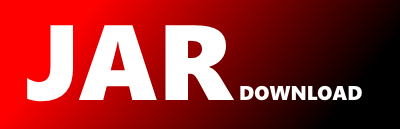
com.treasuredata.client.model.ImmutableTDUser Maven / Gradle / Ivy
Show all versions of td-client Show documentation
package com.treasuredata.client.model;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.google.common.base.MoreObjects;
import com.google.common.base.Optional;
import com.google.common.base.Preconditions;
import com.google.common.collect.Lists;
import com.google.common.primitives.Booleans;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link TDUser}.
*
* Use the builder to create immutable instances:
* {@code ImmutableTDUser.builder()}.
*/
@SuppressWarnings("all")
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "TDUser"})
@Immutable
final class ImmutableTDUser extends TDUser {
private final String id;
private final String name;
private final Optional firstName;
private final Optional lastName;
private final String email;
private final Optional phone;
private final String gravatarUrl;
private final boolean isAdministrator;
private final String createdAt;
private final String updatedAt;
private final boolean isAccountOwner;
private ImmutableTDUser(
String id,
String name,
Optional firstName,
Optional lastName,
String email,
Optional phone,
String gravatarUrl,
boolean isAdministrator,
String createdAt,
String updatedAt,
boolean isAccountOwner) {
this.id = id;
this.name = name;
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
this.phone = phone;
this.gravatarUrl = gravatarUrl;
this.isAdministrator = isAdministrator;
this.createdAt = createdAt;
this.updatedAt = updatedAt;
this.isAccountOwner = isAccountOwner;
}
/**
* @return The value of the {@code id} attribute
*/
@JsonSerialize(using = StringToNumberSerializer.class)
@JsonProperty("id")
@Override
public String getId() {
return id;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("name")
@Override
public String getName() {
return name;
}
/**
* @return The value of the {@code firstName} attribute
*/
@JsonProperty("first_name")
@Override
public Optional getFirstName() {
return firstName;
}
/**
* @return The value of the {@code lastName} attribute
*/
@JsonProperty("last_name")
@Override
public Optional getLastName() {
return lastName;
}
/**
* @return The value of the {@code email} attribute
*/
@JsonProperty("email")
@Override
public String getEmail() {
return email;
}
/**
* @return The value of the {@code phone} attribute
*/
@JsonProperty("phone")
@Override
public Optional getPhone() {
return phone;
}
/**
* @return The value of the {@code gravatarUrl} attribute
*/
@JsonProperty("gravatar_url")
@Override
public String getGravatarUrl() {
return gravatarUrl;
}
/**
* @return The value of the {@code isAdministrator} attribute
*/
@JsonProperty("administrator")
@Override
public boolean isAdministrator() {
return isAdministrator;
}
/**
* @return The value of the {@code createdAt} attribute
*/
@JsonProperty("created_at")
@Override
public String getCreatedAt() {
return createdAt;
}
/**
* @return The value of the {@code updatedAt} attribute
*/
@JsonProperty("updated_at")
@Override
public String getUpdatedAt() {
return updatedAt;
}
/**
* @return The value of the {@code isAccountOwner} attribute
*/
@JsonProperty("account_owner")
@Override
public boolean isAccountOwner() {
return isAccountOwner;
}
/**
* Copy the current immutable object by setting a value for the {@link TDUser#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param id A new value for id
* @return A modified copy of the {@code this} object
*/
public final ImmutableTDUser withId(String id) {
if (this.id.equals(id)) return this;
String newValue = Preconditions.checkNotNull(id, "id");
return new ImmutableTDUser(
newValue,
this.name,
this.firstName,
this.lastName,
this.email,
this.phone,
this.gravatarUrl,
this.isAdministrator,
this.createdAt,
this.updatedAt,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting a value for the {@link TDUser#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param name A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableTDUser withName(String name) {
if (this.name.equals(name)) return this;
String newValue = Preconditions.checkNotNull(name, "name");
return new ImmutableTDUser(
this.id,
newValue,
this.firstName,
this.lastName,
this.email,
this.phone,
this.gravatarUrl,
this.isAdministrator,
this.createdAt,
this.updatedAt,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link TDUser#getFirstName() firstName} attribute.
* @param value The value for firstName
* @return A modified copy of {@code this} object
*/
public final ImmutableTDUser withFirstName(String value) {
Optional newValue = Optional.of(value);
if (this.firstName.equals(newValue)) return this;
return new ImmutableTDUser(
this.id,
this.name,
newValue,
this.lastName,
this.email,
this.phone,
this.gravatarUrl,
this.isAdministrator,
this.createdAt,
this.updatedAt,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting an optional value for the {@link TDUser#getFirstName() firstName} attribute.
* An equality check is used to prevent copying of the same value by returning {@code this}.
* @param optional A value for firstName
* @return A modified copy of {@code this} object
*/
public final ImmutableTDUser withFirstName(Optional optional) {
Optional value = Preconditions.checkNotNull(optional, "firstName");
if (this.firstName.equals(value)) return this;
return new ImmutableTDUser(
this.id,
this.name,
value,
this.lastName,
this.email,
this.phone,
this.gravatarUrl,
this.isAdministrator,
this.createdAt,
this.updatedAt,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link TDUser#getLastName() lastName} attribute.
* @param value The value for lastName
* @return A modified copy of {@code this} object
*/
public final ImmutableTDUser withLastName(String value) {
Optional newValue = Optional.of(value);
if (this.lastName.equals(newValue)) return this;
return new ImmutableTDUser(
this.id,
this.name,
this.firstName,
newValue,
this.email,
this.phone,
this.gravatarUrl,
this.isAdministrator,
this.createdAt,
this.updatedAt,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting an optional value for the {@link TDUser#getLastName() lastName} attribute.
* An equality check is used to prevent copying of the same value by returning {@code this}.
* @param optional A value for lastName
* @return A modified copy of {@code this} object
*/
public final ImmutableTDUser withLastName(Optional optional) {
Optional value = Preconditions.checkNotNull(optional, "lastName");
if (this.lastName.equals(value)) return this;
return new ImmutableTDUser(
this.id,
this.name,
this.firstName,
value,
this.email,
this.phone,
this.gravatarUrl,
this.isAdministrator,
this.createdAt,
this.updatedAt,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting a value for the {@link TDUser#getEmail() email} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param email A new value for email
* @return A modified copy of the {@code this} object
*/
public final ImmutableTDUser withEmail(String email) {
if (this.email.equals(email)) return this;
String newValue = Preconditions.checkNotNull(email, "email");
return new ImmutableTDUser(
this.id,
this.name,
this.firstName,
this.lastName,
newValue,
this.phone,
this.gravatarUrl,
this.isAdministrator,
this.createdAt,
this.updatedAt,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link TDUser#getPhone() phone} attribute.
* @param value The value for phone
* @return A modified copy of {@code this} object
*/
public final ImmutableTDUser withPhone(String value) {
Optional newValue = Optional.of(value);
if (this.phone.equals(newValue)) return this;
return new ImmutableTDUser(
this.id,
this.name,
this.firstName,
this.lastName,
this.email,
newValue,
this.gravatarUrl,
this.isAdministrator,
this.createdAt,
this.updatedAt,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting an optional value for the {@link TDUser#getPhone() phone} attribute.
* An equality check is used to prevent copying of the same value by returning {@code this}.
* @param optional A value for phone
* @return A modified copy of {@code this} object
*/
public final ImmutableTDUser withPhone(Optional optional) {
Optional value = Preconditions.checkNotNull(optional, "phone");
if (this.phone.equals(value)) return this;
return new ImmutableTDUser(
this.id,
this.name,
this.firstName,
this.lastName,
this.email,
value,
this.gravatarUrl,
this.isAdministrator,
this.createdAt,
this.updatedAt,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting a value for the {@link TDUser#getGravatarUrl() gravatarUrl} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param gravatarUrl A new value for gravatarUrl
* @return A modified copy of the {@code this} object
*/
public final ImmutableTDUser withGravatarUrl(String gravatarUrl) {
if (this.gravatarUrl.equals(gravatarUrl)) return this;
String newValue = Preconditions.checkNotNull(gravatarUrl, "gravatarUrl");
return new ImmutableTDUser(
this.id,
this.name,
this.firstName,
this.lastName,
this.email,
this.phone,
newValue,
this.isAdministrator,
this.createdAt,
this.updatedAt,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting a value for the {@link TDUser#isAdministrator() isAdministrator} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param isAdministrator A new value for isAdministrator
* @return A modified copy of the {@code this} object
*/
public final ImmutableTDUser withIsAdministrator(boolean isAdministrator) {
if (this.isAdministrator == isAdministrator) return this;
return new ImmutableTDUser(
this.id,
this.name,
this.firstName,
this.lastName,
this.email,
this.phone,
this.gravatarUrl,
isAdministrator,
this.createdAt,
this.updatedAt,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting a value for the {@link TDUser#getCreatedAt() createdAt} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param createdAt A new value for createdAt
* @return A modified copy of the {@code this} object
*/
public final ImmutableTDUser withCreatedAt(String createdAt) {
if (this.createdAt.equals(createdAt)) return this;
String newValue = Preconditions.checkNotNull(createdAt, "createdAt");
return new ImmutableTDUser(
this.id,
this.name,
this.firstName,
this.lastName,
this.email,
this.phone,
this.gravatarUrl,
this.isAdministrator,
newValue,
this.updatedAt,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting a value for the {@link TDUser#getUpdatedAt() updatedAt} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param updatedAt A new value for updatedAt
* @return A modified copy of the {@code this} object
*/
public final ImmutableTDUser withUpdatedAt(String updatedAt) {
if (this.updatedAt.equals(updatedAt)) return this;
String newValue = Preconditions.checkNotNull(updatedAt, "updatedAt");
return new ImmutableTDUser(
this.id,
this.name,
this.firstName,
this.lastName,
this.email,
this.phone,
this.gravatarUrl,
this.isAdministrator,
this.createdAt,
newValue,
this.isAccountOwner);
}
/**
* Copy the current immutable object by setting a value for the {@link TDUser#isAccountOwner() isAccountOwner} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param isAccountOwner A new value for isAccountOwner
* @return A modified copy of the {@code this} object
*/
public final ImmutableTDUser withIsAccountOwner(boolean isAccountOwner) {
if (this.isAccountOwner == isAccountOwner) return this;
return new ImmutableTDUser(
this.id,
this.name,
this.firstName,
this.lastName,
this.email,
this.phone,
this.gravatarUrl,
this.isAdministrator,
this.createdAt,
this.updatedAt,
isAccountOwner);
}
/**
* This instance is equal to all instances of {@code ImmutableTDUser} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableTDUser
&& equalTo((ImmutableTDUser) another);
}
private boolean equalTo(ImmutableTDUser another) {
return id.equals(another.id)
&& name.equals(another.name)
&& firstName.equals(another.firstName)
&& lastName.equals(another.lastName)
&& email.equals(another.email)
&& phone.equals(another.phone)
&& gravatarUrl.equals(another.gravatarUrl)
&& isAdministrator == another.isAdministrator
&& createdAt.equals(another.createdAt)
&& updatedAt.equals(another.updatedAt)
&& isAccountOwner == another.isAccountOwner;
}
/**
* Computes a hash code from attributes: {@code id}, {@code name}, {@code firstName}, {@code lastName}, {@code email}, {@code phone}, {@code gravatarUrl}, {@code isAdministrator}, {@code createdAt}, {@code updatedAt}, {@code isAccountOwner}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + id.hashCode();
h = h * 17 + name.hashCode();
h = h * 17 + firstName.hashCode();
h = h * 17 + lastName.hashCode();
h = h * 17 + email.hashCode();
h = h * 17 + phone.hashCode();
h = h * 17 + gravatarUrl.hashCode();
h = h * 17 + Booleans.hashCode(isAdministrator);
h = h * 17 + createdAt.hashCode();
h = h * 17 + updatedAt.hashCode();
h = h * 17 + Booleans.hashCode(isAccountOwner);
return h;
}
/**
* Prints the immutable value {@code TDUser} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("TDUser")
.omitNullValues()
.add("id", id)
.add("name", name)
.add("firstName", firstName.orNull())
.add("lastName", lastName.orNull())
.add("email", email)
.add("phone", phone.orNull())
.add("gravatarUrl", gravatarUrl)
.add("isAdministrator", isAdministrator)
.add("createdAt", createdAt)
.add("updatedAt", updatedAt)
.add("isAccountOwner", isAccountOwner)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends TDUser {
@Nullable String id;
@Nullable String name;
Optional firstName = Optional.absent();
Optional lastName = Optional.absent();
@Nullable String email;
Optional phone = Optional.absent();
@Nullable String gravatarUrl;
boolean isAdministrator;
boolean isAdministratorIsSet;
@Nullable String createdAt;
@Nullable String updatedAt;
boolean isAccountOwner;
boolean isAccountOwnerIsSet;
@JsonSerialize(using = StringToNumberSerializer.class)
@JsonProperty("id")
public void setId(String id) {
this.id = id;
}
@JsonProperty("name")
public void setName(String name) {
this.name = name;
}
@JsonProperty("first_name")
public void setFirstName(Optional firstName) {
this.firstName = firstName;
}
@JsonProperty("last_name")
public void setLastName(Optional lastName) {
this.lastName = lastName;
}
@JsonProperty("email")
public void setEmail(String email) {
this.email = email;
}
@JsonProperty("phone")
public void setPhone(Optional phone) {
this.phone = phone;
}
@JsonProperty("gravatar_url")
public void setGravatarUrl(String gravatarUrl) {
this.gravatarUrl = gravatarUrl;
}
@JsonProperty("administrator")
public void setIsAdministrator(boolean isAdministrator) {
this.isAdministrator = isAdministrator;
this.isAdministratorIsSet = true;
}
@JsonProperty("created_at")
public void setCreatedAt(String createdAt) {
this.createdAt = createdAt;
}
@JsonProperty("updated_at")
public void setUpdatedAt(String updatedAt) {
this.updatedAt = updatedAt;
}
@JsonProperty("account_owner")
public void setIsAccountOwner(boolean isAccountOwner) {
this.isAccountOwner = isAccountOwner;
this.isAccountOwnerIsSet = true;
}
@Override
public String getId() { throw new UnsupportedOperationException(); }
@Override
public String getName() { throw new UnsupportedOperationException(); }
@Override
public Optional getFirstName() { throw new UnsupportedOperationException(); }
@Override
public Optional getLastName() { throw new UnsupportedOperationException(); }
@Override
public String getEmail() { throw new UnsupportedOperationException(); }
@Override
public Optional getPhone() { throw new UnsupportedOperationException(); }
@Override
public String getGravatarUrl() { throw new UnsupportedOperationException(); }
@Override
public boolean isAdministrator() { throw new UnsupportedOperationException(); }
@Override
public String getCreatedAt() { throw new UnsupportedOperationException(); }
@Override
public String getUpdatedAt() { throw new UnsupportedOperationException(); }
@Override
public boolean isAccountOwner() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator
static ImmutableTDUser fromJson(Json json) {
ImmutableTDUser.Builder builder = ImmutableTDUser.builder();
if (json.id != null) {
builder.id(json.id);
}
if (json.name != null) {
builder.name(json.name);
}
if (json.firstName != null) {
builder.firstName(json.firstName);
}
if (json.lastName != null) {
builder.lastName(json.lastName);
}
if (json.email != null) {
builder.email(json.email);
}
if (json.phone != null) {
builder.phone(json.phone);
}
if (json.gravatarUrl != null) {
builder.gravatarUrl(json.gravatarUrl);
}
if (json.isAdministratorIsSet) {
builder.isAdministrator(json.isAdministrator);
}
if (json.createdAt != null) {
builder.createdAt(json.createdAt);
}
if (json.updatedAt != null) {
builder.updatedAt(json.updatedAt);
}
if (json.isAccountOwnerIsSet) {
builder.isAccountOwner(json.isAccountOwner);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link TDUser} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable TDUser instance
*/
public static ImmutableTDUser copyOf(TDUser instance) {
if (instance instanceof ImmutableTDUser) {
return (ImmutableTDUser) instance;
}
return ImmutableTDUser.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableTDUser ImmutableTDUser}.
* @return A new ImmutableTDUser builder
*/
public static ImmutableTDUser.Builder builder() {
return new ImmutableTDUser.Builder();
}
/**
* Builds instances of type {@link ImmutableTDUser ImmutableTDUser}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
static final class Builder
implements TDUser.Builder {
private static final long INIT_BIT_ID = 0x1L;
private static final long INIT_BIT_NAME = 0x2L;
private static final long INIT_BIT_EMAIL = 0x4L;
private static final long INIT_BIT_GRAVATAR_URL = 0x8L;
private static final long INIT_BIT_IS_ADMINISTRATOR = 0x10L;
private static final long INIT_BIT_CREATED_AT = 0x20L;
private static final long INIT_BIT_UPDATED_AT = 0x40L;
private static final long INIT_BIT_IS_ACCOUNT_OWNER = 0x80L;
private long initBits = 0xffL;
private @Nullable String id;
private @Nullable String name;
private Optional firstName = Optional.absent();
private Optional lastName = Optional.absent();
private @Nullable String email;
private Optional phone = Optional.absent();
private @Nullable String gravatarUrl;
private boolean isAdministrator;
private @Nullable String createdAt;
private @Nullable String updatedAt;
private boolean isAccountOwner;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code TDUser} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(TDUser instance) {
Preconditions.checkNotNull(instance, "instance");
id(instance.getId());
name(instance.getName());
Optional firstNameOptional = instance.getFirstName();
if (firstNameOptional.isPresent()) {
firstName(firstNameOptional);
}
Optional lastNameOptional = instance.getLastName();
if (lastNameOptional.isPresent()) {
lastName(lastNameOptional);
}
email(instance.getEmail());
Optional phoneOptional = instance.getPhone();
if (phoneOptional.isPresent()) {
phone(phoneOptional);
}
gravatarUrl(instance.getGravatarUrl());
isAdministrator(instance.isAdministrator());
createdAt(instance.getCreatedAt());
updatedAt(instance.getUpdatedAt());
isAccountOwner(instance.isAccountOwner());
return this;
}
/**
* Initializes the value for the {@link TDUser#getId() id} attribute.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
public final Builder id(String id) {
this.id = Preconditions.checkNotNull(id, "id");
initBits &= ~INIT_BIT_ID;
return this;
}
/**
* Initializes the value for the {@link TDUser#getName() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
public final Builder name(String name) {
this.name = Preconditions.checkNotNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the optional value {@link TDUser#getFirstName() firstName} to firstName.
* @param firstName The value for firstName
* @return {@code this} builder for chained invocation
*/
public final Builder firstName(String firstName) {
this.firstName = Optional.of(firstName);
return this;
}
/**
* Initializes the optional value {@link TDUser#getFirstName() firstName} to firstName.
* @param firstName The value for firstName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder firstName(Optional firstName) {
this.firstName = Preconditions.checkNotNull(firstName, "firstName");
return this;
}
/**
* Initializes the optional value {@link TDUser#getLastName() lastName} to lastName.
* @param lastName The value for lastName
* @return {@code this} builder for chained invocation
*/
public final Builder lastName(String lastName) {
this.lastName = Optional.of(lastName);
return this;
}
/**
* Initializes the optional value {@link TDUser#getLastName() lastName} to lastName.
* @param lastName The value for lastName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder lastName(Optional lastName) {
this.lastName = Preconditions.checkNotNull(lastName, "lastName");
return this;
}
/**
* Initializes the value for the {@link TDUser#getEmail() email} attribute.
* @param email The value for email
* @return {@code this} builder for use in a chained invocation
*/
public final Builder email(String email) {
this.email = Preconditions.checkNotNull(email, "email");
initBits &= ~INIT_BIT_EMAIL;
return this;
}
/**
* Initializes the optional value {@link TDUser#getPhone() phone} to phone.
* @param phone The value for phone
* @return {@code this} builder for chained invocation
*/
public final Builder phone(String phone) {
this.phone = Optional.of(phone);
return this;
}
/**
* Initializes the optional value {@link TDUser#getPhone() phone} to phone.
* @param phone The value for phone
* @return {@code this} builder for use in a chained invocation
*/
public final Builder phone(Optional phone) {
this.phone = Preconditions.checkNotNull(phone, "phone");
return this;
}
/**
* Initializes the value for the {@link TDUser#getGravatarUrl() gravatarUrl} attribute.
* @param gravatarUrl The value for gravatarUrl
* @return {@code this} builder for use in a chained invocation
*/
public final Builder gravatarUrl(String gravatarUrl) {
this.gravatarUrl = Preconditions.checkNotNull(gravatarUrl, "gravatarUrl");
initBits &= ~INIT_BIT_GRAVATAR_URL;
return this;
}
/**
* Initializes the value for the {@link TDUser#isAdministrator() isAdministrator} attribute.
* @param isAdministrator The value for isAdministrator
* @return {@code this} builder for use in a chained invocation
*/
public final Builder isAdministrator(boolean isAdministrator) {
this.isAdministrator = isAdministrator;
initBits &= ~INIT_BIT_IS_ADMINISTRATOR;
return this;
}
/**
* Initializes the value for the {@link TDUser#getCreatedAt() createdAt} attribute.
* @param createdAt The value for createdAt
* @return {@code this} builder for use in a chained invocation
*/
public final Builder createdAt(String createdAt) {
this.createdAt = Preconditions.checkNotNull(createdAt, "createdAt");
initBits &= ~INIT_BIT_CREATED_AT;
return this;
}
/**
* Initializes the value for the {@link TDUser#getUpdatedAt() updatedAt} attribute.
* @param updatedAt The value for updatedAt
* @return {@code this} builder for use in a chained invocation
*/
public final Builder updatedAt(String updatedAt) {
this.updatedAt = Preconditions.checkNotNull(updatedAt, "updatedAt");
initBits &= ~INIT_BIT_UPDATED_AT;
return this;
}
/**
* Initializes the value for the {@link TDUser#isAccountOwner() isAccountOwner} attribute.
* @param isAccountOwner The value for isAccountOwner
* @return {@code this} builder for use in a chained invocation
*/
public final Builder isAccountOwner(boolean isAccountOwner) {
this.isAccountOwner = isAccountOwner;
initBits &= ~INIT_BIT_IS_ACCOUNT_OWNER;
return this;
}
/**
* Builds a new {@link ImmutableTDUser ImmutableTDUser}.
* @return An immutable instance of TDUser
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableTDUser build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableTDUser(
id,
name,
firstName,
lastName,
email,
phone,
gravatarUrl,
isAdministrator,
createdAt,
updatedAt,
isAccountOwner);
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if ((initBits & INIT_BIT_ID) != 0) attributes.add("id");
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
if ((initBits & INIT_BIT_EMAIL) != 0) attributes.add("email");
if ((initBits & INIT_BIT_GRAVATAR_URL) != 0) attributes.add("gravatarUrl");
if ((initBits & INIT_BIT_IS_ADMINISTRATOR) != 0) attributes.add("isAdministrator");
if ((initBits & INIT_BIT_CREATED_AT) != 0) attributes.add("createdAt");
if ((initBits & INIT_BIT_UPDATED_AT) != 0) attributes.add("updatedAt");
if ((initBits & INIT_BIT_IS_ACCOUNT_OWNER) != 0) attributes.add("isAccountOwner");
return "Cannot build TDUser, some of required attributes are not set " + attributes;
}
}
}