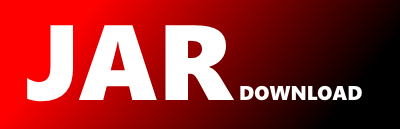
com.tinkerpop.gremlin.structure.FeatureSupportTest Maven / Gradle / Ivy
The newest version!
package com.tinkerpop.gremlin.structure;
import com.tinkerpop.gremlin.AbstractGremlinTest;
import com.tinkerpop.gremlin.ExceptionCoverage;
import com.tinkerpop.gremlin.FeatureRequirement;
import com.tinkerpop.gremlin.FeatureRequirementSet;
import com.tinkerpop.gremlin.GraphManager;
import com.tinkerpop.gremlin.process.T;
import com.tinkerpop.gremlin.structure.Graph.Features.EdgeFeatures;
import com.tinkerpop.gremlin.structure.Graph.Features.EdgePropertyFeatures;
import com.tinkerpop.gremlin.structure.Graph.Features.GraphFeatures;
import com.tinkerpop.gremlin.structure.Graph.Features.VertexFeatures;
import com.tinkerpop.gremlin.structure.Graph.Features.VertexPropertyFeatures;
import com.tinkerpop.gremlin.util.StreamFactory;
import org.junit.Before;
import org.junit.Test;
import org.junit.experimental.runners.Enclosed;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import java.util.Date;
import java.util.UUID;
import static com.tinkerpop.gremlin.structure.Graph.Features.ElementFeatures.*;
import static com.tinkerpop.gremlin.structure.Graph.Features.GraphFeatures.*;
import static com.tinkerpop.gremlin.structure.Graph.Features.VariableFeatures.FEATURE_VARIABLES;
import static com.tinkerpop.gremlin.structure.Graph.Features.VertexFeatures.FEATURE_USER_SUPPLIED_IDS;
import static org.hamcrest.CoreMatchers.is;
import static org.junit.Assert.*;
import static org.junit.Assume.assumeThat;
/**
* Tests that do basic validation of proper Feature settings in Graph implementations.
*
* @author Stephen Mallette (http://stephen.genoprime.com)
*/
@RunWith(Enclosed.class)
@SuppressWarnings("ThrowableResultOfMethodCallIgnored")
public class FeatureSupportTest {
private static final String INVALID_FEATURE_SPECIFICATION = "Features for %s specify that %s is false, but the feature appears to be implemented. Reconsider this setting or throw the standard Exception.";
public static class FeatureToStringTest extends AbstractGremlinTest {
/**
* A rough test to ensure that StringFactory is being used to toString Features.
*/
@Test
public void shouldHaveStandardToStringRepresentation() {
assertTrue(g.features().toString().startsWith("FEATURES"));
}
}
/**
* Feature checks that test {@link com.tinkerpop.gremlin.structure.Graph} functionality to determine if a feature should be on when it is marked
* as not supported.
*/
@ExceptionCoverage(exceptionClass = Graph.Exceptions.class, methods = {
"variablesNotSupported",
"graphComputerNotSupported",
"transactionsNotSupported"
})
public static class GraphFunctionalityTest extends AbstractGremlinTest {
/**
* This isn't really a test. It just pretty prints the features for the graph for reference. Of course,
* if the implementing classes use anonymous inner classes it will
*/
@Test
public void shouldPrintTheFeatureList() {
System.out.println(String.format("Printing Features of %s for reference: ", g.getClass().getSimpleName()));
System.out.println(g.features());
assertTrue(true);
}
/**
* A {@link com.tinkerpop.gremlin.structure.Graph} that does not support {@link com.tinkerpop.gremlin.structure.Graph.Features.GraphFeatures#FEATURE_COMPUTER} must call
* {@link com.tinkerpop.gremlin.structure.Graph.Exceptions#graphComputerNotSupported()}.
*/
@Test
@FeatureRequirement(featureClass = GraphFeatures.class, feature = FEATURE_COMPUTER, supported = false)
public void shouldSupportComputerIfAGraphCanCompute() throws Exception {
try {
g.compute();
fail(String.format(INVALID_FEATURE_SPECIFICATION, GraphFeatures.class.getSimpleName(), FEATURE_COMPUTER));
} catch (Exception e) {
validateException(Graph.Exceptions.graphComputerNotSupported(), e);
}
}
/**
* A {@link com.tinkerpop.gremlin.structure.Graph} that does not support {@link com.tinkerpop.gremlin.structure.Graph.Features.GraphFeatures#FEATURE_TRANSACTIONS} must call
* {@link com.tinkerpop.gremlin.structure.Graph.Exceptions#transactionsNotSupported()}.
*/
@Test
@FeatureRequirement(featureClass = GraphFeatures.class, feature = FEATURE_TRANSACTIONS, supported = false)
public void shouldSupportTransactionsIfAGraphConstructsATx() throws Exception {
try {
g.tx();
fail(String.format(INVALID_FEATURE_SPECIFICATION, GraphFeatures.class.getSimpleName(), FEATURE_TRANSACTIONS));
} catch (Exception e) {
validateException(Graph.Exceptions.transactionsNotSupported(), e);
}
}
/**
* A {@link com.tinkerpop.gremlin.structure.Graph} that does not support {@link com.tinkerpop.gremlin.structure.Graph.Features.VariableFeatures#FEATURE_VARIABLES} must call
* {@link com.tinkerpop.gremlin.structure.Graph.Exceptions#variablesNotSupported()}.
*/
@Test
@FeatureRequirement(featureClass = Graph.Features.VariableFeatures.class, feature = FEATURE_VARIABLES, supported = false)
public void shouldSupportMemoryIfAGraphAcceptsMemory() throws Exception {
try {
g.variables();
fail(String.format(INVALID_FEATURE_SPECIFICATION, Graph.Features.VariableFeatures.class.getSimpleName(), FEATURE_VARIABLES));
} catch (Exception e) {
validateException(Graph.Exceptions.variablesNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.GraphFeatures.class, feature = FEATURE_TRANSACTIONS)
@FeatureRequirement(featureClass = Graph.Features.GraphFeatures.class, feature = FEATURE_THREADED_TRANSACTIONS, supported = false)
public void shouldThrowOnThreadedTransactionNotSupported() {
try {
g.tx().create();
fail("An exception should be thrown since the threaded transaction feature is not supported");
} catch (Exception e) {
validateException(Transaction.Exceptions.threadedTransactionsNotSupported(), e);
}
}
}
/**
* Feature checks that test {@link com.tinkerpop.gremlin.structure.Vertex} functionality to determine if a feature
* should be on when it is marked as not supported.
*/
@ExceptionCoverage(exceptionClass = Vertex.Exceptions.class, methods = {
"userSuppliedIdsNotSupported",
"userSuppliedIdsOfThisTypeNotSupported",
"vertexRemovalNotSupported"
})
@ExceptionCoverage(exceptionClass = Graph.Exceptions.class, methods = {
"vertexAdditionsNotSupported"
})
@ExceptionCoverage(exceptionClass = Element.Exceptions.class, methods = {
"propertyAdditionNotSupported",
"propertyRemovalNotSupported"
})
public static class VertexFunctionalityTest extends AbstractGremlinTest {
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES, supported = false)
public void shouldSupportAddVerticesIfAVertexCanBeAdded() throws Exception {
try {
g.addVertex();
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), VertexFeatures.FEATURE_ADD_VERTICES));
} catch (Exception e) {
validateException(Graph.Exceptions.vertexAdditionsNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS, supported = false)
public void shouldSupportUserSuppliedIdsIfAnIdCanBeAssignedToVertex() throws Exception {
try {
g.addVertex(T.id, GraphManager.get().convertId(99999943835l));
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), FEATURE_USER_SUPPLIED_IDS));
} catch (Exception e) {
validateException(Vertex.Exceptions.userSuppliedIdsNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_STRING_IDS, supported = false)
public void shouldSupportUserSuppliedIdsOfTypeString() throws Exception {
try {
g.addVertex(T.id, "this-is-a-valid-id");
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), FEATURE_STRING_IDS));
} catch (Exception e) {
validateException(Vertex.Exceptions.userSuppliedIdsOfThisTypeNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_NUMERIC_IDS, supported = false)
public void shouldSupportUserSuppliedIdsOfTypeNumeric() throws Exception {
try {
g.addVertex(T.id, 123456);
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), FEATURE_NUMERIC_IDS));
} catch (Exception e) {
validateException(Vertex.Exceptions.userSuppliedIdsOfThisTypeNotSupported(), e);
}
try {
g.addVertex(T.id, 123456l);
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), FEATURE_NUMERIC_IDS));
} catch (Exception e) {
validateException(Vertex.Exceptions.userSuppliedIdsOfThisTypeNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_UUID_IDS, supported = false)
public void shouldSupportUserSuppliedIdsOfTypeUuid() throws Exception {
try {
g.addVertex(T.id, UUID.randomUUID());
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), FEATURE_UUID_IDS));
} catch (Exception e) {
validateException(Vertex.Exceptions.userSuppliedIdsOfThisTypeNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_ANY_IDS, supported = false)
public void shouldSupportUserSuppliedIdsOfTypeAny() throws Exception {
try {
g.addVertex(T.id, new Date());
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), FEATURE_ANY_IDS));
} catch (Exception e) {
validateException(Vertex.Exceptions.userSuppliedIdsOfThisTypeNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS, supported = false)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_STRING_IDS, supported = false)
public void shouldSupportStringIdsIfStringIdsAreGeneratedFromTheGraph() throws Exception {
final Vertex v = g.addVertex();
if (v.id() instanceof String)
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), FEATURE_STRING_IDS));
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS, supported = false)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_UUID_IDS, supported = false)
public void shouldSupportStringIdsIfUuidIdsAreGeneratedFromTheGraph() throws Exception {
final Vertex v = g.addVertex();
if (v.id() instanceof UUID)
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), FEATURE_UUID_IDS));
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS, supported = false)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_NUMERIC_IDS, supported = false)
public void shouldSupportStringIdsIfNumericIdsAreGeneratedFromTheGraph() throws Exception {
final Vertex v = g.addVertex();
if (v.id() instanceof Number)
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), FEATURE_NUMERIC_IDS));
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = VertexFeatures.FEATURE_ADD_PROPERTY, supported = false)
public void shouldSupportAddVertexPropertyIfItCanBeAdded() throws Exception {
try {
final Vertex v = g.addVertex();
v.property("should", "not-add-property");
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), VertexFeatures.FEATURE_ADD_PROPERTY));
} catch (Exception e) {
validateException(Element.Exceptions.propertyAdditionNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_REMOVE_VERTICES, supported = false)
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
public void shouldSupportRemoveVerticesIfAVertexCanBeRemoved() throws Exception {
try {
g.addVertex().remove();
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), VertexFeatures.FEATURE_REMOVE_VERTICES));
} catch (Exception e) {
validateException(Vertex.Exceptions.vertexRemovalNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = VertexFeatures.FEATURE_REMOVE_PROPERTY, supported = false)
@FeatureRequirementSet(FeatureRequirementSet.Package.VERTICES_ONLY)
public void shouldSupportRemovePropertyIfAPropertyCanBeRemoved() throws Exception {
try {
final Vertex v = g.addVertex("name", "me");
v.property("name").remove();
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), VertexFeatures.FEATURE_REMOVE_PROPERTY));
} catch (Exception e) {
validateException(Element.Exceptions.propertyRemovalNotSupported(), e);
}
}
}
/**
* Feature checks that test {@link com.tinkerpop.gremlin.structure.Edge} functionality to determine if a feature
* should be on when it is marked as not supported.
*/
@ExceptionCoverage(exceptionClass = Vertex.Exceptions.class, methods = {
"edgeAdditionsNotSupported"
})
@ExceptionCoverage(exceptionClass = Edge.Exceptions.class, methods = {
"edgeRemovalNotSupported",
"userSuppliedIdsNotSupported",
"userSuppliedIdsOfThisTypeNotSupported"
})
@ExceptionCoverage(exceptionClass = Element.Exceptions.class, methods = {
"propertyAdditionNotSupported",
"propertyRemovalNotSupported"
})
public static class EdgeFunctionalityTest extends AbstractGremlinTest {
@Test
@FeatureRequirement(featureClass = Graph.Features.EdgeFeatures.class, feature = Graph.Features.EdgeFeatures.FEATURE_ADD_EDGES, supported = false)
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
public void shouldSupportAddEdgesIfEdgeCanBeAdded() throws Exception {
try {
final Vertex v = g.addVertex();
v.addEdge("friend", v);
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), EdgeFeatures.FEATURE_ADD_EDGES));
} catch (Exception e) {
validateException(Vertex.Exceptions.edgeAdditionsNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.EdgeFeatures.class, feature = Graph.Features.EdgeFeatures.FEATURE_ADD_EDGES)
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = EdgeFeatures.class, feature = EdgeFeatures.FEATURE_USER_SUPPLIED_IDS, supported = false)
public void shouldSupportUserSuppliedIdsIfAnIdCanBeAssignedToEdge() throws Exception {
try {
final Vertex v = g.addVertex();
v.addEdge("friend", v, T.id, GraphManager.get().convertId(99999943835l));
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), EdgeFeatures.FEATURE_USER_SUPPLIED_IDS));
} catch (Exception e) {
validateException(Edge.Exceptions.userSuppliedIdsNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.EdgeFeatures.class, feature = Graph.Features.EdgeFeatures.FEATURE_ADD_EDGES)
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = EdgeFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS, supported = false)
@FeatureRequirement(featureClass = EdgeFeatures.class, feature = FEATURE_STRING_IDS, supported = false)
public void shouldSupportStringIdsIfStringIdsAreGeneratedFromTheGraph() throws Exception {
final Vertex v = g.addVertex();
final Edge e = v.addEdge("knows", v);
if (e.id() instanceof String)
fail(String.format(INVALID_FEATURE_SPECIFICATION, EdgeFeatures.class.getSimpleName(), FEATURE_STRING_IDS));
}
@Test
@FeatureRequirement(featureClass = Graph.Features.EdgeFeatures.class, feature = Graph.Features.EdgeFeatures.FEATURE_ADD_EDGES)
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = EdgeFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS, supported = false)
@FeatureRequirement(featureClass = EdgeFeatures.class, feature = FEATURE_UUID_IDS, supported = false)
public void shouldSupportStringIdsIfUuidIdsAreGeneratedFromTheGraph() throws Exception {
final Vertex v = g.addVertex();
final Edge e = v.addEdge("knows", v);
if (e.id() instanceof UUID)
fail(String.format(INVALID_FEATURE_SPECIFICATION, EdgeFeatures.class.getSimpleName(), FEATURE_UUID_IDS));
}
@Test
@FeatureRequirement(featureClass = Graph.Features.EdgeFeatures.class, feature = Graph.Features.EdgeFeatures.FEATURE_ADD_EDGES)
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = EdgeFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS, supported = false)
@FeatureRequirement(featureClass = EdgeFeatures.class, feature = FEATURE_NUMERIC_IDS, supported = false)
public void shouldSupportStringIdsIfNumericIdsAreGeneratedFromTheGraph() throws Exception {
final Vertex v = g.addVertex();
final Edge e = v.addEdge("knows", v);
if (e.id() instanceof Number)
fail(String.format(INVALID_FEATURE_SPECIFICATION, EdgeFeatures.class.getSimpleName(), FEATURE_NUMERIC_IDS));
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_STRING_IDS, supported = false)
public void shouldSupportUserSuppliedIdsOfTypeString() throws Exception {
try {
final Vertex v = g.addVertex();
v.addEdge("test", v, T.id, "this-is-a-valid-id");
fail(String.format(INVALID_FEATURE_SPECIFICATION, EdgeFeatures.class.getSimpleName(), FEATURE_STRING_IDS));
} catch (Exception e) {
validateException(Edge.Exceptions.userSuppliedIdsOfThisTypeNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_NUMERIC_IDS, supported = false)
public void shouldSupportUserSuppliedIdsOfTypeNumeric() throws Exception {
try {
final Vertex v = g.addVertex();
v.addEdge("test", v, T.id, 123456);
fail(String.format(INVALID_FEATURE_SPECIFICATION, EdgeFeatures.class.getSimpleName(), FEATURE_NUMERIC_IDS));
} catch (Exception e) {
validateException(Edge.Exceptions.userSuppliedIdsOfThisTypeNotSupported(), e);
}
try {
final Vertex v = g.addVertex();
v.addEdge("test", v, T.id, 123456l);
fail(String.format(INVALID_FEATURE_SPECIFICATION, EdgeFeatures.class.getSimpleName(), FEATURE_NUMERIC_IDS));
} catch (Exception e) {
validateException(Edge.Exceptions.userSuppliedIdsOfThisTypeNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_UUID_IDS, supported = false)
public void shouldSupportUserSuppliedIdsOfTypeUuid() throws Exception {
try {
final Vertex v = g.addVertex();
v.addEdge("test", v, T.id, UUID.randomUUID());
fail(String.format(INVALID_FEATURE_SPECIFICATION, EdgeFeatures.class.getSimpleName(), FEATURE_ANY_IDS));
} catch (Exception e) {
validateException(Edge.Exceptions.userSuppliedIdsOfThisTypeNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = Graph.Features.EdgeFeatures.class, feature = Graph.Features.EdgeFeatures.FEATURE_ADD_EDGES)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_USER_SUPPLIED_IDS)
@FeatureRequirement(featureClass = VertexFeatures.class, feature = FEATURE_ANY_IDS, supported = false)
public void shouldSupportUserSuppliedIdsOfTypeAny() throws Exception {
try {
final Vertex v = g.addVertex();
v.addEdge("test", v, T.id, new Date());
fail(String.format(INVALID_FEATURE_SPECIFICATION, EdgeFeatures.class.getSimpleName(), FEATURE_ANY_IDS));
} catch (Exception e) {
validateException(Edge.Exceptions.userSuppliedIdsOfThisTypeNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
@FeatureRequirement(featureClass = Graph.Features.EdgeFeatures.class, feature = Graph.Features.EdgeFeatures.FEATURE_ADD_EDGES)
@FeatureRequirement(featureClass = Graph.Features.EdgeFeatures.class, feature = EdgeFeatures.FEATURE_ADD_PROPERTY, supported = false)
public void shouldSupportAddVertexPropertyIfItCanBeAdded() throws Exception {
try {
final Vertex v = g.addVertex();
final Edge e = v.addEdge("test", v);
e.property("should", "not-add-property");
fail(String.format(INVALID_FEATURE_SPECIFICATION, EdgePropertyFeatures.class.getSimpleName(), EdgeFeatures.FEATURE_ADD_PROPERTY));
} catch (Exception e) {
validateException(Element.Exceptions.propertyAdditionNotSupported(), e);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.EdgeFeatures.class, feature = Graph.Features.EdgeFeatures.FEATURE_ADD_EDGES)
@FeatureRequirement(featureClass = Graph.Features.EdgeFeatures.class, feature = Graph.Features.EdgeFeatures.FEATURE_REMOVE_EDGES, supported = false)
@FeatureRequirement(featureClass = Graph.Features.VertexFeatures.class, feature = Graph.Features.VertexFeatures.FEATURE_ADD_VERTICES)
public void shouldSupportRemoveEdgesIfEdgeCanBeRemoved() throws Exception {
try {
final Vertex v = g.addVertex();
v.addEdge("friend", v);
v.remove();
fail(String.format(INVALID_FEATURE_SPECIFICATION, VertexFeatures.class.getSimpleName(), EdgeFeatures.FEATURE_REMOVE_EDGES));
} catch (Exception ex) {
validateException(Edge.Exceptions.edgeRemovalNotSupported(), ex);
}
}
@Test
@FeatureRequirement(featureClass = Graph.Features.EdgeFeatures.class, feature = EdgeFeatures.FEATURE_REMOVE_PROPERTY, supported = false)
@FeatureRequirementSet(FeatureRequirementSet.Package.SIMPLE)
public void shouldSupportRemovePropertyIfAPropertyCanBeRemoved() throws Exception {
try {
final Vertex v = g.addVertex();
final Edge e = v.addEdge("label", v);
e.property("name").remove();
fail(String.format(INVALID_FEATURE_SPECIFICATION, EdgeFeatures.class.getSimpleName(), EdgeFeatures.FEATURE_REMOVE_PROPERTY));
} catch (Exception ex) {
validateException(Element.Exceptions.propertyRemovalNotSupported(), ex);
}
}
}
/**
* Feature checks that test {@link com.tinkerpop.gremlin.structure.Element} {@link com.tinkerpop.gremlin.structure.Property} functionality to determine if a feature should be on
* when it is marked as not supported.
*/
@RunWith(Parameterized.class)
@ExceptionCoverage(exceptionClass = Property.Exceptions.class, methods = {
"dataTypeOfPropertyValueNotSupported"
})
public static class ElementPropertyDataTypeFunctionalityTest extends AbstractGremlinTest {
private static final String INVALID_FEATURE_SPECIFICATION = "Features for %s specify that %s is false, but the feature appears to be implemented. Reconsider this setting or throw the standard Exception.";
@Parameterized.Parameters(name = "supports{0}({1})")
public static Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy