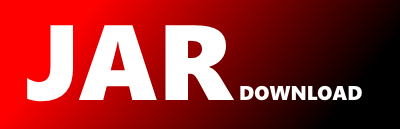
com.tinkerpop.pipes.transform.SelectPipe Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pipes Show documentation
Show all versions of pipes Show documentation
Pipes is a dataflow framework written in Java that enables the splitting, merging, filtering, and
transformation of data from input to output.
Computations are expressed using a combinator model and are evaluated in a memory-efficient, lazy fashion.
package com.tinkerpop.pipes.transform;
import com.tinkerpop.pipes.AbstractPipe;
import com.tinkerpop.pipes.PipeFunction;
import com.tinkerpop.pipes.util.AsPipe;
import com.tinkerpop.pipes.util.PipeHelper;
import com.tinkerpop.pipes.util.structures.Row;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
/**
* SelectPipe will emit a List of the objects that are at the respective 'as'-steps (i.e. partition steps) in the Pipeline.
* In this way, it is possible to isolate particular parts of the Pipeline for emission.
* If stepNames are provided the only those partition steps are emitted.
* If stepFunctions are provided then each object of the step is processed by the function prior to emission.
*
* @author Marko A. Rodriguez (http://markorodriguez.com)
*/
public class SelectPipe extends AbstractPipe implements TransformPipe {
private final PipeFunction[] stepFunctions;
private final boolean doFunctions;
private int currentFunction;
private final List asPipes = new ArrayList();
private final List columnNames;
private final Collection stepNames;
public SelectPipe(final Collection stepNames, final List allPreviousAsPipes, final PipeFunction... stepFunctions) {
this.stepFunctions = stepFunctions;
this.stepNames = stepNames;
if (this.doFunctions = this.stepFunctions.length > 0)
currentFunction = 0;
final List tempNames = new ArrayList();
for (final AsPipe asPipe : allPreviousAsPipes) {
final String columnName = asPipe.getName();
if (null == this.stepNames || this.stepNames.contains(columnName)) {
tempNames.add(columnName);
this.asPipes.add(asPipe);
}
}
if (tempNames.size() > 0)
this.columnNames = tempNames;
else
this.columnNames = null;
}
public Row processNextStart() {
this.starts.next();
final Row row;
if (null == columnNames)
row = new Row();
else
row = new Row(columnNames);
for (final AsPipe asPipe : this.asPipes) {
if (doFunctions) {
row.add(this.stepFunctions[currentFunction++ % stepFunctions.length].compute(asPipe.getCurrentEnd()));
} else {
row.add(asPipe.getCurrentEnd());
}
}
return row;
}
public void reset() {
this.currentFunction = 0;
super.reset();
}
public String toString() {
if (null == this.stepNames)
return PipeHelper.makePipeString(this);
else
return PipeHelper.makePipeString(this, this.stepNames.toArray());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy