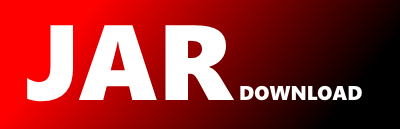
com.tinkerforge.BrickletEnergyMonitor Maven / Gradle / Ivy
/* ***********************************************************
* This file was automatically generated on 2019-11-25. *
* *
* Java Bindings Version 2.1.25 *
* *
* If you have a bugfix for this file and want to commit it, *
* please fix the bug in the generator. You can find a link *
* to the generators git repository on tinkerforge.com *
*************************************************************/
package com.tinkerforge;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.util.concurrent.CopyOnWriteArrayList;
import java.util.Arrays;
import java.util.List;
/**
* Measures Voltage, Current, Energy, Real/Apparent/Reactive Power, Power Factor and Frequency
*/
public class BrickletEnergyMonitor extends Device {
public final static int DEVICE_IDENTIFIER = 2152;
public final static String DEVICE_DISPLAY_NAME = "Energy Monitor Bricklet";
public final static byte FUNCTION_GET_ENERGY_DATA = (byte)1;
public final static byte FUNCTION_RESET_ENERGY = (byte)2;
public final static byte FUNCTION_GET_WAVEFORM_LOW_LEVEL = (byte)3;
public final static byte FUNCTION_GET_TRANSFORMER_STATUS = (byte)4;
public final static byte FUNCTION_SET_TRANSFORMER_CALIBRATION = (byte)5;
public final static byte FUNCTION_GET_TRANSFORMER_CALIBRATION = (byte)6;
public final static byte FUNCTION_CALIBRATE_OFFSET = (byte)7;
public final static byte FUNCTION_SET_ENERGY_DATA_CALLBACK_CONFIGURATION = (byte)8;
public final static byte FUNCTION_GET_ENERGY_DATA_CALLBACK_CONFIGURATION = (byte)9;
public final static byte FUNCTION_GET_SPITFP_ERROR_COUNT = (byte)234;
public final static byte FUNCTION_SET_BOOTLOADER_MODE = (byte)235;
public final static byte FUNCTION_GET_BOOTLOADER_MODE = (byte)236;
public final static byte FUNCTION_SET_WRITE_FIRMWARE_POINTER = (byte)237;
public final static byte FUNCTION_WRITE_FIRMWARE = (byte)238;
public final static byte FUNCTION_SET_STATUS_LED_CONFIG = (byte)239;
public final static byte FUNCTION_GET_STATUS_LED_CONFIG = (byte)240;
public final static byte FUNCTION_GET_CHIP_TEMPERATURE = (byte)242;
public final static byte FUNCTION_RESET = (byte)243;
public final static byte FUNCTION_WRITE_UID = (byte)248;
public final static byte FUNCTION_READ_UID = (byte)249;
public final static byte FUNCTION_GET_IDENTITY = (byte)255;
private final static int CALLBACK_ENERGY_DATA = 10;
public final static int BOOTLOADER_MODE_BOOTLOADER = 0;
public final static int BOOTLOADER_MODE_FIRMWARE = 1;
public final static int BOOTLOADER_MODE_BOOTLOADER_WAIT_FOR_REBOOT = 2;
public final static int BOOTLOADER_MODE_FIRMWARE_WAIT_FOR_REBOOT = 3;
public final static int BOOTLOADER_MODE_FIRMWARE_WAIT_FOR_ERASE_AND_REBOOT = 4;
public final static int BOOTLOADER_STATUS_OK = 0;
public final static int BOOTLOADER_STATUS_INVALID_MODE = 1;
public final static int BOOTLOADER_STATUS_NO_CHANGE = 2;
public final static int BOOTLOADER_STATUS_ENTRY_FUNCTION_NOT_PRESENT = 3;
public final static int BOOTLOADER_STATUS_DEVICE_IDENTIFIER_INCORRECT = 4;
public final static int BOOTLOADER_STATUS_CRC_MISMATCH = 5;
public final static int STATUS_LED_CONFIG_OFF = 0;
public final static int STATUS_LED_CONFIG_ON = 1;
public final static int STATUS_LED_CONFIG_SHOW_HEARTBEAT = 2;
public final static int STATUS_LED_CONFIG_SHOW_STATUS = 3;
private List listenerEnergyData = new CopyOnWriteArrayList();
public class EnergyData {
public int voltage;
public int current;
public int energy;
public int realPower;
public int apparentPower;
public int reactivePower;
public int powerFactor;
public int frequency;
public String toString() {
return "[" + "voltage = " + voltage + ", " + "current = " + current + ", " + "energy = " + energy + ", " + "realPower = " + realPower + ", " + "apparentPower = " + apparentPower + ", " + "reactivePower = " + reactivePower + ", " + "powerFactor = " + powerFactor + ", " + "frequency = " + frequency + "]";
}
}
public class WaveformLowLevel {
public int waveformChunkOffset;
public int[] waveformChunkData = new int[30];
public String toString() {
return "[" + "waveformChunkOffset = " + waveformChunkOffset + ", " + "waveformChunkData = " + Arrays.toString(waveformChunkData) + "]";
}
}
public class TransformerStatus {
public boolean voltageTransformerConnected;
public boolean currentTransformerConnected;
public String toString() {
return "[" + "voltageTransformerConnected = " + voltageTransformerConnected + ", " + "currentTransformerConnected = " + currentTransformerConnected + "]";
}
}
public class TransformerCalibration {
public int voltageRatio;
public int currentRatio;
public int phaseShift;
public String toString() {
return "[" + "voltageRatio = " + voltageRatio + ", " + "currentRatio = " + currentRatio + ", " + "phaseShift = " + phaseShift + "]";
}
}
public class EnergyDataCallbackConfiguration {
public long period;
public boolean valueHasToChange;
public String toString() {
return "[" + "period = " + period + ", " + "valueHasToChange = " + valueHasToChange + "]";
}
}
public class SPITFPErrorCount {
public long errorCountAckChecksum;
public long errorCountMessageChecksum;
public long errorCountFrame;
public long errorCountOverflow;
public String toString() {
return "[" + "errorCountAckChecksum = " + errorCountAckChecksum + ", " + "errorCountMessageChecksum = " + errorCountMessageChecksum + ", " + "errorCountFrame = " + errorCountFrame + ", " + "errorCountOverflow = " + errorCountOverflow + "]";
}
}
/**
* This listener is triggered periodically according to the configuration set by
* {@link BrickletEnergyMonitor#setEnergyDataCallbackConfiguration(long, boolean)}.
*
* The parameters are the same as {@link BrickletEnergyMonitor#getEnergyData()}.
*/
public interface EnergyDataListener extends DeviceListener {
public void energyData(int voltage, int current, int energy, int realPower, int apparentPower, int reactivePower, int powerFactor, int frequency);
}
/**
* Creates an object with the unique device ID \c uid. and adds it to
* the IP Connection \c ipcon.
*/
public BrickletEnergyMonitor(String uid, IPConnection ipcon) {
super(uid, ipcon);
apiVersion[0] = 2;
apiVersion[1] = 0;
apiVersion[2] = 0;
responseExpected[IPConnection.unsignedByte(FUNCTION_GET_ENERGY_DATA)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_RESET_ENERGY)] = RESPONSE_EXPECTED_FLAG_FALSE;
responseExpected[IPConnection.unsignedByte(FUNCTION_GET_WAVEFORM_LOW_LEVEL)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_GET_TRANSFORMER_STATUS)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_SET_TRANSFORMER_CALIBRATION)] = RESPONSE_EXPECTED_FLAG_FALSE;
responseExpected[IPConnection.unsignedByte(FUNCTION_GET_TRANSFORMER_CALIBRATION)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_CALIBRATE_OFFSET)] = RESPONSE_EXPECTED_FLAG_FALSE;
responseExpected[IPConnection.unsignedByte(FUNCTION_SET_ENERGY_DATA_CALLBACK_CONFIGURATION)] = RESPONSE_EXPECTED_FLAG_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_GET_ENERGY_DATA_CALLBACK_CONFIGURATION)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_GET_SPITFP_ERROR_COUNT)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_SET_BOOTLOADER_MODE)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_GET_BOOTLOADER_MODE)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_SET_WRITE_FIRMWARE_POINTER)] = RESPONSE_EXPECTED_FLAG_FALSE;
responseExpected[IPConnection.unsignedByte(FUNCTION_WRITE_FIRMWARE)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_SET_STATUS_LED_CONFIG)] = RESPONSE_EXPECTED_FLAG_FALSE;
responseExpected[IPConnection.unsignedByte(FUNCTION_GET_STATUS_LED_CONFIG)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_GET_CHIP_TEMPERATURE)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_RESET)] = RESPONSE_EXPECTED_FLAG_FALSE;
responseExpected[IPConnection.unsignedByte(FUNCTION_WRITE_UID)] = RESPONSE_EXPECTED_FLAG_FALSE;
responseExpected[IPConnection.unsignedByte(FUNCTION_READ_UID)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnection.unsignedByte(FUNCTION_GET_IDENTITY)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
callbacks[CALLBACK_ENERGY_DATA] = new IPConnection.DeviceCallbackListener() {
public void callback(byte[] packet) {
ByteBuffer bb = ByteBuffer.wrap(packet, 8, packet.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
int voltage = (bb.getInt());
int current = (bb.getInt());
int energy = (bb.getInt());
int realPower = (bb.getInt());
int apparentPower = (bb.getInt());
int reactivePower = (bb.getInt());
int powerFactor = IPConnection.unsignedShort(bb.getShort());
int frequency = IPConnection.unsignedShort(bb.getShort());
for (EnergyDataListener listener: listenerEnergyData) {
listener.energyData(voltage, current, energy, realPower, apparentPower, reactivePower, powerFactor, frequency);
}
}
};
}
/**
* Returns all of the measurements that are done by the Energy Monitor Bricklet.
*
* * Voltage RMS
* * Current RMS
* * Energy (integrated over time)
* * Real Power
* * Apparent Power
* * Reactive Power
* * Power Factor
* * Frequency (AC Frequency of the mains voltage)
*
* The frequency is recalculated every 6 seconds.
*
* All other values are integrated over 10 zero-crossings of the voltage sine wave.
* With a standard AC mains voltage frequency of 50Hz this results in a 5 measurements
* per second (or an integration time of 200ms per measurement).
*
* If no voltage transformer is connected, the Bricklet will use the current waveform
* to calculate the frequency and it will use an integration time of
* 10 zero-crossings of the current waveform.
*/
public EnergyData getEnergyData() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_ENERGY_DATA, this);
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
EnergyData obj = new EnergyData();
obj.voltage = (bb.getInt());
obj.current = (bb.getInt());
obj.energy = (bb.getInt());
obj.realPower = (bb.getInt());
obj.apparentPower = (bb.getInt());
obj.reactivePower = (bb.getInt());
obj.powerFactor = IPConnection.unsignedShort(bb.getShort());
obj.frequency = IPConnection.unsignedShort(bb.getShort());
return obj;
}
/**
* Sets the energy value (see {@link BrickletEnergyMonitor#getEnergyData()}) back to 0Wh.
*/
public void resetEnergy() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_RESET_ENERGY, this);
sendRequest(bb.array());
}
/**
* Returns a snapshot of the voltage and current waveform. The values
* in the returned array alternate between voltage and current. The data from
* one getter call contains 768 data points for voltage and current, which
* correspond to about 3 full sine waves.
*
* The voltage is given with a resolution of 100mV and the current is given
* with a resolution of 10mA.
*
* This data is meant to be used for a non-realtime graphical representation of
* the voltage and current waveforms.
*/
public WaveformLowLevel getWaveformLowLevel() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_WAVEFORM_LOW_LEVEL, this);
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
WaveformLowLevel obj = new WaveformLowLevel();
obj.waveformChunkOffset = IPConnection.unsignedShort(bb.getShort());
for (int i = 0; i < 30; i++) {
obj.waveformChunkData[i] = (bb.getShort());
}
return obj;
}
/**
* Returns *true* if a voltage/current transformer is connected to the Bricklet.
*/
public TransformerStatus getTransformerStatus() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_TRANSFORMER_STATUS, this);
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
TransformerStatus obj = new TransformerStatus();
obj.voltageTransformerConnected = (bb.get()) != 0;
obj.currentTransformerConnected = (bb.get()) != 0;
return obj;
}
/**
* Sets the transformer ratio for the voltage and current transformer in 1/100 form.
*
* Example: If your mains voltage is 230V, you use 9V voltage transformer and a
* 1V:30A current clamp your voltage ratio is 230/9 = 25.56 and your current ratio
* is 30/1 = 30.
*
* In this case you have to set the values 2556 and 3000 for voltage ratio and current
* ratio.
*
* The calibration is saved in non-volatile memory, you only have to set it once.
*
* Set the phase shift to 0. It is for future use and currently not supported by the Bricklet.
*/
public void setTransformerCalibration(int voltageRatio, int currentRatio, int phaseShift) throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)14, FUNCTION_SET_TRANSFORMER_CALIBRATION, this);
bb.putShort((short)voltageRatio);
bb.putShort((short)currentRatio);
bb.putShort((short)phaseShift);
sendRequest(bb.array());
}
/**
* Returns the transformer calibration as set by {@link BrickletEnergyMonitor#setTransformerCalibration(int, int, int)}.
*/
public TransformerCalibration getTransformerCalibration() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_TRANSFORMER_CALIBRATION, this);
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
TransformerCalibration obj = new TransformerCalibration();
obj.voltageRatio = IPConnection.unsignedShort(bb.getShort());
obj.currentRatio = IPConnection.unsignedShort(bb.getShort());
obj.phaseShift = (bb.getShort());
return obj;
}
/**
* Calling this function will start an offset calibration. The offset calibration will
* integrate the voltage and current waveform over a longer time period to find the 0
* transition point in the sine wave.
*
* The Bricklet comes with a factory-calibrated offset value, you should not have to
* call this function.
*
* If you want to re-calibrate the offset we recommend that you connect a load that
* has a smooth sinusoidal voltage and current waveform. Alternatively you can also
* short both inputs.
*
* The calibration is saved in non-volatile memory, you only have to set it once.
*/
public void calibrateOffset() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_CALIBRATE_OFFSET, this);
sendRequest(bb.array());
}
/**
* The period is the period with which the {@link BrickletEnergyMonitor.EnergyDataListener}
* listener is triggered periodically. A value of 0 turns the listener off.
*
* If the `value has to change`-parameter is set to true, the listener is only
* triggered after the value has changed. If the value didn't change within the
* period, the listener is triggered immediately on change.
*
* If it is set to false, the listener is continuously triggered with the period,
* independent of the value.
*/
public void setEnergyDataCallbackConfiguration(long period, boolean valueHasToChange) throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)13, FUNCTION_SET_ENERGY_DATA_CALLBACK_CONFIGURATION, this);
bb.putInt((int)period);
bb.put((byte)(valueHasToChange ? 1 : 0));
sendRequest(bb.array());
}
/**
* Returns the listener configuration as set by
* {@link BrickletEnergyMonitor#setEnergyDataCallbackConfiguration(long, boolean)}.
*/
public EnergyDataCallbackConfiguration getEnergyDataCallbackConfiguration() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_ENERGY_DATA_CALLBACK_CONFIGURATION, this);
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
EnergyDataCallbackConfiguration obj = new EnergyDataCallbackConfiguration();
obj.period = IPConnection.unsignedInt(bb.getInt());
obj.valueHasToChange = (bb.get()) != 0;
return obj;
}
/**
* Returns the error count for the communication between Brick and Bricklet.
*
* The errors are divided into
*
* * ACK checksum errors,
* * message checksum errors,
* * framing errors and
* * overflow errors.
*
* The errors counts are for errors that occur on the Bricklet side. All
* Bricks have a similar function that returns the errors on the Brick side.
*/
public SPITFPErrorCount getSPITFPErrorCount() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_SPITFP_ERROR_COUNT, this);
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
SPITFPErrorCount obj = new SPITFPErrorCount();
obj.errorCountAckChecksum = IPConnection.unsignedInt(bb.getInt());
obj.errorCountMessageChecksum = IPConnection.unsignedInt(bb.getInt());
obj.errorCountFrame = IPConnection.unsignedInt(bb.getInt());
obj.errorCountOverflow = IPConnection.unsignedInt(bb.getInt());
return obj;
}
/**
* Sets the bootloader mode and returns the status after the requested
* mode change was instigated.
*
* You can change from bootloader mode to firmware mode and vice versa. A change
* from bootloader mode to firmware mode will only take place if the entry function,
* device identifier and CRC are present and correct.
*
* This function is used by Brick Viewer during flashing. It should not be
* necessary to call it in a normal user program.
*/
public int setBootloaderMode(int mode) throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)9, FUNCTION_SET_BOOTLOADER_MODE, this);
bb.put((byte)mode);
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
int status = IPConnection.unsignedByte(bb.get());
return status;
}
/**
* Returns the current bootloader mode, see {@link BrickletEnergyMonitor#setBootloaderMode(int)}.
*/
public int getBootloaderMode() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_BOOTLOADER_MODE, this);
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
int mode = IPConnection.unsignedByte(bb.get());
return mode;
}
/**
* Sets the firmware pointer for {@link BrickletEnergyMonitor#writeFirmware(int[])}. The pointer has
* to be increased by chunks of size 64. The data is written to flash
* every 4 chunks (which equals to one page of size 256).
*
* This function is used by Brick Viewer during flashing. It should not be
* necessary to call it in a normal user program.
*/
public void setWriteFirmwarePointer(long pointer) throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)12, FUNCTION_SET_WRITE_FIRMWARE_POINTER, this);
bb.putInt((int)pointer);
sendRequest(bb.array());
}
/**
* Writes 64 Bytes of firmware at the position as written by
* {@link BrickletEnergyMonitor#setWriteFirmwarePointer(long)} before. The firmware is written
* to flash every 4 chunks.
*
* You can only write firmware in bootloader mode.
*
* This function is used by Brick Viewer during flashing. It should not be
* necessary to call it in a normal user program.
*/
public int writeFirmware(int[] data) throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)72, FUNCTION_WRITE_FIRMWARE, this);
for (int i = 0; i < 64; i++) {
bb.put((byte)data[i]);
}
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
int status = IPConnection.unsignedByte(bb.get());
return status;
}
/**
* Sets the status LED configuration. By default the LED shows
* communication traffic between Brick and Bricklet, it flickers once
* for every 10 received data packets.
*
* You can also turn the LED permanently on/off or show a heartbeat.
*
* If the Bricklet is in bootloader mode, the LED is will show heartbeat by default.
*/
public void setStatusLEDConfig(int config) throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)9, FUNCTION_SET_STATUS_LED_CONFIG, this);
bb.put((byte)config);
sendRequest(bb.array());
}
/**
* Returns the configuration as set by {@link BrickletEnergyMonitor#setStatusLEDConfig(int)}
*/
public int getStatusLEDConfig() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_STATUS_LED_CONFIG, this);
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
int config = IPConnection.unsignedByte(bb.get());
return config;
}
/**
* Returns the temperature in °C as measured inside the microcontroller. The
* value returned is not the ambient temperature!
*
* The temperature is only proportional to the real temperature and it has bad
* accuracy. Practically it is only useful as an indicator for
* temperature changes.
*/
public int getChipTemperature() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_CHIP_TEMPERATURE, this);
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
int temperature = (bb.getShort());
return temperature;
}
/**
* Calling this function will reset the Bricklet. All configurations
* will be lost.
*
* After a reset you have to create new device objects,
* calling functions on the existing ones will result in
* undefined behavior!
*/
public void reset() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_RESET, this);
sendRequest(bb.array());
}
/**
* Writes a new UID into flash. If you want to set a new UID
* you have to decode the Base58 encoded UID string into an
* integer first.
*
* We recommend that you use Brick Viewer to change the UID.
*/
public void writeUID(long uid) throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)12, FUNCTION_WRITE_UID, this);
bb.putInt((int)uid);
sendRequest(bb.array());
}
/**
* Returns the current UID as an integer. Encode as
* Base58 to get the usual string version.
*/
public long readUID() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_READ_UID, this);
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
long uid = IPConnection.unsignedInt(bb.getInt());
return uid;
}
/**
* Returns the UID, the UID where the Bricklet is connected to,
* the position, the hardware and firmware version as well as the
* device identifier.
*
* The position can be 'a', 'b', 'c' or 'd'.
*
* The device identifier numbers can be found :ref:`here <device_identifier>`.
* |device_identifier_constant|
*/
public Identity getIdentity() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_IDENTITY, this);
byte[] response = sendRequest(bb.array());
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
Identity obj = new Identity();
obj.uid = IPConnection.string(bb, 8);
obj.connectedUid = IPConnection.string(bb, 8);
obj.position = (char)(bb.get());
for (int i = 0; i < 3; i++) {
obj.hardwareVersion[i] = IPConnection.unsignedByte(bb.get());
}
for (int i = 0; i < 3; i++) {
obj.firmwareVersion[i] = IPConnection.unsignedByte(bb.get());
}
obj.deviceIdentifier = IPConnection.unsignedShort(bb.getShort());
return obj;
}
/**
* Returns a snapshot of the voltage and current waveform. The values
* in the returned array alternate between voltage and current. The data from
* one getter call contains 768 data points for voltage and current, which
* correspond to about 3 full sine waves.
*
* The voltage is given with a resolution of 100mV and the current is given
* with a resolution of 10mA.
*
* This data is meant to be used for a non-realtime graphical representation of
* the voltage and current waveforms.
*/
public int[] getWaveform() throws TinkerforgeException {
WaveformLowLevel ret;
int[] waveform = null; // stop the compiler from wrongly complaining that this variable is used unassigned
int waveformLength = 1536;
int waveformChunkOffset;
int waveformChunkLength;
boolean waveformOutOfSync;
int waveformCurrentLength;
synchronized (streamMutex) {
ret = getWaveformLowLevel();
if (ret.waveformChunkOffset == (1 << 16) - 1) { // maximum chunk offset -> stream has no data
waveformLength = 0;
waveformChunkOffset = 0;
waveformOutOfSync = false;
} else {
waveformChunkOffset = ret.waveformChunkOffset;
waveformOutOfSync = waveformChunkOffset != 0;
}
if (!waveformOutOfSync) {
waveform = new int[waveformLength];
waveformChunkLength = Math.min(waveformLength - waveformChunkOffset, 30);
System.arraycopy(ret.waveformChunkData, 0, waveform, 0, waveformChunkLength);
waveformCurrentLength = waveformChunkLength;
while (waveformCurrentLength < waveformLength) {
ret = getWaveformLowLevel();
waveformOutOfSync = ret.waveformChunkOffset != waveformCurrentLength;
if (waveformOutOfSync) {
break;
}
waveformChunkLength = Math.min(waveformLength - ret.waveformChunkOffset, 30);
System.arraycopy(ret.waveformChunkData, 0, waveform, waveformCurrentLength, waveformChunkLength);
waveformCurrentLength += waveformChunkLength;
}
}
if (waveformOutOfSync) { // discard remaining stream to bring it back in-sync
while (ret.waveformChunkOffset + 30 < waveformLength) {
ret = getWaveformLowLevel();
}
throw new StreamOutOfSyncException("Waveform stream is out-of-sync");
}
}
return waveform;
}
/**
* Adds a EnergyData listener.
*/
public void addEnergyDataListener(EnergyDataListener listener) {
listenerEnergyData.add(listener);
}
/**
* Removes a EnergyData listener.
*/
public void removeEnergyDataListener(EnergyDataListener listener) {
listenerEnergyData.remove(listener);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy