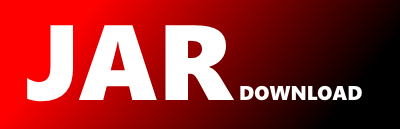
commonMain.protokt.v1.google.cloud.location.locations.kt Maven / Gradle / Ivy
// Generated by protokt version 1.0.0-beta.2. Do not modify.
// Source: google/cloud/location/locations.proto
@file:Suppress("DEPRECATION")
package protokt.v1.google.cloud.location
import protokt.v1.AbstractDeserializer
import protokt.v1.AbstractMessage
import protokt.v1.BuilderDsl
import protokt.v1.Collections.copyList
import protokt.v1.Collections.copyMap
import protokt.v1.Collections.unmodifiableList
import protokt.v1.Collections.unmodifiableMap
import protokt.v1.GeneratedMessage
import protokt.v1.GeneratedProperty
import protokt.v1.Reader
import protokt.v1.SizeCodecs.sizeOf
import protokt.v1.UnknownFieldSet
import protokt.v1.Writer
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.collections.MutableList
import kotlin.collections.MutableMap
import kotlin.jvm.JvmStatic
import kotlin.Any as KotlinAny
import protokt.v1.google.protobuf.Any as ProtobufAny
/**
* The request message for [Locations.ListLocations][google.cloud.location.Locations.ListLocations].
*/
@GeneratedMessage("google.cloud.location.ListLocationsRequest")
public class ListLocationsRequest private constructor(
/**
* The resource that owns the locations collection, if applicable.
*/
@GeneratedProperty(1)
public val name: String,
/**
* The standard list filter.
*/
@GeneratedProperty(2)
public val filter: String,
/**
* The standard list page size.
*/
@GeneratedProperty(3)
public val pageSize: Int,
/**
* The standard list page token.
*/
@GeneratedProperty(4)
public val pageToken: String,
public val unknownFields: UnknownFieldSet = UnknownFieldSet.empty()
) : AbstractMessage() {
private val `$messageSize`: Int by lazy {
var result = 0
if (name.isNotEmpty()) {
result += sizeOf(10u) + sizeOf(name)
}
if (filter.isNotEmpty()) {
result += sizeOf(18u) + sizeOf(filter)
}
if (pageSize != 0) {
result += sizeOf(24u) + sizeOf(pageSize)
}
if (pageToken.isNotEmpty()) {
result += sizeOf(34u) + sizeOf(pageToken)
}
result += unknownFields.size()
result
}
override fun messageSize(): Int = `$messageSize`
override fun serialize(writer: Writer) {
if (name.isNotEmpty()) {
writer.writeTag(10u).write(name)
}
if (filter.isNotEmpty()) {
writer.writeTag(18u).write(filter)
}
if (pageSize != 0) {
writer.writeTag(24u).write(pageSize)
}
if (pageToken.isNotEmpty()) {
writer.writeTag(34u).write(pageToken)
}
writer.writeUnknown(unknownFields)
}
override fun equals(other: KotlinAny?): Boolean =
other is ListLocationsRequest &&
other.name == name &&
other.filter == filter &&
other.pageSize == pageSize &&
other.pageToken == pageToken &&
other.unknownFields == unknownFields
override fun hashCode(): Int {
var result = unknownFields.hashCode()
result = 31 * result + name.hashCode()
result = 31 * result + filter.hashCode()
result = 31 * result + pageSize.hashCode()
result = 31 * result + pageToken.hashCode()
return result
}
override fun toString(): String =
"ListLocationsRequest(" +
"name=$name, " +
"filter=$filter, " +
"pageSize=$pageSize, " +
"pageToken=$pageToken" +
if (unknownFields.isEmpty()) ")" else ", unknownFields=$unknownFields)"
public fun copy(builder: Builder.() -> Unit): ListLocationsRequest =
Builder().apply {
name = [email protected]
filter = [email protected]
pageSize = [email protected]
pageToken = [email protected]
unknownFields = [email protected]
builder()
}.build()
@BuilderDsl
public class Builder {
public var name: String = ""
public var filter: String = ""
public var pageSize: Int = 0
public var pageToken: String = ""
public var unknownFields: UnknownFieldSet = UnknownFieldSet.empty()
public fun build(): ListLocationsRequest =
ListLocationsRequest(
name,
filter,
pageSize,
pageToken,
unknownFields
)
}
public companion object Deserializer : AbstractDeserializer() {
@JvmStatic
override fun deserialize(reader: Reader): ListLocationsRequest {
var name = ""
var filter = ""
var pageSize = 0
var pageToken = ""
var unknownFields: UnknownFieldSet.Builder? = null
while (true) {
when (reader.readTag()) {
0u -> return ListLocationsRequest(
name,
filter,
pageSize,
pageToken,
UnknownFieldSet.from(unknownFields)
)
10u -> name = reader.readString()
18u -> filter = reader.readString()
24u -> pageSize = reader.readInt32()
34u -> pageToken = reader.readString()
else ->
unknownFields =
(unknownFields ?: UnknownFieldSet.Builder()).also {
it.add(reader.readUnknown())
}
}
}
}
@JvmStatic
public operator fun invoke(dsl: Builder.() -> Unit): ListLocationsRequest = Builder().apply(dsl).build()
}
}
/**
* The response message for
* [Locations.ListLocations][google.cloud.location.Locations.ListLocations].
*/
@GeneratedMessage("google.cloud.location.ListLocationsResponse")
public class ListLocationsResponse private constructor(
/**
* A list of locations that matches the specified filter in the request.
*/
@GeneratedProperty(1)
public val locations: List,
/**
* The standard List next-page token.
*/
@GeneratedProperty(2)
public val nextPageToken: String,
public val unknownFields: UnknownFieldSet = UnknownFieldSet.empty()
) : AbstractMessage() {
private val `$messageSize`: Int by lazy {
var result = 0
if (locations.isNotEmpty()) {
result += (sizeOf(10u) * locations.size) + locations.sumOf { sizeOf(it) }
}
if (nextPageToken.isNotEmpty()) {
result += sizeOf(18u) + sizeOf(nextPageToken)
}
result += unknownFields.size()
result
}
override fun messageSize(): Int = `$messageSize`
override fun serialize(writer: Writer) {
locations.forEach { writer.writeTag(10u).write(it) }
if (nextPageToken.isNotEmpty()) {
writer.writeTag(18u).write(nextPageToken)
}
writer.writeUnknown(unknownFields)
}
override fun equals(other: KotlinAny?): Boolean =
other is ListLocationsResponse &&
other.locations == locations &&
other.nextPageToken == nextPageToken &&
other.unknownFields == unknownFields
override fun hashCode(): Int {
var result = unknownFields.hashCode()
result = 31 * result + locations.hashCode()
result = 31 * result + nextPageToken.hashCode()
return result
}
override fun toString(): String =
"ListLocationsResponse(" +
"locations=$locations, " +
"nextPageToken=$nextPageToken" +
if (unknownFields.isEmpty()) ")" else ", unknownFields=$unknownFields)"
public fun copy(builder: Builder.() -> Unit): ListLocationsResponse =
Builder().apply {
locations = [email protected]
nextPageToken = [email protected]
unknownFields = [email protected]
builder()
}.build()
@BuilderDsl
public class Builder {
public var locations: List = emptyList()
set(newValue) {
field = copyList(newValue)
}
public var nextPageToken: String = ""
public var unknownFields: UnknownFieldSet = UnknownFieldSet.empty()
public fun build(): ListLocationsResponse =
ListLocationsResponse(
unmodifiableList(locations),
nextPageToken,
unknownFields
)
}
public companion object Deserializer : AbstractDeserializer() {
@JvmStatic
override fun deserialize(reader: Reader): ListLocationsResponse {
var locations: MutableList? = null
var nextPageToken = ""
var unknownFields: UnknownFieldSet.Builder? = null
while (true) {
when (reader.readTag()) {
0u -> return ListLocationsResponse(
unmodifiableList(locations),
nextPageToken,
UnknownFieldSet.from(unknownFields)
)
10u ->
locations =
(locations ?: mutableListOf()).apply {
reader.readRepeated(false) {
add(reader.readMessage(Location))
}
}
18u -> nextPageToken = reader.readString()
else ->
unknownFields =
(unknownFields ?: UnknownFieldSet.Builder()).also {
it.add(reader.readUnknown())
}
}
}
}
@JvmStatic
public operator fun invoke(dsl: Builder.() -> Unit): ListLocationsResponse = Builder().apply(dsl).build()
}
}
/**
* The request message for [Locations.GetLocation][google.cloud.location.Locations.GetLocation].
*/
@GeneratedMessage("google.cloud.location.GetLocationRequest")
public class GetLocationRequest private constructor(
/**
* Resource name for the location.
*/
@GeneratedProperty(1)
public val name: String,
public val unknownFields: UnknownFieldSet = UnknownFieldSet.empty()
) : AbstractMessage() {
private val `$messageSize`: Int by lazy {
var result = 0
if (name.isNotEmpty()) {
result += sizeOf(10u) + sizeOf(name)
}
result += unknownFields.size()
result
}
override fun messageSize(): Int = `$messageSize`
override fun serialize(writer: Writer) {
if (name.isNotEmpty()) {
writer.writeTag(10u).write(name)
}
writer.writeUnknown(unknownFields)
}
override fun equals(other: KotlinAny?): Boolean =
other is GetLocationRequest &&
other.name == name &&
other.unknownFields == unknownFields
override fun hashCode(): Int {
var result = unknownFields.hashCode()
result = 31 * result + name.hashCode()
return result
}
override fun toString(): String =
"GetLocationRequest(" +
"name=$name" +
if (unknownFields.isEmpty()) ")" else ", unknownFields=$unknownFields)"
public fun copy(builder: Builder.() -> Unit): GetLocationRequest =
Builder().apply {
name = [email protected]
unknownFields = [email protected]
builder()
}.build()
@BuilderDsl
public class Builder {
public var name: String = ""
public var unknownFields: UnknownFieldSet = UnknownFieldSet.empty()
public fun build(): GetLocationRequest =
GetLocationRequest(
name,
unknownFields
)
}
public companion object Deserializer : AbstractDeserializer() {
@JvmStatic
override fun deserialize(reader: Reader): GetLocationRequest {
var name = ""
var unknownFields: UnknownFieldSet.Builder? = null
while (true) {
when (reader.readTag()) {
0u -> return GetLocationRequest(
name,
UnknownFieldSet.from(unknownFields)
)
10u -> name = reader.readString()
else ->
unknownFields =
(unknownFields ?: UnknownFieldSet.Builder()).also {
it.add(reader.readUnknown())
}
}
}
}
@JvmStatic
public operator fun invoke(dsl: Builder.() -> Unit): GetLocationRequest = Builder().apply(dsl).build()
}
}
/**
* A resource that represents Google Cloud Platform location.
*/
@GeneratedMessage("google.cloud.location.Location")
public class Location private constructor(
/**
* Resource name for the location, which may vary between implementations. For example:
* `"projects/example-project/locations/us-east1"`
*/
@GeneratedProperty(1)
public val name: String,
/**
* Cross-service attributes for the location. For example
*
* {"cloud.googleapis.com/region": "us-east1"}
*/
@GeneratedProperty(2)
public val labels: Map,
/**
* Service-specific metadata. For example the available capacity at the given location.
*/
@GeneratedProperty(3)
public val metadata: ProtobufAny?,
/**
* The canonical id for this location. For example: `"us-east1"`.
*/
@GeneratedProperty(4)
public val locationId: String,
/**
* The friendly name for this location, typically a nearby city name. For example, "Tokyo".
*/
@GeneratedProperty(5)
public val displayName: String,
public val unknownFields: UnknownFieldSet = UnknownFieldSet.empty()
) : AbstractMessage() {
private val `$messageSize`: Int by lazy {
var result = 0
if (name.isNotEmpty()) {
result += sizeOf(10u) + sizeOf(name)
}
if (labels.isNotEmpty()) {
result +=
sizeOf(labels, 18u) { k, v ->
LabelsEntry.entrySize(k, v)
}
}
if (metadata != null) {
result += sizeOf(26u) + sizeOf(metadata)
}
if (locationId.isNotEmpty()) {
result += sizeOf(34u) + sizeOf(locationId)
}
if (displayName.isNotEmpty()) {
result += sizeOf(42u) + sizeOf(displayName)
}
result += unknownFields.size()
result
}
override fun messageSize(): Int = `$messageSize`
override fun serialize(writer: Writer) {
if (name.isNotEmpty()) {
writer.writeTag(10u).write(name)
}
labels.entries.forEach {
writer.writeTag(18u).write(LabelsEntry(it.key, it.value))
}
if (metadata != null) {
writer.writeTag(26u).write(metadata)
}
if (locationId.isNotEmpty()) {
writer.writeTag(34u).write(locationId)
}
if (displayName.isNotEmpty()) {
writer.writeTag(42u).write(displayName)
}
writer.writeUnknown(unknownFields)
}
override fun equals(other: KotlinAny?): Boolean =
other is Location &&
other.name == name &&
other.labels == labels &&
other.metadata == metadata &&
other.locationId == locationId &&
other.displayName == displayName &&
other.unknownFields == unknownFields
override fun hashCode(): Int {
var result = unknownFields.hashCode()
result = 31 * result + name.hashCode()
result = 31 * result + labels.hashCode()
result = 31 * result + metadata.hashCode()
result = 31 * result + locationId.hashCode()
result = 31 * result + displayName.hashCode()
return result
}
override fun toString(): String =
"Location(" +
"name=$name, " +
"labels=$labels, " +
"metadata=$metadata, " +
"locationId=$locationId, " +
"displayName=$displayName" +
if (unknownFields.isEmpty()) ")" else ", unknownFields=$unknownFields)"
public fun copy(builder: Builder.() -> Unit): Location =
Builder().apply {
name = [email protected]
labels = [email protected]
metadata = [email protected]
locationId = [email protected]
displayName = [email protected]
unknownFields = [email protected]
builder()
}.build()
@BuilderDsl
public class Builder {
public var name: String = ""
public var labels: Map = emptyMap()
set(newValue) {
field = copyMap(newValue)
}
public var metadata: ProtobufAny? = null
public var locationId: String = ""
public var displayName: String = ""
public var unknownFields: UnknownFieldSet = UnknownFieldSet.empty()
public fun build(): Location =
Location(
name,
unmodifiableMap(labels),
metadata,
locationId,
displayName,
unknownFields
)
}
public companion object Deserializer : AbstractDeserializer() {
@JvmStatic
override fun deserialize(reader: Reader): Location {
var name = ""
var labels: MutableMap? = null
var metadata: ProtobufAny? = null
var locationId = ""
var displayName = ""
var unknownFields: UnknownFieldSet.Builder? = null
while (true) {
when (reader.readTag()) {
0u -> return Location(
name,
unmodifiableMap(labels),
metadata,
locationId,
displayName,
UnknownFieldSet.from(unknownFields)
)
10u -> name = reader.readString()
18u ->
labels =
(labels ?: mutableMapOf()).apply {
reader.readRepeated(false) {
reader.readMessage(LabelsEntry).let {
put(it.key, it.value)
}
}
}
26u -> metadata = reader.readMessage(ProtobufAny)
34u -> locationId = reader.readString()
42u -> displayName = reader.readString()
else ->
unknownFields =
(unknownFields ?: UnknownFieldSet.Builder()).also {
it.add(reader.readUnknown())
}
}
}
}
@JvmStatic
public operator fun invoke(dsl: Builder.() -> Unit): Location = Builder().apply(dsl).build()
}
private class LabelsEntry(
public val key: String,
public val `value`: String
) : AbstractMessage() {
override fun messageSize(): Int = entrySize(key, value)
override fun serialize(writer: Writer) {
writer.writeTag(10u).write(key)
writer.writeTag(18u).write(`value`)
}
public companion object Deserializer : AbstractDeserializer() {
public fun entrySize(
key: String,
`value`: String
): Int =
sizeOf(10u) + sizeOf(key) +
sizeOf(18u) + sizeOf(`value`)
override fun deserialize(reader: Reader): LabelsEntry {
var key = ""
var value = ""
while (true) {
when (reader.readTag()) {
0u -> return LabelsEntry(key, value)
10u -> key = reader.readString()
18u -> value = reader.readString()
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy