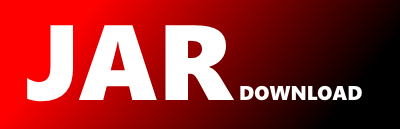
com.treasuredata.client.model.TDExportResultJobRequestBuilder Maven / Gradle / Ivy
Show all versions of td-client Show documentation
package com.treasuredata.client.model;
import com.google.common.base.Optional;
import com.google.common.base.Preconditions;
import com.google.common.collect.Lists;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.NotThreadSafe;
/**
* {@code TDExportResultJobRequestBuilder} collects parameters and invokes the static factory method:
* {@code com.treasuredata.client.model.TDExportResultJobRequest.of(..)}.
* Call the {@link #build()} method to get a result of type {@code com.treasuredata.client.model.TDExportResultJobRequest}.
* {@code TDExportResultJobRequestBuilder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@SuppressWarnings("all")
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "TDExportResultJobRequest.of"})
@NotThreadSafe
public final class TDExportResultJobRequestBuilder {
private static final long INIT_BIT_JOB_ID = 0x1L;
private long initBits = 0x1L;
private @Nullable String jobId;
private Optional resultOutput = Optional.absent();
private Optional resultConnectionId = Optional.absent();
private Optional resultConnectionSettings = Optional.absent();
/**
* Creates a {@code TDExportResultJobRequestBuilder} factory builder.
*/
public TDExportResultJobRequestBuilder() {
}
/**
* Initializes the value for the {@code jobId} attribute.
* @param jobId The value for jobId
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportResultJobRequestBuilder jobId(String jobId) {
this.jobId = Preconditions.checkNotNull(jobId, "jobId");
initBits &= ~INIT_BIT_JOB_ID;
return this;
}
/**
* Initializes the optional value {@code resultOutput} to resultOutput.
* @param resultOutput The value for resultOutput
* @return {@code this} builder for chained invocation
*/
public final TDExportResultJobRequestBuilder resultOutput(String resultOutput) {
this.resultOutput = Optional.of(resultOutput);
return this;
}
/**
* Initializes the optional value {@code resultOutput} to resultOutput.
* @param resultOutput The value for resultOutput
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportResultJobRequestBuilder resultOutput(Optional resultOutput) {
this.resultOutput = Preconditions.checkNotNull(resultOutput, "resultOutput");
return this;
}
/**
* Initializes the optional value {@code resultConnectionId} to resultConnectionId.
* @param resultConnectionId The value for resultConnectionId
* @return {@code this} builder for chained invocation
*/
public final TDExportResultJobRequestBuilder resultConnectionId(String resultConnectionId) {
this.resultConnectionId = Optional.of(resultConnectionId);
return this;
}
/**
* Initializes the optional value {@code resultConnectionId} to resultConnectionId.
* @param resultConnectionId The value for resultConnectionId
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportResultJobRequestBuilder resultConnectionId(Optional resultConnectionId) {
this.resultConnectionId = Preconditions.checkNotNull(resultConnectionId, "resultConnectionId");
return this;
}
/**
* Initializes the optional value {@code resultConnectionSettings} to resultConnectionSettings.
* @param resultConnectionSettings The value for resultConnectionSettings
* @return {@code this} builder for chained invocation
*/
public final TDExportResultJobRequestBuilder resultConnectionSettings(String resultConnectionSettings) {
this.resultConnectionSettings = Optional.of(resultConnectionSettings);
return this;
}
/**
* Initializes the optional value {@code resultConnectionSettings} to resultConnectionSettings.
* @param resultConnectionSettings The value for resultConnectionSettings
* @return {@code this} builder for use in a chained invocation
*/
public final TDExportResultJobRequestBuilder resultConnectionSettings(Optional resultConnectionSettings) {
this.resultConnectionSettings = Preconditions.checkNotNull(resultConnectionSettings, "resultConnectionSettings");
return this;
}
/**
* Invokes {@code com.treasuredata.client.model.TDExportResultJobRequest.of(..)} using the collected parameters and returns the result of the invocation
* @return A result of type {@code com.treasuredata.client.model.TDExportResultJobRequest}
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public TDExportResultJobRequest build() {
checkRequiredAttributes();
return TDExportResultJobRequest.of(jobId, resultOutput, resultConnectionId, resultConnectionSettings);
}
private boolean jobIdIsSet() {
return (initBits & INIT_BIT_JOB_ID) == 0;
}
private void checkRequiredAttributes() throws IllegalStateException {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if (!jobIdIsSet()) attributes.add("jobId");
return "Cannot build of, some of required attributes are not set " + attributes;
}
}