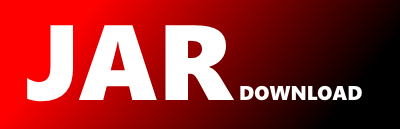
com.trimble.id.CryptographicHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of trimble-id Show documentation
Show all versions of trimble-id Show documentation
Trimble Identity OAuth Client library holds the client classes that are used for communicating with Trimble Identity Service
The newest version!
package com.trimble.id;
import static com.trimble.id.AuthenticationConstants.CODE_CHALLENGE_SIZE;
import static com.trimble.id.AuthenticationConstants.NO_SUCH_ALG_EXCEPTION;
import static com.trimble.id.AuthenticationConstants.STATE_SIZE;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.util.Base64;
public class CryptographicHelper {
private static final Base64.Encoder BASE64 = Base64.getUrlEncoder().withoutPadding();
private static final MessageDigest MESSAGE_DIGEST;
private static final String SHA_256 = "SHA-256";
static {
try {
MESSAGE_DIGEST = MessageDigest.getInstance(SHA_256);
} catch (NoSuchAlgorithmException e) {
throw new SDKClientException(new NoSuchAlgorithmException(NO_SUCH_ALG_EXCEPTION));
}
}
public static byte[] generateRandomBytes(int size) {
byte[] randomBytes = new byte[size];
new SecureRandom().nextBytes(randomBytes);
return randomBytes;
}
public static String toBase64AndUrlEncode(byte[] bytes) {
byte[] base64UrlEncodedBytes = BASE64.encode(bytes);
return new String(base64UrlEncodedBytes);
}
public static byte[] hash(String input) {
return MESSAGE_DIGEST.digest(input.getBytes());
}
public static String generateCodeVerifier() {
byte[] randomBytes = generateRandomBytes(CODE_CHALLENGE_SIZE);
return toBase64AndUrlEncode(randomBytes);
}
public static String createCodeChallenge(String codeVerifier) {
byte[] hashedBytes = hash(codeVerifier);
return toBase64AndUrlEncode(hashedBytes);
}
public static String generateState() {
byte[] randomBytes = generateRandomBytes(STATE_SIZE);
return toBase64AndUrlEncode(randomBytes);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy