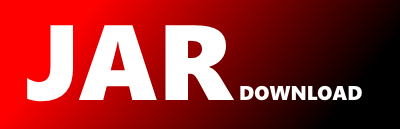
com.tinkerpop.rexster.client.RexsterClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rexster-protocol Show documentation
Show all versions of rexster-protocol Show documentation
RexPro is a binary protocol for Rexster graph server.
package com.tinkerpop.rexster.client;
import com.tinkerpop.rexster.protocol.BitWorks;
import com.tinkerpop.rexster.protocol.RexsterBindings;
import com.tinkerpop.rexster.protocol.msg.ErrorResponseMessage;
import com.tinkerpop.rexster.protocol.msg.MessageFlag;
import com.tinkerpop.rexster.protocol.msg.MsgPackScriptResponseMessage;
import com.tinkerpop.rexster.protocol.msg.RexProMessage;
import com.tinkerpop.rexster.protocol.msg.ScriptRequestMessage;
import org.apache.commons.configuration.Configuration;
import org.apache.log4j.Logger;
import org.glassfish.grizzly.Connection;
import org.glassfish.grizzly.GrizzlyFuture;
import org.glassfish.grizzly.nio.NIOConnection;
import org.glassfish.grizzly.nio.transport.TCPNIOTransport;
import org.msgpack.MessagePack;
import org.msgpack.template.Template;
import org.msgpack.type.Value;
import org.msgpack.unpacker.BufferUnpacker;
import org.msgpack.unpacker.Converter;
import org.msgpack.unpacker.UnpackerIterator;
import javax.script.Bindings;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import static org.msgpack.template.Templates.TString;
import static org.msgpack.template.Templates.TValue;
import static org.msgpack.template.Templates.tMap;
/**
* Basic client for sending Gremlin scripts to Rexster and receiving results as Map objects with String
* keys and MsgPack Value objects. This client is only for sessionless communication with Rexster and
* therefore all Gremlin scripts sent as requests to Rexster should be careful to handle their own
* transactional semantics. In other words, do not count on sending a script that mutates some aspect of the
* graph in one request and then a second request later to commit the transaction as there is no guarantee that
* the transaction will be handled properly.
*
* @author Stephen Mallette (http://stephen.genoprime.com)
*/
public class RexsterClient {
private static final Logger logger = Logger.getLogger(RexsterClient.class);
private final NIOConnection[] connections;
private int currentConnection = 0;
private final MessagePack msgpack = new MessagePack();
private final int timeoutConnection;
private final int timeoutWrite;
private final int timeoutRead;
private final int retries;
private final int waitBetweenRetries;
private final int asyncWriteQueueMaxBytes;
private final int arraySizeLimit;
private final int mapSizeLimit;
private final int rawSizeLimit;
private final String language;
private final TCPNIOTransport transport;
private final String[] hosts;
private final int port;
protected static ConcurrentHashMap> responses = new ConcurrentHashMap>();
protected RexsterClient(final Configuration configuration, final TCPNIOTransport transport) {
this.timeoutConnection = configuration.getInt(RexsterClientTokens.CONFIG_TIMEOUT_CONNECTION_MS);
this.timeoutRead = configuration.getInt(RexsterClientTokens.CONFIG_TIMEOUT_READ_MS);
this.timeoutWrite = configuration.getInt(RexsterClientTokens.CONFIG_TIMEOUT_WRITE_MS);
this.retries = configuration.getInt(RexsterClientTokens.CONFIG_MESSAGE_RETRY_COUNT);
this.waitBetweenRetries = configuration.getInt(RexsterClientTokens.CONFIG_MESSAGE_RETRY_WAIT_MS);
this.asyncWriteQueueMaxBytes = configuration.getInt(RexsterClientTokens.CONFIG_MAX_ASYNC_WRITE_QUEUE_BYTES);
this.arraySizeLimit = configuration.getInt(RexsterClientTokens.CONFIG_DESERIALIZE_ARRAY_SIZE_LIMIT);
this.mapSizeLimit = configuration.getInt(RexsterClientTokens.CONFIG_DESERIALIZE_MAP_SIZE_LIMIT);
this.rawSizeLimit = configuration.getInt(RexsterClientTokens.CONFIG_DESERIALIZE_RAW_SIZE_LIMIT);
this.language = configuration.getString(RexsterClientTokens.CONFIG_LANGUAGE);
this.transport = transport;
this.port = configuration.getInt(RexsterClientTokens.CONFIG_PORT);
final String hostname = configuration.getString(RexsterClientTokens.CONFIG_HOSTNAME);
this.hosts = hostname.split(",");
this.connections = new NIOConnection[this.hosts.length];
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy