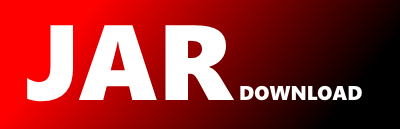
com.tobedevoured.command.Planable Maven / Gradle / Ivy
package com.tobedevoured.command;
import java.util.List;
import java.util.Map;
/**
* Plan used to execute a method on an {@link ByYourCommand} annotated Class
*
* @author Michael Guymon
*
*/
public interface Planable {
/**
* Get the target for this Plan
*
* @return Object
* @throws CommandException
*/
public abstract Object buildTarget() throws CommandException;
/**
* Add a {@link CommandMethod}
*
* @param command {@link CommandMethod}
*/
public abstract void addCommand(CommandMethod command);
/**
* Exec default {@link CommandMethod}
*
* @param params {@link List}
* @return {@link CommandMethod}
* @throws CommandException
*/
public abstract CommandMethod exec(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy