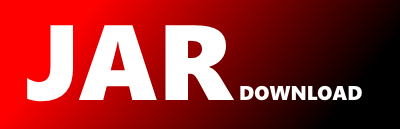
com.treasuredata.client.model.TDExportJobRequestBuilder Maven / Gradle / Ivy
Show all versions of td-client Show documentation
package com.treasuredata.client.model;
import com.google.common.base.Optional;
import com.google.common.base.Preconditions;
import com.google.common.collect.Lists;
import java.util.Date;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.NotThreadSafe;
/**
* {@code TDExportJobRequestBuilder} collects parameters and invokes the static factory method:
* {@code com.treasuredata.client.model.TDExportJobRequest.of(..)}.
* Call the {@link #build()} method to get a result of type {@code com.treasuredata.client.model.TDExportJobRequest}.
* {@code TDExportJobRequestBuilder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@SuppressWarnings("all")
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "TDExportJobRequest.of"})
@NotThreadSafe
public final class TDExportJobRequestBuilder {
private static final long INIT_BIT_DATABASE = 0x1L;
private static final long INIT_BIT_TABLE = 0x2L;
private static final long INIT_BIT_FROM = 0x4L;
private static final long INIT_BIT_TO = 0x8L;
private static final long INIT_BIT_FILE_FORMAT = 0x10L;
private static final long INIT_BIT_ACCESS_KEY_ID = 0x20L;
private static final long INIT_BIT_SECRET_ACCESS_KEY = 0x40L;
private static final long INIT_BIT_BUCKET_NAME = 0x80L;
private static final long INIT_BIT_FILE_PREFIX = 0x100L;
private long initBits = 0x1ffL;
private @Nullable String database;
private @Nullable String table;
private @Nullable Date from;
private @Nullable Date to;
private @Nullable TDExportFileFormatType fileFormat;
private @Nullable String accessKeyId;
private @Nullable String secretAccessKey;
private @Nullable String bucketName;
private @Nullable String filePrefix;
private Optional poolName = Optional.absent();
private Optional domainKey = Optional.absent();
/**
* Creates a {@code TDExportJobRequestBuilder} factory builder.
*/
public TDExportJobRequestBuilder() {
}
/**
* Initializes the value for the {@code database} attribute.
* @param database The value for database
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportJobRequestBuilder database(String database) {
this.database = Preconditions.checkNotNull(database, "database");
initBits &= ~INIT_BIT_DATABASE;
return this;
}
/**
* Initializes the value for the {@code table} attribute.
* @param table The value for table
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportJobRequestBuilder table(String table) {
this.table = Preconditions.checkNotNull(table, "table");
initBits &= ~INIT_BIT_TABLE;
return this;
}
/**
* Initializes the value for the {@code from} attribute.
* @param from The value for from
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportJobRequestBuilder from(Date from) {
this.from = Preconditions.checkNotNull(from, "from");
initBits &= ~INIT_BIT_FROM;
return this;
}
/**
* Initializes the value for the {@code to} attribute.
* @param to The value for to
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportJobRequestBuilder to(Date to) {
this.to = Preconditions.checkNotNull(to, "to");
initBits &= ~INIT_BIT_TO;
return this;
}
/**
* Initializes the value for the {@code fileFormat} attribute.
* @param fileFormat The value for fileFormat
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportJobRequestBuilder fileFormat(TDExportFileFormatType fileFormat) {
this.fileFormat = Preconditions.checkNotNull(fileFormat, "fileFormat");
initBits &= ~INIT_BIT_FILE_FORMAT;
return this;
}
/**
* Initializes the value for the {@code accessKeyId} attribute.
* @param accessKeyId The value for accessKeyId
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportJobRequestBuilder accessKeyId(String accessKeyId) {
this.accessKeyId = Preconditions.checkNotNull(accessKeyId, "accessKeyId");
initBits &= ~INIT_BIT_ACCESS_KEY_ID;
return this;
}
/**
* Initializes the value for the {@code secretAccessKey} attribute.
* @param secretAccessKey The value for secretAccessKey
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportJobRequestBuilder secretAccessKey(String secretAccessKey) {
this.secretAccessKey = Preconditions.checkNotNull(secretAccessKey, "secretAccessKey");
initBits &= ~INIT_BIT_SECRET_ACCESS_KEY;
return this;
}
/**
* Initializes the value for the {@code bucketName} attribute.
* @param bucketName The value for bucketName
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportJobRequestBuilder bucketName(String bucketName) {
this.bucketName = Preconditions.checkNotNull(bucketName, "bucketName");
initBits &= ~INIT_BIT_BUCKET_NAME;
return this;
}
/**
* Initializes the value for the {@code filePrefix} attribute.
* @param filePrefix The value for filePrefix
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportJobRequestBuilder filePrefix(String filePrefix) {
this.filePrefix = Preconditions.checkNotNull(filePrefix, "filePrefix");
initBits &= ~INIT_BIT_FILE_PREFIX;
return this;
}
/**
* Initializes the optional value {@code poolName} to poolName.
* @param poolName The value for poolName
* @return {@code this} builder for chained invocation
*/
public final TDExportJobRequestBuilder poolName(String poolName) {
this.poolName = Optional.of(poolName);
return this;
}
/**
* Initializes the optional value {@code poolName} to poolName.
* @param poolName The value for poolName
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportJobRequestBuilder poolName(Optional poolName) {
this.poolName = Preconditions.checkNotNull(poolName, "poolName");
return this;
}
/**
* Initializes the optional value {@code domainKey} to domainKey.
* @param domainKey The value for domainKey
* @return {@code this} builder for chained invocation
*/
public final TDExportJobRequestBuilder domainKey(String domainKey) {
this.domainKey = Optional.of(domainKey);
return this;
}
/**
* Initializes the optional value {@code domainKey} to domainKey.
* @param domainKey The value for domainKey
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportJobRequestBuilder domainKey(Optional domainKey) {
this.domainKey = Preconditions.checkNotNull(domainKey, "domainKey");
return this;
}
/**
* Invokes {@code com.treasuredata.client.model.TDExportJobRequest.of(..)} using the collected parameters and returns the result of the invocation
* @return A result of type {@code com.treasuredata.client.model.TDExportJobRequest}
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public TDExportJobRequest build() {
checkRequiredAttributes();
return TDExportJobRequest.of(database,
table,
from,
to,
fileFormat,
accessKeyId,
secretAccessKey,
bucketName,
filePrefix,
poolName,
domainKey);
}
private boolean databaseIsSet() {
return (initBits & INIT_BIT_DATABASE) == 0;
}
private boolean tableIsSet() {
return (initBits & INIT_BIT_TABLE) == 0;
}
private boolean fromIsSet() {
return (initBits & INIT_BIT_FROM) == 0;
}
private boolean toIsSet() {
return (initBits & INIT_BIT_TO) == 0;
}
private boolean fileFormatIsSet() {
return (initBits & INIT_BIT_FILE_FORMAT) == 0;
}
private boolean accessKeyIdIsSet() {
return (initBits & INIT_BIT_ACCESS_KEY_ID) == 0;
}
private boolean secretAccessKeyIsSet() {
return (initBits & INIT_BIT_SECRET_ACCESS_KEY) == 0;
}
private boolean bucketNameIsSet() {
return (initBits & INIT_BIT_BUCKET_NAME) == 0;
}
private boolean filePrefixIsSet() {
return (initBits & INIT_BIT_FILE_PREFIX) == 0;
}
private void checkRequiredAttributes() throws IllegalStateException {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if (!databaseIsSet()) attributes.add("database");
if (!tableIsSet()) attributes.add("table");
if (!fromIsSet()) attributes.add("from");
if (!toIsSet()) attributes.add("to");
if (!fileFormatIsSet()) attributes.add("fileFormat");
if (!accessKeyIdIsSet()) attributes.add("accessKeyId");
if (!secretAccessKeyIsSet()) attributes.add("secretAccessKey");
if (!bucketNameIsSet()) attributes.add("bucketName");
if (!filePrefixIsSet()) attributes.add("filePrefix");
return "Cannot build of, some of required attributes are not set " + attributes;
}
}