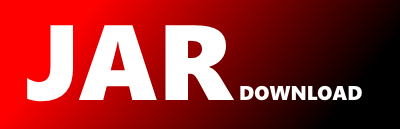
com.tink.rest.models.Account.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest Show documentation
Show all versions of rest Show documentation
A library for Tink core services
/**
* NOTE: This class is auto generated by the Swagger Gradle Codegen for the following API: Tink API
*
* More info on this tool is available on https://github.com/Yelp/swagger-gradle-codegen
*/
package com.tink.rest.models
import com.squareup.moshi.Json
import com.squareup.moshi.JsonClass
/**
* An account could either be a debit account, a credit card, a loan or mortgage.
* @property accountNumber The account number of the account. The format of the account numbers may differ between account types and banks. This property can be updated in a update account request.
* @property balance The current balance of the account. The definition of the balance property differs between account types. `SAVINGS`: the balance represent the actual amount of cash in the account, `INVESTMENT`: the balance represents the value of the investments connected to this accounts including any available cash, `MORTGAGE`: the balance represents the loan debt outstanding from this account, `CREDIT_CARD: the balance represent the outstanding balance on the account, it does not include any available credit or purchasing power the user has with the credit provider.
* @property credentialsId The internal identifier of the credentials that the account belongs to.
* @property excluded Indicates if the user has excluded the account. Categorization and PFM Features are excluded, and transactions belonging to this account are not searchable. This property can be updated in a update account request.
* @property favored Indicates if the user has favored the account. This property can be updated in a update account request.
* @property id The internal identifier of account.
* @property name The display name of the account. This property can be updated in a update account request.
* @property ownership The ownership ratio indicating how much of the account is owned by the user. The ownership determine the percentage of the amounts on transactions belonging to this account, that should be attributed to the user when statistics are calculated. This property has a default value, and it can only be updated by you in a update account request.
* @property type The type of the account. This property can be updated in a update account request.
* @property identifiers All possible ways to uniquely identify this `Account`. An se-identifier is built up like: `se://{clearingnumber}{accountnumber}`.
* @property transferDestinations The destinations this Account can transfer money to, be that payment or bank transfer recipients. This field is only populated if account data is requested via GET /transfer/accounts.
* @property details Details contains information only applicable for accounts of the types loans and mortgages. All banks do not offer detail information about their loan and mortgages therefore will details not be present on all accounts of the types loan and mortgages.
* @property holderName The name of the account holder.
* @property closed A closed account indicates that it was no longer available from the connected financial institution, most likely due to it having been closed by the user.
* @property flags A list of flags specifying attributes on an account.
* @property accountExclusion Indicates features this account should be excluded from. Possible values are: `NONE`: No features are excluded from this account, `PFM_DATA`: Personal Finance Management Features, like statistics and activities are excluded, `PFM_AND_SEARCH`: Personal Finance Management Features are excluded, and transactions belonging to this account are not searchable. This is the equivalent of the, now deprecated, boolean flag 'excluded', `AGGREGATION`: No data will be aggregated for this account and, all data associated with the account is removed (except account name and account number). This property can be updated in a update account request.
* @property currencyDenominatedBalance The current balance of the account. The definition of the balance property differ between account types. `SAVINGS`: the balance represent the actual amount of cash in the account, `INVESTMENT`: the balance represents the value of the investments connected to this accounts including any available cash, `MORTGAGE`: the balance represents the loan debt outstanding from this account, `CREDIT_CARD`: the balance represent the outstanding balance on the account, it does not include any available credit or purchasing power the user has with the credit provider. The balance is represented as a scale and unscaled value together with the ISO 4217 currency code of the amount.
* @property refreshed Timestamp of when the account was last refreshed.
* @property financialInstitutionId A unique identifier to group accounts belonging the same financial institution. Available for aggregated accounts only.
*/
@JsonClass(generateAdapter = true)
data class Account(
@Json(name = "accountNumber") @field:Json(name = "accountNumber") var accountNumber: String,
@Json(name = "balance") @field:Json(name = "balance") var balance: Double,
@Json(name = "credentialsId") @field:Json(name = "credentialsId") var credentialsId: String,
@Json(name = "excluded") @field:Json(name = "excluded") var excluded: Boolean,
@Json(name = "favored") @field:Json(name = "favored") var favored: Boolean,
@Json(name = "flags") @field:Json(name = "flags") var flags: String? = null,
@Json(name = "id") @field:Json(name = "id") var id: String,
@Json(name = "name") @field:Json(name = "name") var name: String,
@Json(name = "ownership") @field:Json(name = "ownership") var ownership: Double,
@Json(name = "type") @field:Json(name = "type") var type: Account.TypeEnum,
@Json(name = "accountExclusion") @field:Json(name = "accountExclusion") var accountExclusion: Account.AccountExclusionEnum,
@Json(name = "identifiers") @field:Json(name = "identifiers") var identifiers: String? = null,
@Json(name = "transferDestinations") @field:Json(name = "transferDestinations") var transferDestinations: List? = null,
@Json(name = "details") @field:Json(name = "details") var details: AccountDetails? = null,
@Json(name = "holderName") @field:Json(name = "holderName") var holderName: String? = null,
@Json(name = "closed") @field:Json(name = "closed") var closed: Boolean? = null,
@Json(name = "currencyDenominatedBalance") @field:Json(name = "currencyDenominatedBalance") var currencyDenominatedBalance: CurrencyDenominatedAmount? = null,
@Json(name = "refreshed") @field:Json(name = "refreshed") var refreshed: Long? = null,
@Json(name = "financialInstitutionId") @field:Json(name = "financialInstitutionId") var financialInstitutionId: String? = null,
@Json(name = "firstSeen") @field:Json(name = "firstSeen") var firstSeen: Long? = null
) {
/**
* The type of the account. This property can be updated in a update account request.
* Values: CHECKING, SAVINGS, INVESTMENT, MORTGAGE, CREDIT_CARD, LOAN, PENSION, OTHER, EXTERNAL
*/
@JsonClass(generateAdapter = false)
enum class TypeEnum(val value: String) {
@Json(name = "UNKNOWN") UNKNOWN("UNKNOWN"),
@Json(name = "CHECKING") CHECKING("CHECKING"),
@Json(name = "SAVINGS") SAVINGS("SAVINGS"),
@Json(name = "INVESTMENT") INVESTMENT("INVESTMENT"),
@Json(name = "MORTGAGE") MORTGAGE("MORTGAGE"),
@Json(name = "CREDIT_CARD") CREDIT_CARD("CREDIT_CARD"),
@Json(name = "LOAN") LOAN("LOAN"),
@Json(name = "PENSION") PENSION("PENSION"),
@Json(name = "OTHER") OTHER("OTHER"),
@Json(name = "EXTERNAL") EXTERNAL("EXTERNAL")
}
/**
* A list of flags specifying attributes on an account.
* Values: BUSINESS, MANDATE
*/
@JsonClass(generateAdapter = false)
enum class FlagsEnum(val value: String) {
@Json(name = "UNKNOWN") UNKNOWN("UNKNOWN"),
@Json(name = "BUSINESS") BUSINESS("BUSINESS"),
@Json(name = "MANDATE") MANDATE("MANDATE")
}
/**
* Indicates features this account should be excluded from. Possible values are: `NONE`: No features are excluded from this account, `PFM_DATA`: Personal Finance Management Features, like statistics and activities are excluded, `PFM_AND_SEARCH`: Personal Finance Management Features are excluded, and transactions belonging to this account are not searchable. This is the equivalent of the, now deprecated, boolean flag 'excluded', `AGGREGATION`: No data will be aggregated for this account and, all data associated with the account is removed (except account name and account number). This property can be updated in a update account request.
* Values: AGGREGATION, PFM_AND_SEARCH, PFM_DATA, NONE
*/
@JsonClass(generateAdapter = false)
enum class AccountExclusionEnum(val value: String) {
@Json(name = "UNKNOWN") UNKNOWN("UNKNOWN"),
@Json(name = "AGGREGATION") AGGREGATION("AGGREGATION"),
@Json(name = "PFM_AND_SEARCH") PFM_AND_SEARCH("PFM_AND_SEARCH"),
@Json(name = "PFM_DATA") PFM_DATA("PFM_DATA"),
@Json(name = "NONE") NONE("NONE")
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy