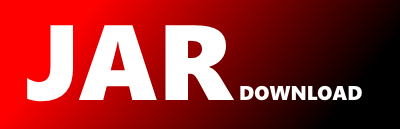
com.tinkerforge.BrickletAnalogOut Maven / Gradle / Ivy
/* ***********************************************************
* This file was automatically generated on 2020-06-25. *
* *
* Java Bindings Version 2.1.28 *
* *
* If you have a bugfix for this file and want to commit it, *
* please fix the bug in the generator. You can find a link *
* to the generators git repository on tinkerforge.com *
*************************************************************/
package com.tinkerforge;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.util.concurrent.CopyOnWriteArrayList;
import java.util.Arrays;
import java.util.List;
/**
* Generates configurable DC voltage between 0V and 5V
*/
public class BrickletAnalogOut extends Device {
public final static int DEVICE_IDENTIFIER = 220;
public final static String DEVICE_DISPLAY_NAME = "Analog Out Bricklet";
public final static byte FUNCTION_SET_VOLTAGE = (byte)1;
public final static byte FUNCTION_GET_VOLTAGE = (byte)2;
public final static byte FUNCTION_SET_MODE = (byte)3;
public final static byte FUNCTION_GET_MODE = (byte)4;
public final static byte FUNCTION_GET_IDENTITY = (byte)255;
public final static short MODE_ANALOG_VALUE = (short)0;
public final static short MODE_1K_TO_GROUND = (short)1;
public final static short MODE_100K_TO_GROUND = (short)2;
public final static short MODE_500K_TO_GROUND = (short)3;
/**
* Creates an object with the unique device ID \c uid. and adds it to
* the IP Connection \c ipcon.
*/
public BrickletAnalogOut(String uid, IPConnection ipcon) {
super(uid, ipcon);
apiVersion[0] = 2;
apiVersion[1] = 0;
apiVersion[2] = 0;
deviceIdentifier = DEVICE_IDENTIFIER;
deviceDisplayName = DEVICE_DISPLAY_NAME;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_SET_VOLTAGE)] = RESPONSE_EXPECTED_FLAG_FALSE;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_GET_VOLTAGE)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_SET_MODE)] = RESPONSE_EXPECTED_FLAG_FALSE;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_GET_MODE)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_GET_IDENTITY)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
ipcon.addDevice(this);
}
/**
* Sets the voltage. Calling this function will set
* the mode to 0 (see {@link BrickletAnalogOut#setMode(short)}).
*/
public void setVoltage(int voltage) throws TinkerforgeException {
checkValidity();
ByteBuffer bb = ipcon.createRequestPacket((byte)10, FUNCTION_SET_VOLTAGE, this);
bb.putShort((short)voltage);
sendRequest(bb.array(), 0);
}
/**
* Returns the voltage as set by {@link BrickletAnalogOut#setVoltage(int)}.
*/
public int getVoltage() throws TinkerforgeException {
checkValidity();
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_VOLTAGE, this);
byte[] response = sendRequest(bb.array(), 10);
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
int voltage = IPConnectionBase.unsignedShort(bb.getShort());
return voltage;
}
/**
* Sets the mode of the analog value. Possible modes:
*
* * 0: Normal Mode (Analog value as set by {@link BrickletAnalogOut#setVoltage(int)} is applied)
* * 1: 1k Ohm resistor to ground
* * 2: 100k Ohm resistor to ground
* * 3: 500k Ohm resistor to ground
*
* Setting the mode to 0 will result in an output voltage of 0 V. You can jump
* to a higher output voltage directly by calling {@link BrickletAnalogOut#setVoltage(int)}.
*/
public void setMode(short mode) throws TinkerforgeException {
checkValidity();
ByteBuffer bb = ipcon.createRequestPacket((byte)9, FUNCTION_SET_MODE, this);
bb.put((byte)mode);
sendRequest(bb.array(), 0);
}
/**
* Returns the mode as set by {@link BrickletAnalogOut#setMode(short)}.
*/
public short getMode() throws TinkerforgeException {
checkValidity();
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_MODE, this);
byte[] response = sendRequest(bb.array(), 9);
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
short mode = IPConnectionBase.unsignedByte(bb.get());
return mode;
}
/**
* Returns the UID, the UID where the Bricklet is connected to,
* the position, the hardware and firmware version as well as the
* device identifier.
*
* The position can be 'a', 'b', 'c', 'd', 'e', 'f', 'g' or 'h' (Bricklet Port).
* The Raspberry Pi HAT (Zero) Brick is always at position 'i' and the Bricklet
* connected to an :ref:`Isolator Bricklet <isolator_bricklet>` is always as
* position 'z'.
*
* The device identifier numbers can be found :ref:`here <device_identifier>`.
* |device_identifier_constant|
*/
public Identity getIdentity() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_IDENTITY, this);
byte[] response = sendRequest(bb.array(), 33);
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
Identity obj = new Identity();
obj.uid = IPConnectionBase.string(bb, 8);
obj.connectedUid = IPConnectionBase.string(bb, 8);
obj.position = (char)(bb.get());
for (int i = 0; i < 3; i++) {
obj.hardwareVersion[i] = IPConnectionBase.unsignedByte(bb.get());
}
for (int i = 0; i < 3; i++) {
obj.firmwareVersion[i] = IPConnectionBase.unsignedByte(bb.get());
}
obj.deviceIdentifier = IPConnectionBase.unsignedShort(bb.getShort());
return obj;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy