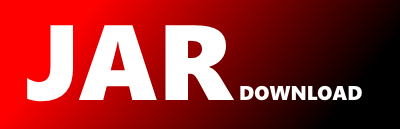
com.trimble.id.BearerTokenHttpClientProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of trimble-id Show documentation
Show all versions of trimble-id Show documentation
Trimble Identity OAuth Client library holds the client classes that are used for communicating with Trimble Identity Service
The newest version!
package com.trimble.id;
import static com.trimble.id.AuthenticationConstants.SDK_VARIANT;
import static com.trimble.id.AuthenticationConstants.SDK_VERSION;
import java.net.URI;
import java.util.concurrent.CompletableFuture;
import com.trimble.id.analytics.AnalyticsHttpClient;
/** A HttpClient provider for APIs using Bearer token authorization */
public class BearerTokenHttpClientProvider implements IHttpClientProvider {
private HttpClient httpClient;
private ITokenProvider tokenProvider;
private URI baseUri;
/**
* Public constructor of BearerTokenHttpClientProvider
*
* @param tokenProvider The token provider that will be used to get the access
* token
* @param baseUri The base URI of the API
* @param configuration The configuration of the HttpClient
*/
public BearerTokenHttpClientProvider(ITokenProvider tokenProvider, URI baseUri,
HttpClientConfiguration configuration) {
this.tokenProvider = tokenProvider;
this.baseUri = baseUri;
this.httpClient = new HttpClient(this.baseUri, configuration);
AnalyticsHttpClient.sendInitEvent(this.getClass().getSimpleName(), this.getClass().getPackage().getName(),
SDK_VERSION, null);
}
/**
* Public constructor of BearerTokenHttpClientProvider
*
* @param tokenProvider The token provider that will be used to get the access
* token
* @param baseUri The base URI of the API
*/
public BearerTokenHttpClientProvider(ITokenProvider tokenProvider, URI baseUri) {
this.tokenProvider = tokenProvider;
this.baseUri = baseUri;
this.httpClient = new HttpClient(this.baseUri);
AnalyticsHttpClient.sendInitEvent(this.getClass().getSimpleName(), this.getClass().getPackage().getName(),
SDK_VERSION, null);
}
/**
* Get the HttpClient with the authorization token set
*
* @return A CompletableFuture that contains the HttpClient with the
* authorization token set
*/
public CompletableFuture retrieveClient() throws SDKClientException {
AnalyticsHttpClient.sendMethodEvent("retrieveClient", SDK_VARIANT, this.getClass().getPackage().getName(),
SDK_VERSION);
return tokenProvider.getAccessToken().thenApply((token) -> {
httpClient.setAuthorizationToken(token);
return httpClient;
});
}
/**
* Set the token provider
*
* @param tokenProvider - The token provider that will be used to get the access
* token
* @return BearerTokenHttpClientProvider
*/
public BearerTokenHttpClientProvider withTokenProvider(FixedTokenProvider tokenProvider) {
this.tokenProvider = tokenProvider;
return this;
}
/**
* Dispose the HttpClient
*/
public void dispose() {
httpClient.dispose();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy