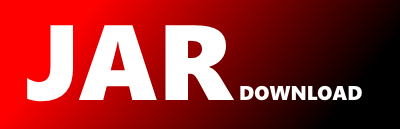
com.treasuredata.client.model.TDJobRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of td-client Show documentation
Show all versions of td-client Show documentation
Treasure Data Client for Java.
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.treasuredata.client.model;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.google.common.base.Optional;
/**
*
*/
public class TDJobRequest
{
private final String database;
private final TDJob.Type type;
private final String query;
private final TDJob.Priority priority;
private final Optional resultOutput;
private final Optional retryLimit;
private final Optional poolName;
private final Optional table;
private final Optional config;
private final Optional scheduledTime;
private final Optional domainKey;
private final Optional resultConnectionId;
private final Optional resultConnectionSettings;
private final Optional engineVersion;
@Deprecated
public TDJobRequest(String database, TDJob.Type type, String query, TDJob.Priority priority, Optional resultOutput, Optional retryLimit, Optional poolName, Optional table, Optional config)
{
this.database = database;
this.type = type;
this.query = query;
this.priority = priority;
this.resultOutput = resultOutput;
this.retryLimit = retryLimit;
this.poolName = poolName;
this.table = table;
this.config = config;
this.scheduledTime = Optional.absent();
this.domainKey = Optional.absent();
this.resultConnectionId = Optional.absent();
this.resultConnectionSettings = Optional.absent();
this.engineVersion = Optional.absent();
}
private TDJobRequest(TDJobRequestBuilder builder)
{
this.database = builder.getDatabase();
this.type = builder.getType();
this.query = builder.getQuery();
this.priority = builder.getPriority();
this.resultOutput = builder.getResultOutput();
this.retryLimit = builder.getRetryLimit();
this.poolName = builder.getPoolName();
this.table = builder.getTable();
this.config = builder.getConfig();
this.scheduledTime = builder.getScheduledTime();
this.domainKey = builder.getDomainKey();
this.resultConnectionId = builder.getResultConnectionId();
this.resultConnectionSettings = builder.getResultConnectionSettings();
this.engineVersion = builder.getEngineVersion();
}
public static TDJobRequest newPrestoQuery(String database, String query)
{
return new TDJobRequestBuilder()
.setType(TDJob.Type.PRESTO)
.setDatabase(database)
.setQuery(query)
.createTDJobRequest();
}
public static TDJobRequest newPrestoQuery(String database, String query, String resultOutput)
{
return new TDJobRequestBuilder()
.setType(TDJob.Type.PRESTO)
.setDatabase(database)
.setQuery(query)
.setResultOutput(resultOutput)
.createTDJobRequest();
}
public static TDJobRequest newPrestoQuery(String database, String query, String resultOutput, String poolName)
{
return new TDJobRequestBuilder()
.setType(TDJob.Type.PRESTO)
.setDatabase(database)
.setQuery(query)
.setResultOutput(resultOutput)
.setPoolName(poolName)
.createTDJobRequest();
}
public static TDJobRequest newHiveQuery(String database, String query)
{
return new TDJobRequestBuilder()
.setType(TDJob.Type.HIVE)
.setDatabase(database)
.setQuery(query)
.createTDJobRequest();
}
public static TDJobRequest newHiveQuery(String database, String query, String resultOutput)
{
return new TDJobRequestBuilder()
.setType(TDJob.Type.HIVE)
.setDatabase(database)
.setQuery(query)
.setResultOutput(resultOutput)
.createTDJobRequest();
}
public static TDJobRequest newHiveQuery(String database, String query, String resultOutput, String poolName)
{
return new TDJobRequestBuilder()
.setType(TDJob.Type.HIVE)
.setDatabase(database)
.setQuery(query)
.setResultOutput(resultOutput)
.setPoolName(poolName)
.createTDJobRequest();
}
public static TDJobRequest newPigQuery(String database, String query)
{
return new TDJobRequestBuilder()
.setType(TDJob.Type.PIG)
.setDatabase(database)
.setQuery(query)
.createTDJobRequest();
}
public static TDJobRequest newPigQuery(String database, String query, String resultOutput)
{
return new TDJobRequestBuilder()
.setType(TDJob.Type.PIG)
.setDatabase(database)
.setQuery(query)
.setResultOutput(resultOutput)
.createTDJobRequest();
}
public static TDJobRequest newPigQuery(String database, String query, String resultOutput, String poolName)
{
return new TDJobRequestBuilder()
.setType(TDJob.Type.PIG)
.setDatabase(database)
.setQuery(query)
.setResultOutput(resultOutput)
.setPoolName(poolName)
.createTDJobRequest();
}
public static TDJobRequest newBulkLoad(String database, String table, ObjectNode config)
{
return new TDJobRequestBuilder()
.setType(TDJob.Type.BULKLOAD)
.setDatabase(database)
.setTable(table)
.setConfig(config)
.setQuery("")
.createTDJobRequest();
}
public String getDatabase()
{
return database;
}
public Optional getTable()
{
return table;
}
public TDJob.Type getType()
{
return type;
}
public String getQuery()
{
return query;
}
public TDJob.Priority getPriority()
{
return priority;
}
public Optional getRetryLimit()
{
return retryLimit;
}
public Optional getResultOutput()
{
return resultOutput;
}
public Optional getPoolName()
{
return poolName;
}
public Optional getConfig()
{
return config;
}
public Optional getScheduledTime()
{
return scheduledTime;
}
public Optional getDomainKey()
{
return domainKey;
}
public Optional getResultConnectionId()
{
return resultConnectionId;
}
public Optional getResultConnectionSettings()
{
return resultConnectionSettings;
}
public Optional getEngineVersion()
{
return engineVersion;
}
static TDJobRequest of(TDJobRequestBuilder builder)
{
return new TDJobRequest(builder);
}
@Override
public String toString()
{
return "TDJobRequest{" +
"database='" + database + '\'' +
", type=" + type +
", query='" + query + '\'' +
", priority=" + priority +
", resultOutput=" + resultOutput +
", retryLimit=" + retryLimit +
", poolName=" + poolName +
", table=" + table +
", config=" + config +
", scheduledTime=" + scheduledTime +
", domainKey=" + domainKey +
", resultConnectionId=" + resultConnectionId +
", resultConnectionSettings=" + resultConnectionSettings +
", engineVersion=" + engineVersion +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy