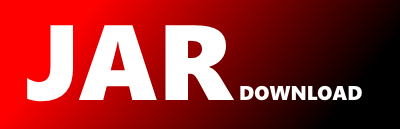
com.tinkerpop.blueprints.util.MultiIterable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of blueprints-core Show documentation
Show all versions of blueprints-core Show documentation
Core interfaces and utilities for Blueprints
The newest version!
package com.tinkerpop.blueprints.util;
import com.tinkerpop.blueprints.CloseableIterable;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
/**
* A helper class that is used to combine multiple iterables into a single closeable iterable.
*
* @author Marko A. Rodriguez (http://markorodriguez.com)
*/
public class MultiIterable implements CloseableIterable {
private final List> iterables;
public MultiIterable(final List> iterables) {
this.iterables = iterables;
}
public Iterator iterator() {
if (this.iterables.size() == 0) {
return (Iterator) Collections.emptyList().iterator();
} else {
return new Iterator() {
private Iterator currentIterator = iterables.get(0).iterator();
private int current = 0;
public void remove() {
currentIterator.remove();
}
public boolean hasNext() {
while (true) {
if (currentIterator.hasNext()) {
return true;
} else {
this.current++;
if (this.current >= iterables.size())
break;
this.currentIterator = iterables.get(this.current).iterator();
}
}
return false;
}
public S next() {
while (true) {
if (currentIterator.hasNext()) {
return currentIterator.next();
} else {
this.current++;
if (this.current >= iterables.size())
break;
this.currentIterator = iterables.get(current).iterator();
}
}
throw new NoSuchElementException();
}
};
}
}
@Override
public void close() {
for (Iterable itty : iterables) {
if (itty instanceof CloseableIterable) {
((CloseableIterable) itty).close();
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy