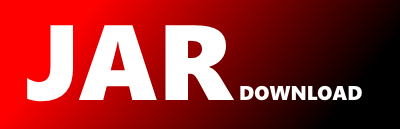
com.tinesoft.gwt.pixlr.client.util.StringUtils Maven / Gradle / Ivy
package com.tinesoft.gwt.pixlr.client.util;
/**
* A Simple String utility class (inspired from Apache commons-lang's StringUtils)
*
* @author Tine Kondo
* @version $Id$
*/
public class StringUtils {
/**
*
* Checks if the String contains only unicode letters.
*
*
*
* null
will return false
. An empty String ("") will return
* true
.
*
*
*
* StringUtils.isAlpha(null) = false
* StringUtils.isAlpha("") = true
* StringUtils.isAlpha(" ") = false
* StringUtils.isAlpha("abc") = true
* StringUtils.isAlpha("ab2c") = false
* StringUtils.isAlpha("ab-c") = false
*
*
* @param str the String to check, may be null
* @return true
if only contains letters, and is non-null
*/
public static boolean isAlpha(final String str) {
if (str == null) {
return false;
}
final int sz = str.length();
for (int i = 0; i < sz; i++) {
if (Character.isLetter(str.charAt(i)) == false) {
return false;
}
}
return true;
}
/**
*
* Checks if the String contains only unicode letters or digits.
*
*
*
* null
will return false
. An empty String ("") will return
* true
.
*
*
*
* StringUtils.isAlphanumeric(null) = false
* StringUtils.isAlphanumeric("") = true
* StringUtils.isAlphanumeric(" ") = false
* StringUtils.isAlphanumeric("abc") = true
* StringUtils.isAlphanumeric("ab c") = false
* StringUtils.isAlphanumeric("ab2c") = true
* StringUtils.isAlphanumeric("ab-c") = false
*
*
* @param str the String to check, may be null
* @return true
if only contains letters or digits, and is non-null
*/
public static boolean isAlphanumeric(final String str) {
if (str == null) {
return false;
}
final int sz = str.length();
for (int i = 0; i < sz; i++) {
if (Character.isLetterOrDigit(str.charAt(i)) == false) {
return false;
}
}
return true;
}
/**
*
* Checks if the String contains only unicode letters, digits or space (' '
).
*
*
*
* null
will return false
. An empty String ("") will return
* true
.
*
*
*
* StringUtils.isAlphanumeric(null) = false
* StringUtils.isAlphanumeric("") = true
* StringUtils.isAlphanumeric(" ") = true
* StringUtils.isAlphanumeric("abc") = true
* StringUtils.isAlphanumeric("ab c") = true
* StringUtils.isAlphanumeric("ab2c") = true
* StringUtils.isAlphanumeric("ab-c") = false
*
*
* @param str the String to check, may be null
* @return true
if only contains letters, digits or space, and is non-null
*/
public static boolean isAlphanumericSpace(final String str) {
if (str == null) {
return false;
}
final int sz = str.length();
for (int i = 0; i < sz; i++) {
if ((Character.isLetterOrDigit(str.charAt(i)) == false) && (str.charAt(i) != ' ')) {
return false;
}
}
return true;
}
/**
*
* Checks if a String is whitespace, empty ("") or null.
*
*
*
* StringUtils.isBlank(null) = true
* StringUtils.isBlank("") = true
* StringUtils.isBlank(" ") = true
* StringUtils.isBlank("bob") = false
* StringUtils.isBlank(" bob ") = false
*
*
* @param str the String to check, may be null
* @return true
if the String is null, empty or whitespace
* @since 2.0
*/
public static boolean isBlank(final String str) {
if ((str == null) || (str.trim().length() == 0)) {
return true;
}
return false;
}
/**
*
* Checks if a String is empty ("") or null.
*
*
*
* StringUtils.isEmpty(null) = true
* StringUtils.isEmpty("") = true
* StringUtils.isEmpty(" ") = false
* StringUtils.isEmpty("bob") = false
* StringUtils.isEmpty(" bob ") = false
*
*
*
* NOTE: This method does not trim the String. That functionality is available in isBlank().
*
*
* @param str the String to check, may be null
* @return true
if the String is empty or null
*/
public static boolean isEmpty(final String str) {
return (str == null) || (str.length() == 0);
}
/**
*
* Checks if a String is not empty (""), not null and not whitespace only.
*
*
*
* StringUtils.isNotBlank(null) = false
* StringUtils.isNotBlank("") = false
* StringUtils.isNotBlank(" ") = false
* StringUtils.isNotBlank("bob") = true
* StringUtils.isNotBlank(" bob ") = true
*
*
* @param str the String to check, may be null
* @return true
if the String is not empty and not null and not whitespace
*/
public static boolean isNotBlank(final String str) {
return !StringUtils.isBlank(str);
}
/**
*
* Checks if a String is not empty ("") and not null.
*
*
*
* StringUtils.isNotEmpty(null) = false
* StringUtils.isNotEmpty("") = false
* StringUtils.isNotEmpty(" ") = true
* StringUtils.isNotEmpty("bob") = true
* StringUtils.isNotEmpty(" bob ") = true
*
*
* @param str the String to check, may be null
* @return true
if the String is not empty and not null
*/
public static boolean isNotEmpty(final String str) {
return !StringUtils.isEmpty(str);
}
/**
*
* Checks if the String contains only unicode digits. A decimal point is not a unicode digit and
* returns false.
*
*
*
* null
will return false
. An empty String ("") will return
* true
.
*
*
*
* StringUtils.isNumeric(null) = false
* StringUtils.isNumeric("") = true
* StringUtils.isNumeric(" ") = false
* StringUtils.isNumeric("123") = true
* StringUtils.isNumeric("12 3") = false
* StringUtils.isNumeric("ab2c") = false
* StringUtils.isNumeric("12-3") = false
* StringUtils.isNumeric("12.3") = false
*
*
* @param str the String to check, may be null
* @return true
if only contains digits, and is non-null
*/
public static boolean isNumeric(final String str) {
if (str == null) {
return false;
}
final int sz = str.length();
for (int i = 0; i < sz; i++) {
if (Character.isDigit(str.charAt(i)) == false) {
return false;
}
}
return true;
}
/**
*
* Checks if the String contains only unicode digits or space (' '
). A decimal
* point is not a unicode digit and returns false.
*
*
*
* null
will return false
. An empty String ("") will return
* true
.
*
*
*
* StringUtils.isNumeric(null) = false
* StringUtils.isNumeric("") = true
* StringUtils.isNumeric(" ") = true
* StringUtils.isNumeric("123") = true
* StringUtils.isNumeric("12 3") = true
* StringUtils.isNumeric("ab2c") = false
* StringUtils.isNumeric("12-3") = false
* StringUtils.isNumeric("12.3") = false
*
*
* @param str the String to check, may be null
* @return true
if only contains digits or space, and is non-null
*/
public static boolean isNumericSpace(final String str) {
if (str == null) {
return false;
}
final int sz = str.length();
for (int i = 0; i < sz; i++) {
if ((Character.isDigit(str.charAt(i)) == false) && (str.charAt(i) != ' ')) {
return false;
}
}
return true;
}
/**
* Utility class No public constructor
**/
public StringUtils() {
}
}