// Drop the collection and all its documents in the database passed in the request.
rpc DropCollection(DropCollectionRequest) returns (DropCollectionResponse) {
option (google.api.http) = {
delete : "/api/v1/databases/{db}/collections/{collection}/drop"
body : "*"
};
}
// List returns all the databases.
rpc ListDatabases(ListDatabasesRequest) returns (ListDatabasesResponse) {
option (google.api.http) = {
post : "/api/v1/databases/list"
};
}
// List all collections in the database passed in the request.
rpc ListCollections(ListCollectionsRequest) returns (ListCollectionsResponse) {
option (google.api.http) = {
post : "/api/v1/databases/{db}/collections/list"
body : "*"
};
}
// Creates a new database and returns a AlreadyExists error if the database already exists.
rpc CreateDatabase(CreateDatabaseRequest) returns (CreateDatabaseResponse) {
option (google.api.http) = {
post : "/api/v1/databases/{db}/create"
body : "*"
};
}
// Drop database deletes all the collections in the database along with all it documents.
rpc DropDatabase(DropDatabaseRequest) returns (DropDatabaseResponse) {
option (google.api.http) = {
delete : "/api/v1/databases/{db}/drop"
body : "*"
};
}
// Describe database describes the information related to database along
// with all the collections inside database.
rpc DescribeDatabase(DescribeDatabaseRequest) returns (DescribeDatabaseResponse) {
option (google.api.http) = {
post : "/api/v1/databases/{db}/describe"
body : "*"
};
}
// Describe collection describes the information related to collection.
rpc DescribeCollection(DescribeCollectionRequest) returns (DescribeCollectionResponse) {
option (google.api.http) = {
post : "/api/v1/databases/{db}/collections/{collection}/describe"
body : "*"
};
}
rpc Stream(StreamRequest) returns (stream StreamResponse) {
option (google.api.http) = {
post : "/api/v1/databases/{db}/stream"
body : "*"
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy