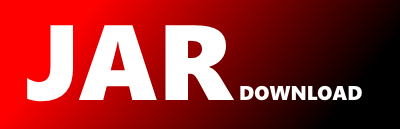
com.trendyol.kediatr.PublishStrategies.kt Maven / Gradle / Ivy
package com.trendyol.kediatr
import kotlinx.coroutines.*
class ContinueOnExceptionPublishStrategy : PublishStrategy {
override suspend fun publish(
notification: T,
notificationHandlers: Collection>,
dispatcher: CoroutineDispatcher
) {
coroutineScope {
withContext(dispatcher) {
val exceptions = mutableListOf()
notificationHandlers.forEach {
try {
it.handle(notification)
} catch (e: Exception) {
exceptions.add(e)
}
}
if (exceptions.isNotEmpty()) {
throw AggregateException(exceptions)
}
}
}
}
}
class StopOnExceptionPublishStrategy : PublishStrategy {
override suspend fun publish(
notification: T,
notificationHandlers: Collection>,
dispatcher: CoroutineDispatcher
) {
coroutineScope {
withContext(dispatcher) {
notificationHandlers.forEach { it.handle(notification) }
}
}
}
}
class ParallelNoWaitPublishStrategy : PublishStrategy {
override suspend fun publish(
notification: T,
notificationHandlers: Collection>,
dispatcher: CoroutineDispatcher
) {
coroutineScope {
withContext(dispatcher) {
notificationHandlers.forEach { launch { it.handle(notification) } }
}
}
}
}
class ParallelWhenAllPublishStrategy : PublishStrategy {
override suspend fun publish(
notification: T,
notificationHandlers: Collection>,
dispatcher: CoroutineDispatcher
) {
coroutineScope {
withContext(dispatcher) {
val deferredResults = notificationHandlers.map { async { it.handle(notification) } }
deferredResults.awaitAll()
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy