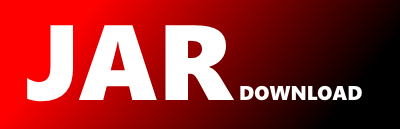
com.tinkerforge.BrickletCO2 Maven / Gradle / Ivy
/* ***********************************************************
* This file was automatically generated on 2020-06-25. *
* *
* Java Bindings Version 2.1.28 *
* *
* If you have a bugfix for this file and want to commit it, *
* please fix the bug in the generator. You can find a link *
* to the generators git repository on tinkerforge.com *
*************************************************************/
package com.tinkerforge;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.util.concurrent.CopyOnWriteArrayList;
import java.util.Arrays;
import java.util.List;
/**
* Measures CO2 concentration in ppm
*/
public class BrickletCO2 extends Device {
public final static int DEVICE_IDENTIFIER = 262;
public final static String DEVICE_DISPLAY_NAME = "CO2 Bricklet";
public final static byte FUNCTION_GET_CO2_CONCENTRATION = (byte)1;
public final static byte FUNCTION_SET_CO2_CONCENTRATION_CALLBACK_PERIOD = (byte)2;
public final static byte FUNCTION_GET_CO2_CONCENTRATION_CALLBACK_PERIOD = (byte)3;
public final static byte FUNCTION_SET_CO2_CONCENTRATION_CALLBACK_THRESHOLD = (byte)4;
public final static byte FUNCTION_GET_CO2_CONCENTRATION_CALLBACK_THRESHOLD = (byte)5;
public final static byte FUNCTION_SET_DEBOUNCE_PERIOD = (byte)6;
public final static byte FUNCTION_GET_DEBOUNCE_PERIOD = (byte)7;
public final static byte FUNCTION_GET_IDENTITY = (byte)255;
private final static int CALLBACK_CO2_CONCENTRATION = 8;
private final static int CALLBACK_CO2_CONCENTRATION_REACHED = 9;
public final static char THRESHOLD_OPTION_OFF = 'x';
public final static char THRESHOLD_OPTION_OUTSIDE = 'o';
public final static char THRESHOLD_OPTION_INSIDE = 'i';
public final static char THRESHOLD_OPTION_SMALLER = '<';
public final static char THRESHOLD_OPTION_GREATER = '>';
private List listenerCO2Concentration = new CopyOnWriteArrayList();
private List listenerCO2ConcentrationReached = new CopyOnWriteArrayList();
public class CO2ConcentrationCallbackThreshold {
public char option;
public int min;
public int max;
public String toString() {
return "[" + "option = " + option + ", " + "min = " + min + ", " + "max = " + max + "]";
}
}
/**
* This listener is triggered periodically with the period that is set by
* {@link BrickletCO2#setCO2ConcentrationCallbackPeriod(long)}. The parameter is the CO2
* concentration of the sensor.
*
* The {@link BrickletCO2.CO2ConcentrationListener} listener is only triggered if the CO2 concentration
* has changed since the last triggering.
*/
public interface CO2ConcentrationListener extends DeviceListener {
public void co2Concentration(int co2Concentration);
}
/**
* This listener is triggered when the threshold as set by
* {@link BrickletCO2#setCO2ConcentrationCallbackThreshold(char, int, int)} is reached.
* The parameter is the CO2 concentration.
*
* If the threshold keeps being reached, the listener is triggered periodically
* with the period as set by {@link BrickletCO2#setDebouncePeriod(long)}.
*/
public interface CO2ConcentrationReachedListener extends DeviceListener {
public void co2ConcentrationReached(int co2Concentration);
}
/**
* Creates an object with the unique device ID \c uid. and adds it to
* the IP Connection \c ipcon.
*/
public BrickletCO2(String uid, IPConnection ipcon) {
super(uid, ipcon);
apiVersion[0] = 2;
apiVersion[1] = 0;
apiVersion[2] = 0;
deviceIdentifier = DEVICE_IDENTIFIER;
deviceDisplayName = DEVICE_DISPLAY_NAME;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_GET_CO2_CONCENTRATION)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_SET_CO2_CONCENTRATION_CALLBACK_PERIOD)] = RESPONSE_EXPECTED_FLAG_TRUE;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_GET_CO2_CONCENTRATION_CALLBACK_PERIOD)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_SET_CO2_CONCENTRATION_CALLBACK_THRESHOLD)] = RESPONSE_EXPECTED_FLAG_TRUE;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_GET_CO2_CONCENTRATION_CALLBACK_THRESHOLD)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_SET_DEBOUNCE_PERIOD)] = RESPONSE_EXPECTED_FLAG_TRUE;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_GET_DEBOUNCE_PERIOD)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
responseExpected[IPConnectionBase.unsignedByte(FUNCTION_GET_IDENTITY)] = RESPONSE_EXPECTED_FLAG_ALWAYS_TRUE;
callbacks[CALLBACK_CO2_CONCENTRATION] = new IPConnection.DeviceCallbackListener() {
public void callback(byte[] packet) {
if (packet.length != 10) {
return; // silently ignoring callback with wrong length
}
ByteBuffer bb = ByteBuffer.wrap(packet, 8, packet.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
int co2Concentration = IPConnectionBase.unsignedShort(bb.getShort());
for (CO2ConcentrationListener listener: listenerCO2Concentration) {
listener.co2Concentration(co2Concentration);
}
}
};
callbacks[CALLBACK_CO2_CONCENTRATION_REACHED] = new IPConnection.DeviceCallbackListener() {
public void callback(byte[] packet) {
if (packet.length != 10) {
return; // silently ignoring callback with wrong length
}
ByteBuffer bb = ByteBuffer.wrap(packet, 8, packet.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
int co2Concentration = IPConnectionBase.unsignedShort(bb.getShort());
for (CO2ConcentrationReachedListener listener: listenerCO2ConcentrationReached) {
listener.co2ConcentrationReached(co2Concentration);
}
}
};
ipcon.addDevice(this);
}
/**
* Returns the measured CO2 concentration.
*
* If you want to get the CO2 concentration periodically, it is recommended to use
* the {@link BrickletCO2.CO2ConcentrationListener} listener and set the period with
* {@link BrickletCO2#setCO2ConcentrationCallbackPeriod(long)}.
*/
public int getCO2Concentration() throws TinkerforgeException {
checkValidity();
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_CO2_CONCENTRATION, this);
byte[] response = sendRequest(bb.array(), 10);
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
int co2Concentration = IPConnectionBase.unsignedShort(bb.getShort());
return co2Concentration;
}
/**
* Sets the period with which the {@link BrickletCO2.CO2ConcentrationListener} listener is
* triggered periodically. A value of 0 turns the listener off.
*
* The {@link BrickletCO2.CO2ConcentrationListener} listener is only triggered if the CO2 concentration
* has changed since the last triggering.
*/
public void setCO2ConcentrationCallbackPeriod(long period) throws TinkerforgeException {
checkValidity();
ByteBuffer bb = ipcon.createRequestPacket((byte)12, FUNCTION_SET_CO2_CONCENTRATION_CALLBACK_PERIOD, this);
bb.putInt((int)period);
sendRequest(bb.array(), 0);
}
/**
* Returns the period as set by {@link BrickletCO2#setCO2ConcentrationCallbackPeriod(long)}.
*/
public long getCO2ConcentrationCallbackPeriod() throws TinkerforgeException {
checkValidity();
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_CO2_CONCENTRATION_CALLBACK_PERIOD, this);
byte[] response = sendRequest(bb.array(), 12);
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
long period = IPConnectionBase.unsignedInt(bb.getInt());
return period;
}
/**
* Sets the thresholds for the {@link BrickletCO2.CO2ConcentrationReachedListener} listener.
*
* The following options are possible:
*
* \verbatim
* "Option", "Description"
*
* "'x'", "Listener is turned off"
* "'o'", "Listener is triggered when the CO2 concentration is *outside* the min and max values"
* "'i'", "Listener is triggered when the CO2 concentration is *inside* the min and max values"
* "'<'", "Listener is triggered when the CO2 concentration is smaller than the min value (max is ignored)"
* "'>'", "Listener is triggered when the CO2 concentration is greater than the min value (max is ignored)"
* \endverbatim
*/
public void setCO2ConcentrationCallbackThreshold(char option, int min, int max) throws TinkerforgeException {
checkValidity();
ByteBuffer bb = ipcon.createRequestPacket((byte)13, FUNCTION_SET_CO2_CONCENTRATION_CALLBACK_THRESHOLD, this);
bb.put((byte)option);
bb.putShort((short)min);
bb.putShort((short)max);
sendRequest(bb.array(), 0);
}
/**
* Returns the threshold as set by {@link BrickletCO2#setCO2ConcentrationCallbackThreshold(char, int, int)}.
*/
public CO2ConcentrationCallbackThreshold getCO2ConcentrationCallbackThreshold() throws TinkerforgeException {
checkValidity();
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_CO2_CONCENTRATION_CALLBACK_THRESHOLD, this);
byte[] response = sendRequest(bb.array(), 13);
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
CO2ConcentrationCallbackThreshold obj = new CO2ConcentrationCallbackThreshold();
obj.option = (char)(bb.get());
obj.min = IPConnectionBase.unsignedShort(bb.getShort());
obj.max = IPConnectionBase.unsignedShort(bb.getShort());
return obj;
}
/**
* Sets the period with which the threshold listeners
*
* * {@link BrickletCO2.CO2ConcentrationReachedListener},
*
* are triggered, if the thresholds
*
* * {@link BrickletCO2#setCO2ConcentrationCallbackThreshold(char, int, int)},
*
* keep being reached.
*/
public void setDebouncePeriod(long debounce) throws TinkerforgeException {
checkValidity();
ByteBuffer bb = ipcon.createRequestPacket((byte)12, FUNCTION_SET_DEBOUNCE_PERIOD, this);
bb.putInt((int)debounce);
sendRequest(bb.array(), 0);
}
/**
* Returns the debounce period as set by {@link BrickletCO2#setDebouncePeriod(long)}.
*/
public long getDebouncePeriod() throws TinkerforgeException {
checkValidity();
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_DEBOUNCE_PERIOD, this);
byte[] response = sendRequest(bb.array(), 12);
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
long debounce = IPConnectionBase.unsignedInt(bb.getInt());
return debounce;
}
/**
* Returns the UID, the UID where the Bricklet is connected to,
* the position, the hardware and firmware version as well as the
* device identifier.
*
* The position can be 'a', 'b', 'c', 'd', 'e', 'f', 'g' or 'h' (Bricklet Port).
* The Raspberry Pi HAT (Zero) Brick is always at position 'i' and the Bricklet
* connected to an :ref:`Isolator Bricklet <isolator_bricklet>` is always as
* position 'z'.
*
* The device identifier numbers can be found :ref:`here <device_identifier>`.
* |device_identifier_constant|
*/
public Identity getIdentity() throws TinkerforgeException {
ByteBuffer bb = ipcon.createRequestPacket((byte)8, FUNCTION_GET_IDENTITY, this);
byte[] response = sendRequest(bb.array(), 33);
bb = ByteBuffer.wrap(response, 8, response.length - 8);
bb.order(ByteOrder.LITTLE_ENDIAN);
Identity obj = new Identity();
obj.uid = IPConnectionBase.string(bb, 8);
obj.connectedUid = IPConnectionBase.string(bb, 8);
obj.position = (char)(bb.get());
for (int i = 0; i < 3; i++) {
obj.hardwareVersion[i] = IPConnectionBase.unsignedByte(bb.get());
}
for (int i = 0; i < 3; i++) {
obj.firmwareVersion[i] = IPConnectionBase.unsignedByte(bb.get());
}
obj.deviceIdentifier = IPConnectionBase.unsignedShort(bb.getShort());
return obj;
}
/**
* Adds a CO2Concentration listener.
*/
public void addCO2ConcentrationListener(CO2ConcentrationListener listener) {
listenerCO2Concentration.add(listener);
}
/**
* Removes a CO2Concentration listener.
*/
public void removeCO2ConcentrationListener(CO2ConcentrationListener listener) {
listenerCO2Concentration.remove(listener);
}
/**
* Adds a CO2ConcentrationReached listener.
*/
public void addCO2ConcentrationReachedListener(CO2ConcentrationReachedListener listener) {
listenerCO2ConcentrationReached.add(listener);
}
/**
* Removes a CO2ConcentrationReached listener.
*/
public void removeCO2ConcentrationReachedListener(CO2ConcentrationReachedListener listener) {
listenerCO2ConcentrationReached.remove(listener);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy