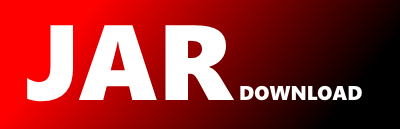
com.tngtech.jgiven.integration.spring.SpringStepMethodInterceptor Maven / Gradle / Ivy
Show all versions of jgiven-spring Show documentation
package com.tngtech.jgiven.integration.spring;
import java.lang.reflect.Method;
import org.aopalliance.intercept.MethodInterceptor;
import org.aopalliance.intercept.MethodInvocation;
import com.tngtech.jgiven.impl.intercept.StepMethodInterceptor;
/**
* StepMethodInterceptor that uses {@link MethodInterceptor} for intercepting JGiven methods
* See below on how to configure this bean.
*
*
* Sample Configuration:
*
* {@literal @}Bean
* {@literal @}Scope("prototype")
* public SpringStepMethodInterceptor springStepMethodInterceptor() {
* return new SpringStepMethodInterceptor();
* }
*
*
* The StepMethodInterceptor is stateful, and thus should use "prototype" scope
* @since 0.8.0
*/
public class SpringStepMethodInterceptor extends StepMethodInterceptor implements MethodInterceptor {
public SpringStepMethodInterceptor() {
super(null, null);
}
@Override
public Object invoke(final MethodInvocation invocation) throws Throwable {
Object receiver = invocation.getThis();
Method method = invocation.getMethod();
Object[] parameters = invocation.getArguments();
Invoker invoker = new Invoker() {
@Override
public Object proceed() throws Throwable {
return invocation.proceed();
}
};
if (getScenarioMethodHandler() == null || getStackDepth() == null) {
return invoker.proceed(); // not running in JGiven context
}
return doIntercept(receiver, method, parameters, invoker);
}
}