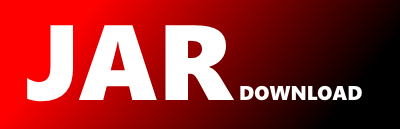
com.trimble.id.FixedEndpointProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of trimble-id Show documentation
Show all versions of trimble-id Show documentation
Trimble Identity OAuth Client library holds the client classes that are used for communicating with Trimble Identity Service
The newest version!
package com.trimble.id;
import static com.trimble.id.AuthenticationConstants.SDK_VERSION;
import java.net.URI;
import java.util.concurrent.CompletableFuture;
import com.trimble.id.analytics.AnalyticsHttpClient;
/** An endpoint provider that returns fixed values */
public class FixedEndpointProvider implements IEndpointProvider {
private URI authorizationEndpoint;
private URI tokenEndpoint;
private URI jwksEndpoint;
private URI revocationEndpoint;
private URI endSessionEndpoint;
/** Public constructor to create FixedEndpointProvider without any endpoints */
public FixedEndpointProvider() {
this.authorizationEndpoint = null;
this.tokenEndpoint = null;
this.jwksEndpoint = null;
this.revocationEndpoint = null;
this.endSessionEndpoint = null;
AnalyticsHttpClient.sendInitEvent(this.getClass().getSimpleName(), this.getClass().getPackage().getName(), SDK_VERSION);
}
/**
* Public constructor of FixedEndpointProvider
*
* @param authorizationEndpoint The authorization endpoint
* @param tokenEndpoint The token endpoint
*/
public FixedEndpointProvider(URI authorizationEndpoint, URI tokenEndpoint) {
this();
this.authorizationEndpoint = authorizationEndpoint;
this.tokenEndpoint = tokenEndpoint;
}
/**
* Fluent extension to add authorization endpoint to the endpoint provider
*
* @param authorizationEndpoint The authorization endpoint
* @return The endpoint provider with the authorization endpoint added
*/
public FixedEndpointProvider WithAuthorizationEndpoint(URI authorizationEndpoint) {
this.authorizationEndpoint = authorizationEndpoint;
return this;
}
/**
* Fluent extension to add token endpoint to the endpoint provider
*
* @param tokenEndpoint The token endpoint
* @return The endpoint provider with the token endpoint added
*/
public FixedEndpointProvider WithTokenEndpoint(URI tokenEndpoint) {
this.tokenEndpoint = tokenEndpoint;
return this;
}
/**
* Fluent extension to add JSON Web Keyset endpoint to the endpoint provider
*
* @param jwksEndpoint The JSON Web Keyset endpoint
* @return The endpoint provider with the JSON Web Keyset endpoint added
*/
public FixedEndpointProvider WithJSONWebKeysetEndpoint(URI jwksEndpoint) {
this.jwksEndpoint = jwksEndpoint;
return this;
}
/**
* Fluent extension to add revocation endpoint to the endpoint provider
*
* @param revocationEndpoint The revocation endpoint
* @return The endpoint provider with the revocation endpoint added
*/
public FixedEndpointProvider WithRevocationEndpoint(URI revocationEndpoint) {
this.revocationEndpoint = revocationEndpoint;
return this;
}
/**
* Fluent extension to add end session endpoint to the endpoint provider
*
* @param endSessionEndpoint The end session endpoint
* @return The endpoint provider with the end session endpoint added
*/
public FixedEndpointProvider WithEndSessionEndpoint(URI endSessionEndpoint) {
this.endSessionEndpoint = endSessionEndpoint;
return this;
}
/**
* Get the authorization endpoint
*
* @return A CompletableFuture that contains the authorization endpoint
*/
public CompletableFuture getAuthorizationEndpoint() {
return CompletableFuture.completedFuture(this.authorizationEndpoint);
}
/**
* Get the token endpoint
*
* @return A CompletableFuture that contains the token endpoint
*/
public CompletableFuture getTokenEndpoint() {
return CompletableFuture.completedFuture(this.tokenEndpoint);
}
/**
* Get the JSON Web Keyset endpoint
*
* @return A CompletableFuture that contains the JSON Web Keyset endpoint
*/
public CompletableFuture getJSONWebKeysetEndpoint() {
return CompletableFuture.completedFuture(this.jwksEndpoint);
}
/**
* Get the revocation endpoint
*
* @return A CompletableFuture that contains the revocation endpoint
*/
public CompletableFuture getRevocationEndpoint() {
return CompletableFuture.completedFuture(this.revocationEndpoint);
}
/**
* Get the end session endpoint
*
* @return A CompletableFuture that contains the end session endpoint
*/
@Override
public CompletableFuture getEndSessionEndpoint() {
return CompletableFuture.completedFuture(this.endSessionEndpoint);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy