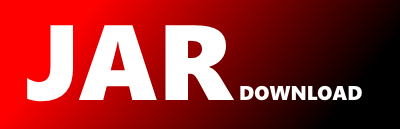
jdbishard.dropwizard.testapp.dao.ClientDAO Maven / Gradle / Ivy
The newest version!
package jdbishard.dropwizard.testapp.dao;
import java.util.Optional;
import java.util.UUID;
import jdbishard.jdbi.DAORegistry;
import jdbishard.sharding.ShardRegistry;
/**
* This class demonstrates hiding of shard-specific DAOs from business logic. It delegates
* to the appropriate ClientShardDAO instance for the operation being performed.
*/
public class ClientDAO {
private final ShardRegistry shardRegistry;
private final DAORegistry daoRegistry;
public ClientDAO(
ShardRegistry shardRegistry,
DAORegistry daoRegistry) {
this.shardRegistry = shardRegistry;
this.daoRegistry = daoRegistry;
}
public void createTablesEverywhere() {
for (String shardId : shardRegistry.registeredShardIDs()) {
Optional dao = daoRegistry.selectByShard(
ClientShardDAO.class,
shardId);
checkNotPresent(dao, "dao", "shardId: " + shardId);
dao.get().createClientAgeTable();
}
}
public void insertClientAge(UUID clientId, int age) {
Optional clientShard = shardRegistry.getShardFor(clientId);
checkNotPresent(clientShard, "shard", "clientId: " + clientId);
Optional dao = daoRegistry.selectByShard(
ClientShardDAO.class,
clientShard.get());
checkNotPresent(dao, "dao", "shardId: " + clientShard.get());
dao.get().insertClientAge(clientId, age);
}
public int findAgeById(UUID clientId) {
Optional clientShard = shardRegistry.getShardFor(clientId);
checkNotPresent(clientShard, "shard", "clientId: " + clientId);
Optional dao = daoRegistry.selectByShard(
ClientShardDAO.class,
clientShard.get());
checkNotPresent(dao, "dao", "shardId: " + clientShard.get());
return dao.get().findAgeById(clientId);
}
public int sumAgeByShard(String shardId) {
Optional dao = daoRegistry.selectByShard(
ClientShardDAO.class, shardId);
checkNotPresent(dao, "dao", "shardId: " + shardId);
return dao.get().sumClientAge();
}
public int sumAge() {
int age = 0;
for (String shardId : shardRegistry.registeredShardIDs()) {
Optional dao = daoRegistry.selectByShard(
ClientShardDAO.class,
shardId);
checkNotPresent(dao, "dao", "shardId: " + shardId);
age += dao.get().sumClientAge();
}
return age;
}
private void checkNotPresent(Optional o, String name, String context) {
if (!o.isPresent()) {
throw new RuntimeException(name + " required but not present. " + context);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy