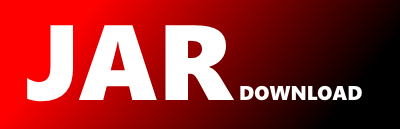
com.tinkerpop.blueprints.util.DefaultVertexQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blueprints-core Show documentation
Show all versions of blueprints-core Show documentation
Core interfaces and utilities for Blueprints
package com.tinkerpop.blueprints.util;
import com.tinkerpop.blueprints.Predicate;
import com.tinkerpop.blueprints.Direction;
import com.tinkerpop.blueprints.Edge;
import com.tinkerpop.blueprints.Element;
import com.tinkerpop.blueprints.Vertex;
import com.tinkerpop.blueprints.VertexQuery;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
/**
* For those graph engines that do not support the low-level querying of the edges of a vertex, then DefaultVertexQuery can be used.
* DefaultVertexQuery assumes, at minimum, that Vertex.getOutEdges() and Vertex.getInEdges() is implemented by the respective Vertex.
*
* @author Marko A. Rodriguez (http://markorodriguez.com)
*/
public class DefaultVertexQuery extends DefaultQuery implements VertexQuery {
protected final Vertex vertex;
public DefaultVertexQuery(final Vertex vertex) {
this.vertex = vertex;
}
public VertexQuery has(final String key) {
super.has(key);
return this;
}
public VertexQuery hasNot(final String key) {
super.hasNot(key);
return this;
}
public VertexQuery has(final String key, final Object value) {
super.has(key, value);
return this;
}
public VertexQuery hasNot(final String key, final Object value) {
super.hasNot(key, value);
return this;
}
public VertexQuery has(final String key, final Predicate predicate, final Object value) {
super.has(key, predicate, value);
return this;
}
public > VertexQuery has(final String key, final T value, final Compare compare) {
super.has(key, compare, value);
return this;
}
public > VertexQuery interval(final String key, final T startValue, final T endValue) {
super.interval(key, startValue, endValue);
return this;
}
public VertexQuery limit(final int limit) {
super.limit(limit);
return this;
}
public VertexQuery direction(final Direction direction) {
this.direction = direction;
return this;
}
public VertexQuery labels(final String... labels) {
this.labels = labels;
return this;
}
public Iterable edges() {
return new DefaultVertexQueryIterable(false);
}
public Iterable vertices() {
return new DefaultVertexQueryIterable(true);
}
public long count() {
long count = 0;
for (final Edge edge : this.edges()) {
count++;
}
return count;
}
public Object vertexIds() {
final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy