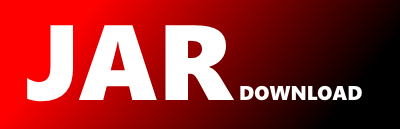
com.tradeshift.sdk.linelinker.TradeshiftLinelinkerClient Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2018 Tradeshift Inc. and/or its affiliates. All rights reserved.
*/
package com.tradeshift.sdk.linelinker;
import static org.asynchttpclient.util.HttpConstants.Methods.POST;
import java.io.IOException;
import java.util.Objects;
import java.util.concurrent.CompletionStage;
import org.asynchttpclient.RequestBuilder;
import org.asynchttpclient.Response;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.module.SimpleModule;
import com.fasterxml.jackson.databind.ser.std.ToStringSerializer;
import com.tradeshift.sdk.core.TradeshiftClient;
import com.tradeshift.sdk.core.TradeshiftClientConfiguration;
import com.tradeshift.sdk.core.domain.ID;
import com.tradeshift.sdk.linelinker.domain.Input;
import com.tradeshift.sdk.linelinker.domain.Result;
import com.tradeshift.sdk.linelinker.json.IDDeserializer;
import com.tradeshift.sdk.linelinker.json.JsonResult;
public final class TradeshiftLinelinkerClient extends TradeshiftClient implements TradeshiftLinelinker {
private static final String BASE_PATH = "rest/external/linelink";
private final ObjectMapper objectMapper;
public TradeshiftLinelinkerClient(TradeshiftClientConfiguration config) {
super(config);
objectMapper = new ObjectMapper();
SimpleModule module = new SimpleModule();
module.addDeserializer(ID.class, new IDDeserializer());
module.addSerializer(ID.class, new ToStringSerializer());
objectMapper.registerModule(module);
}
@Override
public CompletionStage linelink(Input request) {
Objects.requireNonNull(request);
final String url = String.format("%s/%s", endpoint, BASE_PATH);
return execute(authenticated(request, new RequestBuilder(POST)
.setUrl(url)
.setBody(serializeInput(request))
)).thenApply(response -> {
if (response.getStatusCode() == 200) {
return new Result(request.getUserContext(), deserializeResult(response).getResult());
}
throw parseHttpStatusCode(request.getUserContext(), response);
});
}
private byte[] serializeInput(Input request) {
try {
return objectMapper.writeValueAsBytes(request);
} catch (JsonProcessingException e) {
throw new RuntimeException(e);
}
}
private JsonResult deserializeResult(Response response) {
try {
return objectMapper.readValue(response.getResponseBodyAsBytes(), JsonResult.class);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy