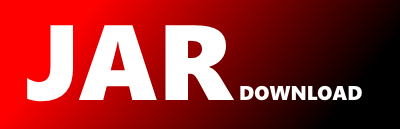
org.eclipse.wst.common.frameworks.datamodel.IDataModel Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2003, 2006 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
package org.eclipse.wst.common.frameworks.datamodel;
import java.util.Collection;
import java.util.List;
import org.eclipse.core.runtime.IStatus;
/**
*
* IDataModels are the core piece of a framework used to simplify data collection, operation
* execution, and Wizard generation.
*
*
* IDataModels are primarily an intelligent mechanism for managing data. Each IDataModel tracks
* specific Objects known as "properties". Each property may be set or get using its property name.
* A Collection of property names for an IDataModel instance may be retreived using
* getAllProperties()
. In addition to getting/setting properties, IDataModels may
* also provide default values for unset properties, human readable descriptors for properties,
* enumerations of valid property values, validation for properties, and enablement for properties.
*
*
* IDataModels may also be nested (and unnested) recursively within another. When one IDataModel is
* nested within another, then client code may access all properties on the former through the
* latter. This is especially useful when the same IDataModel (tracking the same properties) may be
* used within the context of several different broader scenarios. Nesting may apply to any
* IDataModel, and may be abitraryly deep (even cylical if you dare). Nesting offers flexibility,
* especially for extension by 3rd party clients.
*
*
* Each IDataModel may also supply an IDataModelOperation (a subclass of
* org.eclipse.core.commands.operations.IUndoableOperation) for execution. When executed within the
* IDataModel framework all these operations are inherently and abitrarily extendable.
*
*
* Each IDataModel may also indirectly supply a DataModelWizard. This indirection is necessary to
* spilt UI dependencies from the core IDataModel framework. DataModelWizards are also inherently
* extendable.
*
*
* IDataModels are not meant to be instantiated directly, rather they are built from an
* IDataModelProvider. Clients wishing to define their own IDataModel must do so by implementing an
* IDataModelProvider. Clients wishing to utilize an IDataModel must create it using the
* DataModelFactory with the associated IDataModelProvider.
*
*
*
* This interface is not intended to be implemented by clients.
*
*
* @see org.eclipse.wst.common.frameworks.datamodel.IDataModelProvider
* @see org.eclipse.wst.common.frameworks.datamodel.DataModelFactory
*
* @since 1.0
*/
public interface IDataModel {
/**
*
* Returns the unique ID which identifies this IDataModel instance. The same ID should be used
* by the default operation (if any) for clients to extend or instantiate directly, the
* DataModelWizard (if any) for clients to extend or instantiate directly.
*
*
* Note, this is not the same as a hashcode. Multiple IDataModel instances created with the same
* IDataModelProvider type will all have the same ID.
*
*
* An IDataModel implementor defines this in IDataModelProvider.
*
*
* @see IDataModelProvider#getID()
*
* @return the unique ID for this IDataModel
*/
public String getID();
/**
*
* Returns the default operation to execute against this IDataModel. The returned operation is
* derived from the operation supplied by the backing IDataModelProvider. The returned type is
* IDataModelManagerOperation which adds additional functionality to the returned
* IDataModelOperation.
*
*
* An IDataModel implementor defines this in IDataModelProvider.
*
*
* @see IDataModelProvider#getDefaultOperation()
*
* @return the default operation
*/
public IDataModelOperation getDefaultOperation();
/**
*
* This method only pertains to IDataModels for extedended operations. The returned extended
* context is used by the IDataModelOperation framework to determine whether a particular
* extended operation should execute. The returned list is should contain Objects adaptable to
* IProject. This IDataModel's function groups are looked up through the extension mechanism. If
* a function group is defined, it is first checked for enablement. Then each adapted IProject
* is inspected to verify it handles the function group. If all these conditions are met, then
* the extended operation associated with this IDataModel is executed; otherwise it is skipped.
* If no function group is defined, or no extended context is defined (i.e. this method returns
* an empty list, or the objects in the returned list are not adaptable to IProject) then the
* extended operation will execute (it will never be skipped).
*
*
* An IDataModel implementor defines this in IDataModelProvider.
*
*
* This method should not be called by clients.
*
*
* @return a List of Objects adaptable to IProject
*
* @see IDataModelProvider#getExtendedContext()
*/
public List getExtendedContext();
/**
*
* Returns the property value for the specified propertyName.
*
*
* If the specified propertyName is not a property then a RuntimeException will be thrown.
*
*
* Before the property is returned, first the owning IDataModel must be located. If the
* specified propertyName is a base property {@link #isBaseProperty(String)}, then this
* IDataModel is the owner. Otherwise, a recursive search through the nested IDataModels is
* conducted to locate the owning IDataModel. If more than one nested IDataModel defines the
* property, then the first one located is considered the owning IDataModel.
*
*
* Once the owning IDataModel is found the property is checked to see if it is set
* {@link #isPropertySet(String)}. If the property is set, the set value is returned. If the
* property is not set, its default is returned {@link #getDefaultProperty(String)}.
*
*
* There are convenience methods for getting primitive int
and
* boolean
types as well as Strings.
*
* - {@link #getIntProperty(String)}
* - {@link #getBooleanProperty(String)}
* - {@link #getStringProperty(String)}
*
*
*
* @param propertyName
* the property name
* @return the property
*
* @see #setProperty(String, Object)
* @see #getBooleanProperty(String)
* @see #getIntProperty(String)
* @see #getStringProperty(String)
*/
public Object getProperty(String propertyName);
/**
*
* Returns the default property value for the specified propertyName.
*
*
* An IDataModel implementor defines this in IDataModelProvider.
*
*
* @see IDataModelProvider#getDefaultProperty(String)
*/
public Object getDefaultProperty(String propertyName);
/**
*
* A convenience method for getting ints. If the property is set then this method is equavalent
* to:
*
*
* ((Integer)getProperty(propertyName)).intValue();
*
*
* -1
is returned if a call to getProperty(propertyName) returns
* null
.
*
*
* @param propertyName
* the property name
* @return the int value of the property
* @see #setProperty(String, Object)
* @see #setIntProperty(String, int)
*/
public int getIntProperty(String propertyName);
/**
*
* A convenience method for getting booleans. If the property is set then this method is
* equavalent to:
*
*
* ((Boolean)getProperty(propertyName)).booleanValue();
*
*
* false
is returned if a call to getProperty(propertyName) returns
* null
.
*
*
* @param propertyName
* the property name
* @return the boolean value of the property
* @see #setProperty(String, Object)
* @see #setBooleanProperty(String, boolean)
*/
public boolean getBooleanProperty(String propertyName);
/**
*
* A convenience method for getting Strings. If the property is set then this method is
* equavalent to:
*
*
* (String)getProperty(propertyName)
*
*
* ""
is returned if a call to getProperty(propertyName) returns
* null
.
*
*
* @param propertyName
* @see #setProperty(String, Object)
*/
public String getStringProperty(String propertyName);
/**
*
* Sets the specified propertyName to the specified propertyValue. Subsequent calls to
* {@link #getProperty(String)} will return the same propertyValue.
*
*
* When a propertyValue other than null
is set, then the property is considered
* "set" (see {@link #isPropertySet(String)}), conversly, a propertyValue of null
* is considered "unset".
*
*
* If the specified propertyName is not a property (see {@link #isProperty(String)}) then a
* RuntimeException will be thrown.
*
*
* There are convenience methods for setting primitive int
and
* boolean
types as well as Strings.
*
* - {@link #setIntProperty(String, int)}
* - {@link #setBooleanProperty(String, boolean)}
* - {@link #setStringProperty(String, String)}
*
*
*
* An IDataModel implementor may define additional post set logic in
* {@link IDataModelProvider#propertySet(String, Object)}.
*
*
* @param propertyName
* the name of the property to set
* @param propertyValue
* the value to set the property
*
*
* @see #getProperty(String)
* @see #isPropertySet(String)
* @see #isProperty(String)
* @see IDataModelProvider#propertySet(String, Object)
*/
public void setProperty(String propertyName, Object propertyValue);
/**
*
* A convenience method for setting ints. This method is equavalent to:
*
* setProperty(propertyName, new Integer(propertyValue));
*
*
* @param propertyName
* the name of the property
* @param propertyValue
* the int
value of the property
* @see #setProperty(String, Object)
* @see #getIntProperty(String)
*/
public void setIntProperty(String propertyName, int propertyValue);
/**
*
* A convenience method for setting booleans. This method is equavalent to:
*
* setProperty(propertyName, (value) ? Boolean.TRUE : Boolean.FALSE);
*
*
* @param propertyName
* the name of the property
* @param propertyValue
* the boolean
value of the property
* @see #setProperty(String, Object)
* @see #getBooleanProperty(String)
*/
public void setBooleanProperty(String propertyName, boolean propertyValue);
/**
*
* A convenience method for setting Strings. This method is equavalent to:
*
* setProperty(propertyName, propertyValue);
*
*
* @param propertyName
* the name of the property
* @param propertyValue
* the value of the property
* @see #setProperty(String, Object)
* @see #getStringProperty(String)
*/
public void setStringProperty(String propertyName, String propertyValue);
/**
*
* This method is used to nest the specified IDataModel within this IDataModel. The
* nestedModelName
argument should be a unique String to identify this particular
* nested IDataModel. The same String is required when accessing the nested IDataModel using
* either {@link #getNestedModel(String)} or {@link #removeNestedModel(String)}. If the
* specified nested IDataModel has already been nested under this IDataModel or it is the same
* instance as this IDataModel, then calling this method will have no effect.
*
*
* @param nestedModelName
* the name of the IDataModel to be nested
* @param dataModel
* the IDataModel to be nested
* @return true
if the nesting was successful, false
otherwise.
*
* @see #getNestedModel(String)
* @see #removeNestedModel(String)
*/
public boolean addNestedModel(String nestedModelName, IDataModel dataModel);
/**
*
* Remove the specified nestedModel.
*
*
* @param nestedModelName
* the name of the nested IDataModel to remove.
* @return the IDataModel removed, or null
if the nested model does not exist or
* if the specified name is null.
*/
public IDataModel removeNestedModel(String nestedModelName);
/**
*
* Returns true
if a nested model exists (at the top level only) with the
* specified name and false
otherwise.
*
*
* @param nestedModelName
* the name of the nested IDataModel to check.
* @return Returns true
if a nested model exists (at the top level only) with the
* specified name and false
otherwise.
*/
public boolean isNestedModel(String nestedModelName);
/**
*
* Returns the nested IDataModel identified the by the specified name. A RuntimeException is
* thrown if there is no such nested IDataModel (i.e. isNestedModel() would return
* false
).
*
*
* @param nestedModelName
* the name of the nested IDataModel to get.
* @return the nested IDataModel
*/
public IDataModel getNestedModel(String nestedModelName);
/**
*
* Returns a Collection of all nested IDataModels, or an empty Collection if none exist.
*
*
* @return a Collection of all nested IDataModels, or an empty Collection if none exist.
*/
public Collection getNestedModels();
/**
*
* Returns a Collection of all nested IDataModels names, or an empty Collection if none exist.
*
*
* @return a Collection of all nested IDataModels names, or an empty Collection if none exist.
*/
public Collection getNestedModelNames();
/**
*
* Returns a Collection of all nesting (the inverse of nested) IDataModels, or an empty
* Collection if none exist.
*
*
* @return a Collection of all nesting (the inverse of nested) IDataModels, or an empty
* Collection if none exist.
*/
public Collection getNestingModels();
/**
*
* Returns a Collection of all base properties (not including nested properties), or an empty
* Collection if none exist.
*
*
* @return a Collection of all base properties (not including nested properties), or an empty
* Collection if none exist.
*/
public Collection getBaseProperties();
/**
*
* Returns a Collection of all properties of recursively nested IDataModels, or an empty
* Collection if none exist.
*
*
* @return a Collection of all properties of recursively nested IDataModels, or an empty
* Collection if none exist.
*/
public Collection getNestedProperties();
/**
*
* Returns a Collection of all properties (the union of getBaseProperties() and
* getNestedProperties()), or an empty Collection if none exist.
*
*
* @return a Collection of all properties (the union of getBaseProperties() and
* getNestedProperties()), or an empty Collection if none exist.
*/
public Collection getAllProperties();
/**
*
* Returns true
if the specified propertyName is a valid propertyName for this
* root IDataModel only. Nested IDataModels are not checked, though it is possible for a nested
* IDataModel to contain the same property.
*
*
* @param propertyName
* the property name to check
* @return true
if this property is a base property, false
* otherwise.
*
* @see #isProperty(String)
* @see #isNestedProperty(String)
*/
public boolean isBaseProperty(String propertyName);
/**
*
* Returns true
if the specified propertyName is a valid propertyName for this
* DataModel or any of its (recursively) nested IDataModels.
*
*
* @param propertyName
* the property name to check
* @return true
if this is a property, false
otherwise.
*
* @see #isBaseProperty(String)
*/
public boolean isProperty(String propertyName);
/**
*
* Returns true
if the specified propertyName is a valid propertyName for any of
* its (recursively) nested IDataModels. The root IDataModel is not checked, though it is
* possible for the root IDataModel to contain the same property.
*
*
* @param propertyName
* the property name to check
* @return true
if the property is nested, false
otherwise.
* @see #isBaseProperty(String)
*/
public boolean isNestedProperty(String propertyName);
/**
*
* Returns true
if the specified property has been set on the IDataModel. If it
* has not been set, then a call to get the same property will return the default value.
*
*
* @param propertyName
* the property name to check
* @return true
if the property is set, false
otherwise.
*/
public boolean isPropertySet(String propertyName);
/**
*
* Returns true
if the specified property is enabled and false
* otherwise.
*
*
* An IDataModel implementor defines this in IDataModelProvider.
*
*
* @see IDataModelProvider#isPropertyEnabled(String)
*
* @param propertyName
* the property name to check
* @return true
if the specified property is enabled and false
* otherwise.
*/
public boolean isPropertyEnabled(String propertyName);
/**
*
* Returns false
if the the IStatus returned by validateProperty(String) is ERROR
* and true
otherwise.
*
*
* @param propertyName
* the property name to check
* @return false
if the the IStatus returned by validateProperty(String) is ERROR
* and true
otherwise.
*/
public boolean isPropertyValid(String propertyName);
/**
*
* Returns an IStatus for the specified property. Retuns an IStatus.OK if the returned value is
* valid with respect itself, other properites, and broader context of the IDataModel.
* IStatus.ERROR is returned if the returned value is invalid. IStatus.WARNING may also be
* returned if the value is not optimal.
*
*
* An IDataModel implementor defines this in IDataModelProvider.
*
*
* @see IDataModelProvider#validate(String)
*/
public IStatus validateProperty(String propertyName);
/**
*
* Returns false
if the IStatus returned by validate(true) is ERROR and
* true
otherwise.
*
*
* @return false
if the IStatus returned by validate(true) is ERROR and
* true
otherwise.
*/
public boolean isValid();
/**
*
* Equavalent to calling validate(true)
.
*
*
* @return an IStatus
*/
public IStatus validate();
/**
*
* Iterates over all base properties and nested models IDs and calls validate(String). This
* method returns when any call to validate(String) returns an IStatus error and
* stopAtFirstFailure is set to true.
*
*
* @param stopAtFirstFailure
* whether validation should stop at the first failure
* @return an IStatus
*/
public IStatus validate(boolean stopAtFirstFailure);
/**
*
* Returns a DataModelPropertyDescriptor for the specified property. The
* getPropertyValue()
method on the returned DataModelPropertyDescriptor will be
* the same value as returned by getPropertyValue(propertyName)
.
*
*
* Following the example introduced in {@link #getValidPropertyDescriptors(String)}, suppose
* the SHIRT_SIZE
property is currently set to 1. A call to this method would
* return a DataModelPropertyDescriptor whose getPropertyValue()
returns
* 1
and whose getPropertyDescription()
returns small
.
*
*
* Also, note that even if a particular property is not confined to a finite set of values as
* defined by {@link #getValidPropertyDescriptors(String)}this method will always return a
* valid DataModelPropertyDescriptor.
*
*
*
* An IDataModel implementor defines this in IDataModelProvider.
*
*
* @param propertyName
* @return the DataModelPropertyDescriptor for the specified property
*
* @see #getValidPropertyDescriptors(String)
*/
public DataModelPropertyDescriptor getPropertyDescriptor(String propertyName);
/**
*
* Returns a DataModelPropertyDescriptor array consisting of all valid
* DataModelPropertyDescriptors for the specified property. Each
* {@link DataModelPropertyDescriptor} contains a value and a human readible description for the
* value. The set of all values in the returned array are those values which are valid for the
* IDataModel. This value set only makes sense when valid property values conform to a well
* defined finite set. If no such value set exists for the property, then a 0 length array is
* returned. null
is never returned.
*
*
* As an example, suppose there is a property called SHIRT_SIZE
which is an
* Integer
type. Also suppse that valid shirt sizes are only small, medium, or
* large. However, the actual values representing small, medium, and large are 1, 2, and 3
* respectively. A call to getValidPropertyDescriptors(SHIRT_SIZE)
would return a
* DataModelPropertyDescriptor array where the value, description pairs would be {(1, small),
* (2, medium), (3, large)}.
*
*
* An IDataModel implementor defines this in IDataModelProvider.
*
*
* @param propertyName
* then name of the property to check
* @return the array of valid DataModelPropertyDescriptors
* @see #getPropertyDescriptor(String)
*/
public DataModelPropertyDescriptor[] getValidPropertyDescriptors(String propertyName);
/**
*
* Adds the specified IDataModelListener to listen for DataModelEvents. If the specified
* listener has already been added, calling this method will have no effect.
*
*
* @param dataModelListener
* the new listener to add.
*
* @see #removeListener(IDataModelListener)
*/
public void addListener(IDataModelListener dataModelListener);
/**
*
* Remove the specified IDataModelListener. If the specified listener is not a registered
* listenr on this IDataModel, then calling this method will have no effect.
*
*
* @param dataModelListener
* the listener to remove.
* @see #addListener(IDataModelListener)
*/
public void removeListener(IDataModelListener dataModelListener);
/**
*
* A constant used for notification.
*
*
* @see DataModelEvent#VALUE_CHG
* @see #notifyPropertyChange(String, int)
*/
public static final int VALUE_CHG = DataModelEvent.VALUE_CHG;
/**
*
* A constant used for notification. This contant is different from the others because it does
* not map to an event type on DataModelEvent. When notifying with this type, a check is first
* done to see whether the property is set. If the property is NOT set, then a
* VALUE_CHG
is fired, otherwise nothing happens.
*
*
* @see #notifyPropertyChange(String, int)
*/
public static final int DEFAULT_CHG = DataModelEvent.DEFAULT_CHG;
/**
*
* A constant used for notification.
*
*
* @see DataModelEvent#ENABLE_CHG
* @see #notifyPropertyChange(String, int)
*/
public static final int ENABLE_CHG = DataModelEvent.ENABLE_CHG;
/**
*
* A constant used for notification.
*
*
* @see DataModelEvent#VALID_VALUES_CHG
* @see #notifyPropertyChange(String, int)
*/
public static final int VALID_VALUES_CHG = DataModelEvent.VALID_VALUES_CHG;
/**
*
* Notify all listeners of a property change. eventType
specifies the type of
* change. Acceptible values for eventType are VALUE_CHG
,
* DEFAULT_CHG
, ENABLE_CHG
, VALID_VALUES_CHG
. If
* the eventType is DEFAULT_CHG
and the specified property is set, then this
* method will do nothing.
*
*
* Typically this method should only be invoked by an IDataModelProvider from its propertySet
* implementation.
*
*
* @param propertyName
* the name of the property changing
* @param eventType
* the type of event to fire
*
* @see #VALUE_CHG
* @see #DEFAULT_CHG
* @see #ENABLE_CHG
* @see #VALID_VALUES_CHG
* @see DataModelEvent
*/
public void notifyPropertyChange(String propertyName, int eventType);
/**
*
* A typical dispose method used to clean up any resources not handled by general garbage
* collection.
*
*
*/
public void dispose();
}